原文链接:https://www.cnblogs.com/liuq/p/9330134.html
点乘和矩阵乘的区别:
1. 点乘(即“ * ”) ---- 各个矩阵对应元素做乘法
若 w 为 m*1 的矩阵,x 为 m*n 的矩阵,那么通过点乘结果就会得到一个 mn 的矩阵。
若 w 为 m*n 的矩阵,x 为 m*n 的矩阵,那么通过点乘结果就会得到一个 mn 的矩阵。
w的列数只能为 1 或与x的列数相等(即n),w的行数与x的行数相等 才能进行乘法运算。
2. 矩阵乘 ---- 按照矩阵乘法规则做运算
若 w 为 m*p 的矩阵,x 为 p*n 的矩阵,那么通过矩阵相乘结果就会得到一个 m*n 的矩阵。
只有w 的列数 == x的行数 时,才能进行乘法运算。
代码示例
1. numpy
点乘
import numpy as np
w = np.array([[0.4], [1.2]])
x = np.array([range(1,6), range(5,10)])
print("w:")
print(w)
print("x:")
print(x)
print("点乘w * x:")
print(w * x)
运行结果
w:
[[0.4]
[1.2]]
x:
[[1 2 3 4 5]
[5 6 7 8 9]]
点乘w * x:
[[ 0.4 0.8 1.2 1.6 2. ]
[ 6. 7.2 8.4 9.6 10.8]]
矩阵乘
import numpy as np
w = np.array([[0.4, 1.2]])
x = np.array([range(1,6), range(5,10)])
print("w:")
print(w)
print("x:")
print(x)
print("矩阵乘w * x:")
print(np.dot(w, x))
运行结果
w:
[[0.4 1.2]]
x:
[[1 2 3 4 5]
[5 6 7 8 9]]
矩阵乘w * x:
[[ 6.4 8. 9.6 11.2 12.8]]
tensorflow
点乘
import tensorflow as tf
w = tf.Variable([[0.4], [1.2]], dtype=tf.float32)
x = tf.Variable([[1.0, 2.0, 3.0, 4.0, 5.0],[6.0, 7.0, 8.0, 9.0, 10.0]], dtype=tf.float32)
y1 = w * x
y2 = tf.multiply(w, x)
w2 = tf.Variable(0.1, dtype=tf.float32)
y3 = w2 * x
y4 = tf.multiply(w2, x)
init = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init)
print("w:")
print(sess.run(w))
print("w.shape:")
print(w.shape)
print("x:")
print(sess.run(x))
print("x.shape:")
print(x.shape)
print("y1:")
print(sess.run(y1))
print("y1.shape:")
print(y1.shape)
print("y2:")
print(sess.run(y2))
print("y2.shape:")
print(y2.shape)
print("--------")
print("w2:")
print(sess.run(w2))
print("w2.shape")
print(w2.shape)
print("y3:")
print(sess.run(y3))
print("y3.shape:")
print(y3.shape)
print("y4:")
print(sess.run(y4))
print("y4.shape:")
print(y4.shape)
运行结果
w:
[[0.4]
[1.2]]
w.shape:
(2, 1)
x:
[[ 1. 2. 3. 4. 5.]
[ 6. 7. 8. 9. 10.]]
x.shape:
(2, 5)
y1:
[[ 0.4 0.8 1.2 1.6 2. ]
[ 7.2000003 8.400001 9.6 10.8 12. ]]
y1.shape:
(2, 5)
y2:
[[ 0.4 0.8 1.2 1.6 2. ]
[ 7.2000003 8.400001 9.6 10.8 12. ]]
y2.shape:
(2, 5)
--------
w2:
0.1
w2.shape
()
y3:
[[0.1 0.2 0.3 0.4 0.5 ]
[0.6 0.7 0.8 0.90000004 1. ]]
y3.shape:
(2, 5)
y4:
[[0.1 0.2 0.3 0.4 0.5 ]
[0.6 0.7 0.8 0.90000004 1. ]]
y4.shape:
(2, 5)
矩阵乘
import tensorflow as tf
w = tf.Variable([[0.4, 1.2]], dtype=tf.float32)
x = tf.Variable([[1.0, 2.0, 3.0, 4.0, 5.0],[6.0, 7.0, 8.0, 9.0, 10.0]], dtype=tf.float32)
y = tf.matmul(w, x)
init = tf.global_variables_initializer()
with tf.Session() as sess:
sess.run(init)
print("w:")
print(sess.run(w))
print("w.shape:")
print(w.shape)
print("x:")
print(sess.run(x))
print("x.shape:")
print(x.shape)
print("y:")
print(sess.run(y))
print("y.shape:")
print(y.shape)
运行结果
扫描二维码关注公众号,回复:
10953222 查看本文章
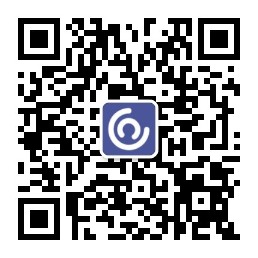
w:
[[0.4 1.2]]
w.shape:
(1, 2)
x:
[[ 1. 2. 3. 4. 5.]
[ 6. 7. 8. 9. 10.]]
x.shape:
(2, 5)
y:
[[ 7.6000004 9.200001 10.8 12.400001 14. ]]
y.shape:
(1, 5)