dialog在Android开发中会有很多的地方用到,如果不熟悉的话,这一个小的功能可能会耽搁你恒昌时间,下面是我实现自定义dialog的一种方法:
上代码:
1、首先是布局文件:(布局不太好看,后续再更改,已经可以实现自定义的需求了)
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
<TextView
android:id="@+id/tv_prompt"
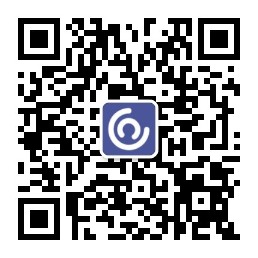
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="16sp"
android:paddingTop="8dp"
android:paddingBottom="5dp"
android:layout_centerHorizontal="true"
android:textColor="@color/colorAccent"
android:text="消息提醒"/>
<LinearLayout
android:id="@+id/llayout_msg"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/tv_prompt"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="30dp"
android:text="提现金额:"/>
<TextView
android:id="@+id/tv_crash"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=""/>
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_below="@id/llayout_msg"
android:background="#dfdfdf" />
<LinearLayout
android:layout_below="@+id/llayout_msg"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:orientation="horizontal">
<Button
android:id="@+id/btn_negative_custom_dialog"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:background="@android:color/transparent"
android:text="取消"
android:textColor="@android:color/holo_blue_dark" />
<View
android:layout_width="1dp"
android:layout_height="match_parent"
android:background="#dfdfdf" />
<Button
android:id="@+id/btn_positive_custom_dialog"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:background="@android:color/transparent"
android:text="确定"
android:textColor="@android:color/holo_blue_dark" />
</LinearLayout>
</RelativeLayout>
2、自定义的dialog类CustomDialog (这才是核心)
public class CustomDialog extends Dialog {
public CustomDialog(Context context) {
super(context);
}
public CustomDialog(Context context, int themeResId) {
super(context, themeResId);
}
protected CustomDialog(Context context, boolean cancelable, OnCancelListener cancelListener) {
super(context, cancelable, cancelListener);
}
public static class Builder{
private Context context;
private String title; //标题
private String message;//提示消息
private String negative_text;//消极的
private String positive_text;//积极的
private OnClickListener negativeListener;//消极的监听
private OnClickListener positiveListener;//积极的监听
public Builder(Context context) {
this.context = context;
}
public Builder setTitle(String title) {
if (title == null) {
this.title = "提醒";
}
this.title = title;
return this;
}
public Builder setMessage(String message) {
if (message == null) {
this.message = "您没有填写提示信息哦";
}
this.message = message;
return this;
}
public Builder setNegativeButton(String negative_text, OnClickListener negativeListener) {
if (negative_text == null) {
this.negative_text = "取消";
}
this.negative_text = negative_text;
this.negativeListener = negativeListener;
return this;
}
public Builder setPositionButton(String positive_text, OnClickListener positiveListener) {
if (positive_text == null) {
this.positive_text = "确定";
}
this.positive_text = positive_text;
this.positiveListener = positiveListener;
return this;
}
private Button btn_negative_custom_dialog;//消极
private Button btn_positive_custom_dialog;//积极
private TextView tv_crash;//提现金额
public CustomDialog create() {
final CustomDialog dialog = new CustomDialog(context);
View view = LayoutInflater.from(context).inflate(R.layout.layout_withdrawal_dialog, null);
dialog.requestWindowFeature(Window.FEATURE_NO_TITLE);
dialog.setContentView(view, new LinearLayout.LayoutParams(ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT));
btn_negative_custom_dialog = (Button) view.findViewById(R.id.btn_negative_custom_dialog);
btn_positive_custom_dialog = (Button) view.findViewById(R.id.btn_positive_custom_dialog);
tv_crash = ((TextView) view.findViewById(R.id.tv_crash));
tv_crash.setText(message);
btn_negative_custom_dialog.setText(negative_text);
btn_positive_custom_dialog.setText(positive_text);
dialog.getWindow().setBackgroundDrawable(new ColorDrawable(Color.WHITE));
btn_negative_custom_dialog.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
negativeListener.onClick(dialog, Dialog.BUTTON_NEGATIVE);
}
});
btn_positive_custom_dialog.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
positiveListener.onClick(dialog, Dialog.BUTTON_POSITIVE);
}
});
return dialog;
}
}
}
3、在需要弹出dialog的地方调用
CustomDialog.Builder builder = new CustomDialog.Builder(this);
builder.setTitle("消息提醒")
.setMessage(String.valueOf("提醒内容区域"))
.setNegativeButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.dismiss();
Toast.makeText(MainActivity.this, "点击了取消", Toast.LENGTH_SHORT).show();
}
})
.setPositionButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.dismiss();
Toast.makeText(MainActivity.this, "点击了确定", Toast.LENGTH_SHORT).show();
}
})
.create()
.show();