关于android自定义底部菜单实现与Fragment间的切换,实现过程大体如下:
attrs.xml中的内容:
<resources> <attr name="text" format="string"/> <attr name="icon_normal" format="reference"/> <attr name="icon_pressed" format="reference"/> <declare-styleable name="BottomMenu"> <attr name="text"/> <attr name="icon_normal"/> <attr name="icon_pressed"/> </declare-styleable> </resources>
自定义BottomMenu继承LinearLayout:
public class BottomMenu extends LinearLayout { //xml布局文字属性 private TextView mTv; //xml布局图片属性 private ImageView mIv; //未选中和选中时图片资源id private int mIconNormal, mIconPressed; //区分当前控件是否选中 private boolean mIsSelect; //对应的Fragment private Fragment mFragment; //程序内实例化时使用,只传入context即可。 public BottomMenu(Context context) { super(context); } //用与layout文件实例化,会把xml内的参数同过AttributeSet带入到view内 public BottomMenu(Context context, @Nullable AttributeSet attrs) { super(context, attrs); init(attrs); } //第三个主题的style信息,也会从xml里带入 public BottomMenu(Context context, @Nullable AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); init(attrs); } @SuppressLint("WrongViewCast") private void init(AttributeSet attrs) { //加载对用控件布局文件 LayoutInflater.from(getContext()).inflate(R.layout.layout_custom_bottom_menu, this); //查找控件 mIv = this.findViewById(R.id.iv_bottom); mTv = this.findViewById(R.id.tv_bottom); //通过TypeArray获取对应自定义属性值 TypedArray typedArray = getContext().obtainStyledAttributes(attrs,R.styleable.BottomMenu); String text = typedArray.getString(R.styleable.BottomMenu_text); mIconNormal = typedArray.getResourceId(R.styleable.BottomMenu_icon_normal,0); mIconPressed = typedArray.getResourceId(R.styleable.BottomMenu_icon_pressed,0); mTv.setText(text); mIv.setImageResource(mIconNormal); } /** * 控件被选中状态 */ public void selected(){ if(mIsSelect){ return; } //重新给icon设置被点击时候的图片 mIv.setImageResource(mIconPressed); mIsSelect = true; //重新给文字设置颜色 mTv.setTextColor(getContext().getResources().getColor(R.color.menuSelect)); } /** * 控件未选中状态 */ public void unSelect(){ if(mIsSelect){ } mIsSelect = false; mIv.setImageResource(mIconNormal); mTv.setTextColor(getContext().getResources().getColor(R.color.black)); } public boolean isSelect(){ return mIsSelect; } /** * 设置对应控件对应的Fragment * @param fragment */ public void setFragment(Fragment fragment){ this.mFragment = fragment; } public Fragment getFragment(){ return this.mFragment; } }
Activity xml布局使用自定义控件
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:bottom="http://schemas.android.com/apk/res/cn.puming.struggle.buskiosk" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="cn.puming.struggle.buskiosk.MainActivity"> <FrameLayout android:id="@+id/fragment_container" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_marginBottom="55dp"/> <LinearLayout android:layout_width="match_parent" android:layout_height="50dp" android:layout_alignParentBottom="true" android:layout_marginBottom="15dp" android:orientation="horizontal"> <cn.puming.struggle.buskiosk.customView.BottomMenu android:id="@+id/bottom_home" android:layout_width="match_parent" android:layout_height="wrap_content" bottom:icon_normal="@mipmap/ic_menu_home_normal" bottom:icon_pressed="@mipmap/ic_menu_home_pressed" android:layout_weight="1" bottom:text="首页"/> <cn.puming.struggle.buskiosk.customView.BottomMenu android:id="@+id/bottom_gift" android:layout_width="match_parent" android:layout_height="wrap_content" bottom:icon_normal="@mipmap/ic_menu_welfare_normal" bottom:icon_pressed="@mipmap/ic_menu_welfare_pressed" android:layout_weight="1" bottom:text="礼包"/> <cn.puming.struggle.buskiosk.customView.BottomMenu