695. Max Area of Island
Given a non-empty 2D array grid of 0’s and 1’s, an island is a group of 1’s (representing land) connected 4-directionally (horizontal or vertical.) You may assume all four edges of the grid are surrounded by water.
Find the maximum area of an island in the given 2D array. (If there is no island, the maximum area is 0.)
Example 1:
[[0,0,1,0,0,0,0,1,0,0,0,0,0],
[0,0,0,0,0,0,0,1,1,1,0,0,0],
[0,1,1,0,1,0,0,0,0,0,0,0,0],
[0,1,0,0,1,1,0,0,1,0,1,0,0],
[0,1,0,0,1,1,0,0,1,1,1,0,0],
[0,0,0,0,0,0,0,0,0,0,1,0,0],
[0,0,0,0,0,0,0,1,1,1,0,0,0],
[0,0,0,0,0,0,0,1,1,0,0,0,0]]
Given the above grid, return 6. Note the answer is not 11, because the island must be connected 4-directionally.
思路:
这一题我自己想了好久没想出来,参考了一下大神的答案,现在大致说一下:
其实就是求大矩阵下有几个连着的1,就是求连着的1的最大数目(1和1之间不能用0隔开,否则就不算了)
遍历矩阵中的每一个点,注意这个矩阵是会变化的,原来是1访问一次之后就会变成0,也就是会实时更新。然后把这个为1的点放到stack列表中,然后开始遍历这个点周围的四个点的值,如果周围某一个点为1,就把这个新点的坐标也放到stack种存起来,但是要注意更新这些为1的点的值,也就是存到stack之后就得置为0了,一次次的访问stack中的值,其实也就是上一次存的值为1的那些点的坐标(这里每访问一次stack 就会删掉一个值)再遍历当前点的周围四个点,如果发现有新的1值点,再存到stack中去,知道stack为空,,接下来就可以重新开始检索矩阵中的元素,找到该元素为起始点构成的新面积值,然后更新max的值(存的是当前所构成的最大面积值)
class Solution:
def maxAreaOfIsland(self, grid):
"""
:type grid: List[List[int]]
:rtype: int
"""
Max=0
m,n=len(grid),len(grid[0])
for i in range(m):
for j in range(n):
if grid[i][j]:
area=0 #每次查找一个点 都初始化当前点的area=0
stack=[(i,j)] #存放的是当前点周围为1的点的个数
grid[i][j]=0
while stack:
cur_i,cur_j=stack.pop()
area+=1
if cur_i-1>=0 and grid[cur_i-1][cur_j]:
stack.append((cur_i-1,cur_j))
grid[cur_i-1][cur_j]=0
if cur_i+1<m and grid[cur_i+1][cur_j]:
stack.append((cur_i+1,cur_j))
grid[cur_i+1][cur_j]=0
if cur_j-1>=0 and grid[cur_i][cur_j-1]:
stack.append((cur_i,cur_j-1))
grid[cur_i][cur_j-1]=0
if cur_j+1<n and grid[cur_i][cur_j+1]:
stack.append((cur_i,cur_j+1))
grid[cur_i][cur_j+1]=0
print(area)
Max=max(area,Max)
return Max
283. Move Zeroes
Given an array nums, write a function to move all 0’s to the end of it while maintaining the relative order of the non-zero elements.
For example, given nums = [0, 1, 0, 3, 12], after calling your function, nums should be [1, 3, 12, 0, 0].
Note:
You must do this in-place without making a copy of the array.
Minimize the total number of operations.
Credits:
Special thanks to @jianchao.li.fighter for adding this problem and creating all test cases.
思路:
这一题真的不是用来充数的嘛,,,
class Solution:
def moveZeroes(self, nums):
"""
:type nums: List[int]
:rtype: void Do not return anything, modify nums in-place instead.
"""
n=0
for i in nums:
if i==0:
nums.remove(0)
nums.append(0)
448. Find All Numbers Disappeared in an Array
Given an array of integers where 1 ≤ a[i] ≤ n (n = size of array), some elements appear twice and others appear once.
Find all the elements of [1, n] inclusive that do not appear in this array.
Could you do it without extra space and in O(n) runtime? You may assume the returned list does not count as extra space.
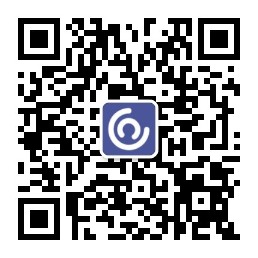
Example:
Input:
[4,3,2,7,8,2,3,1]
Output:
[5,6]
思路:
这一题思路应该很简单,可是写出来以后发现超时了竟然,然后参考了这篇博客提到的difference函数 可以用来求两个集合的差集,这样运行就不会超时啦(博客还讲了求两个集合的并集用的intersection()函数)
class Solution:
def findDisappearedNumbers(self, nums):
"""
:type nums: List[int]
:rtype: List[int]
"""
n=len(nums)
L=[]
for i in range(1,n+1):
L.append(i)
return list(set(L).difference(set(nums)))
下面这个是我第一次写的超时的,,不过简单的数据也是可以的,嘻嘻
def findDisappearedNumbers(nums):
"""
:type nums: List[int]
:rtype: List[int]
"""
L=[]
n=len(nums)
for i in range(1,n+1):
if i not in nums:
L.append(i)
return L
830. Positions of Large Groups
In a string S of lowercase letters, these letters form consecutive groups of the same character.
For example, a string like S = “abbxxxxzyy” has the groups “a”, “bb”, “xxxx”, “z” and “yy”.
Call a group large if it has 3 or more characters. We would like the starting and ending positions of every large group.
The final answer should be in lexicographic order.
Example 1:
Input: “abbxxxxzzy”
Output: [[3,6]]
Explanation: “xxxx” is the single large group with starting 3 and ending positions 6.
思路:
这一题其实挺简单,就是从重复的那个字符串开始计数,从第一个字符遍历到倒数第二个字符,查看下一个字符和当前字符是否一样,一样则将长度累加,否则就重新开始计数,但是在清空之前需要判断重复的字符串长度是不是满足要求,决定是否存到列表中,后来运行当出现的字符串是‘aaa’这种开始到最后都相同,才发现逻辑上有点问题,就是需要在满足第一个条件累加计数时判断是不是到末尾了,这种情况也应该返回,但是不属于第二种情况也就是下一个字符不等于上一个字符的情况,因为到末尾他没有下一个啦~~
我也不知道为什么又想的如此复杂。。
class Solution:
def largeGroupPositions(self, S):
"""
:type S: str
:rtype: List[List[int]]
"""
L=[]
n,length=len(S),1
for i in range(n-1):
if S[i+1]==S[i]:
length+=1
if i==n-2:
if length>=3:
L.append((i-length+2,i+1))
return L
else:
if length>=3:
L.append((i-length+1,i))
length=1
return L
嗯,下面的是我看的讨论区别人的代码,觉得又被秒了。。。
def largeGroupPositions(self, S):
i, j, N = 0, 0, len(S)
res = []
while j < N:
while j < N and S[j] == S[i]: j += 1
if j - i >= 3: res.append((i, j - 1))
i = j
return res