在 Java 中,我们一般使用 JdbcTemplate、JPA、MyBatis 等数据持久化方案,当然,最简单的就是 Spring 自带的 JdbcTemplate,下面我们就一起来看看吧
1、引入 JdbcTemplate 依赖
<!-- mysql -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!-- jdbc连接 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<!-- lombok插件 -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
2、数据库配置内容
spring:
# 数据库配置
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://127.0.0.1:3306/test?useUnicode=true&useJDBCCompliantTimezoneShift=true&useLegacyDatetimeCode=false&serverTimezone=UTC&useSSL=true&characterEncoding=UTF-8
# springboot 2.0 整合了hikari ,据说这是目前性能最好的java数据库连接池
hikari:
username: root
password: 123456
3、开始使用
创建 User.java 类
@Data
public class User {
/**
* 主键id
*/
private long id;
/**
* 登录账号
*/
private String name;
/**
* 登录密码
*/
private String password;
/**
* 性别
*/
private int sex;
/**
* 年龄
*/
private int age;
}
这里使用了 lombok 插件,所以使用 @Data 注解可以省略了 get、set、toString 方法
创建 UserDao.java 数据持久层
@Repository
public class User {
@Autowired
private JdbcTemplate jdbcTemplate;
...
}
@Repository,表示这个类具有对对象进行CRUD(增删改查)的功能
@Autowired,自动注入 JdbcTemplate
增
public int addUser(User user) {
String sql = "insert into user(name,password,sex,age) value(?,?,?,?)";
return jdbcTemplate.update(sql, new Object[]{user.getName(), user.getPassword(), user.getSex(), user.getAge()});
}
返回了数据库受影响行数 1
删
扫描二维码关注公众号,回复:
11064869 查看本文章
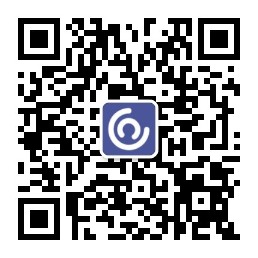
public int deleteUser(int id) {
String sql = "delete from user where id = ?";
return jdbcTemplate.update(sql, new Object[]{id});
}
改
public int updateUser(User user) {
String sql = "update user set name = ?,password = ?,sex = ?,age = ? where id = ?";
return jdbcTemplate.update(sql, new Object[]{user.getName(), user.getPassword(), user.getSex(), user.getAge(), user.getId()});
}
查
public List<User> listUser() {
String sql = "select id,name,password,sex,age from user where 1 = 1";
return jdbcTemplate.query(sql, new BeanPropertyRowMapper<>(User.class));
}
除了这些基本用法之外,JdbcTemplate 也支持其他用法,例如调用存储过程等,这些都比较容易,而且和 Jdbc 本身都比较相似
4、源码分析
在 org.springframework.boot.autoconfigure.jdbc.JdbcTemplateAutoConfiguration 类中,我们可以看到:
@Configuration
@ConditionalOnClass({ DataSource.class, JdbcTemplate.class })
@ConditionalOnSingleCandidate(DataSource.class)
@AutoConfigureAfter(DataSourceAutoConfiguration.class)
@EnableConfigurationProperties(JdbcProperties.class)
public class JdbcTemplateAutoConfiguration {
@Configuration
static class JdbcTemplateConfiguration {
private final DataSource dataSource;
private final JdbcProperties properties;
JdbcTemplateConfiguration(DataSource dataSource, JdbcProperties properties) {
this.dataSource = dataSource;
this.properties = properties;
}
@Bean
@Primary
@ConditionalOnMissingBean(JdbcOperations.class)
public JdbcTemplate jdbcTemplate() {
JdbcTemplate jdbcTemplate = new JdbcTemplate(this.dataSource);
JdbcProperties.Template template = this.properties.getTemplate();
jdbcTemplate.setFetchSize(template.getFetchSize());
jdbcTemplate.setMaxRows(template.getMaxRows());
if (template.getQueryTimeout() != null) {
jdbcTemplate
.setQueryTimeout((int) template.getQueryTimeout().getSeconds());
}
return jdbcTemplate;
}
}
@Configuration
@Import(JdbcTemplateConfiguration.class)
static class NamedParameterJdbcTemplateConfiguration {
@Bean
@Primary
@ConditionalOnSingleCandidate(JdbcTemplate.class)
@ConditionalOnMissingBean(NamedParameterJdbcOperations.class)
public NamedParameterJdbcTemplate namedParameterJdbcTemplate(
JdbcTemplate jdbcTemplate) {
return new NamedParameterJdbcTemplate(jdbcTemplate);
}
}
}
当前类路径下存在 DataSource 和 JdbcTemplate 时,该类就会被自动配置,JdbcTemplate 方法则表示,如果开发者没有自己提供一个 JdbcOperations 的实例的话,系统就自动配置一个 JdbcTemplate Bean(JdbcTemplate 是 JdbcOperations 接口的一个实现)
如您在阅读中发现不足,欢迎留言!!!