android:id="@+id/bottom_found" android:layout_width="match_parent" android:layout_height="wrap_content" bottom:icon_normal="@mipmap/ic_menu_community_normal" bottom:icon_pressed="@mipmap/ic_menu_community_pressed" android:layout_weight="1" bottom:text="发现"/> <cn.puming.struggle.buskiosk.customView.BottomMenu android:id="@+id/bottom_msg" android:layout_width="match_parent" android:layout_height="wrap_content" bottom:icon_normal="@mipmap/ic_menu_msg_normal" bottom:icon_pressed="@mipmap/ic_menu_msg_pressed" android:layout_weight="1" bottom:text="消息"/> <cn.puming.struggle.buskiosk.customView.BottomMenu android:id="@+id/bottom_me" android:layout_width="match_parent" android:layout_height="wrap_content" bottom:icon_normal="@mipmap/ic_menu_personal_normal" bottom:icon_pressed="@mipmap/ic_menu_personal_pressed" android:layout_weight="1" bottom:text="我的"/> </LinearLayout> </RelativeLayout>
Activit中代码
public class MainActivity extends AppCompatActivity implements View.OnClickListener{ private ImageView mIv; private BottomMenu mBottomHome; private BottomMenu mBottomGift; private BottomMenu mBottomMe; private BottomMenu mLastBottomMenu; private BottomMenu mBottomFound; private BottomMenu mBottomMsg; private Fragment mHomeFragment,mGiftFragment,mMeFragment,mMsgFragment,mFoundFragment; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mBottomHome = this.findViewById(R.id.bottom_home); mBottomGift = this.findViewById(R.id.bottom_gift); mBottomMe = this.findViewById(R.id.bottom_me); mBottomFound = this.findViewById(R.id.bottom_found); mBottomMsg = this.findViewById(R.id.bottom_msg); mLastBottomMenu = mBottomHome; mBottomHome.selected(); initFragment(); initEvent(); } private void initFragment() { mHomeFragment = new HomeFragment(); mGiftFragment = new GiftFragment(); mMeFragment = new MeFragment(); mMsgFragment = new MsgFragment(); mFoundFragment = new FoundFragment(); FragmentManager manager = getSupportFragmentManager(); FragmentTransaction transaction = manager.beginTransaction(); transaction.add(R.id.fragment_container,mHomeFragment); transaction.add(R.id.fragment_container,mGiftFragment); transaction.add(R.id.fragment_container,mMeFragment); transaction.add(R.id.fragment_container,mMsgFragment); transaction.add(R.id.fragment_container,mFoundFragment); transaction.hide(mGiftFragment); transaction.hide(mMsgFragment); transaction.hide(mMeFragment); transaction.hide(mFoundFragment); transaction.commit(); mBottomHome.setFragment(mHomeFragment); mBottomGift.setFragment(mGiftFragment); mBottomFound.setFragment(mFoundFragment); mBottomMsg.setFragment(mMsgFragment); mBottomMe.setFragment(mMeFragment); } private void initEvent() { mBottomHome.setOnClickListener(this); mBottomGift.setOnClickListener(this); mBottomMe.setOnClickListener(this); mBottomMsg.setOnClickListener(this); mBottomFound.setOnClickListener(this); } @Override public void onClick(View v) { BottomMenu bottomMenu = (BottomMenu) v; if(bottomMenu.isSelect()){ return; } bottomMenu.selected(); FragmentTransaction transaction = getSupportFragmentManager().beginTransaction(); transaction.show(bottomMenu.getFragment()); if(mLastBottomMenu != null){ mLastBottomMenu.unSelect(); transaction.hide(mLastBottomMenu.getFragment()); mLastBottomMenu = bottomMenu; } transaction.commit(); } }
效果图:
扫描二维码关注公众号,回复:
1082320 查看本文章
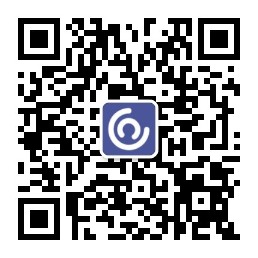