题目一:数组中的字符串匹配
题目链接: https://leetcode-cn.com/problems/string-matching-in-an-array/
解题思路:
按照字符串长度排序,然后从前向后遍历,前面的字符串是否是后面字符串的子串
因为长字符串不可能是短字符串的子串,所以每次第二层遍历的开始位置,从第一层字符串的下一个开始
代码实现:
class Solution:
def stringMatching(self, words: List[str]) -> List[str]:
res = []
words.sort(key = lambda item: len(item))
num = len(words)
for i in range(num):
for j in range(i + 1, num):
if -1 != words[j].find(words[i]):
# print(i, j, words[i], words[j], words[j].find(words[i]))
res.append(words[i])
break
return res
题目二:查询带键的排列
题目链接: https://leetcode-cn.com/problems/queries-on-a-permutation-with-key/
解题思路:
创建一个1 - m为元素的数组,每次将新元素从列表中删除,并插入到数组头部
扫描二维码关注公众号,回复:
10746210 查看本文章
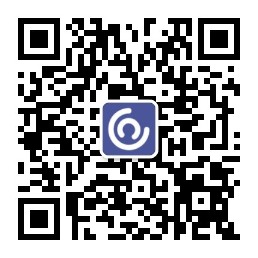
代码实现:
class Solution:
def processQueries(self, queries: List[int], m: int) -> List[int]:
p_lst = [i for i in range(1, m + 1)]
res = []
for a in queries:
idx = p_lst.index(a)
res.append(idx)
p_lst.pop(idx)
p_lst.insert(0, a)
return res
题目三:HTML 实体解析器
题目链接: https://leetcode-cn.com/problems/html-entity-parser/
解题思路:
字符串替换
代码实现:
class Solution:
def entityParser(self, text: str) -> str:
rec = {'"' : '"', ''' : "'", '&' : '&', '>' : '>', '<' : '<', '⁄' : '/'}
for key, val in rec.items():
text = text.replace(key, val)
return text
题目四:给 N x 3 网格图涂色的方案数
题目链接: https://leetcode-cn.com/problems/number-of-ways-to-paint-n-x-3-grid/
解题思路:
方法一:遍历
遍历所有可能性,然后累加
方法二:DP
假设颜色和数字的对应关系为:
红色 : 0
黄色 : 1
绿色 : 2
可以看出,上一行每个使用三种颜色(012格式)时,对应下一行的4种情况,其中2个是三种颜色的情况,2个是两种颜色的情况
上一行使用两种颜色(010格式)时,对应下一行的5种情况,其中2个是三种颜色的情况,3个是两种颜色的情况
所以只需要知道上一行的情况,就可以继续推导出下一行对应的一共有多少种情况
变量:
- last_type_012_num : 上一行012三种颜色的可能情况数
- last_type_010_num : 上一行010三种颜色的可能情况数
- curr_type_012_num : 当前行012可能情况数
- curr_type_010_num : 当前行010可能情况数
- total_num : 当前行总的可能情况数
状态转移公式:
- curr_type_012_num = last_type_012_num * 2 + last_type_010_num * 2
- curr_type_010_num = last_type_012_num * 2 + last_type_010_num * 3
- total_num = curr_type_012_num + curr_type_010_num
代码实现:
方法一:
递归(超时):
class Solution:
def numOfWays(self, n: int) -> int:
max_num = pow(10, 9) + 7
def get_next_line(last_line, n):
nonlocal max_num
if n <= 0:
return 1
num = 0
for i in [0, 1, 2]:
if i == last_line[0]:
continue
for j in [0, 1, 2]:
if j == last_line[1] or j == i:
continue
for k in [0, 1, 2]:
if k == last_line[2] or k == j:
continue
curr_num = 0
curr_line = [i, j, k]
curr_num += get_next_line(curr_line, n - 1)
num += curr_num
num %= max_num
return num
num = 0
for i in [0, 1, 2]:
for j in [0, 1, 2]:
if j == i:
continue
for k in [0, 1, 2]:
curr_num = 0
if k == j:
continue
curr_line = [i, j, k]
curr_num += get_next_line(curr_line, n - 1)
num += curr_num
num %= max_num
return num
迭代:
class Solution:
def numOfWays(self, n: int) -> int:
max_num = pow(10, 9) + 7
lst = {'010','012','020','021','101','102','120','121','201','202','210','212'}
res = {a : 1 for a in lst}
for _ in range(n - 1):
curr_res = {a : 0 for a in lst}
for a in lst:
for b in lst:
if all(last != curr for last, curr in zip(a, b)):
curr_res[a] += res[b]
curr_res[a] %= max_num
res = curr_res
return sum(res.values()) % max_num
方法二:
class Solution:
def numOfWays(self, n: int) -> int:
curr_type_012_num = curr_type_010_num = 6
max_num = pow(10, 9) + 7
for _ in range(n - 1):
curr_type_012_num, curr_type_010_num = curr_type_012_num * 2 + curr_type_010_num * 2, curr_type_012_num * 2 + curr_type_010_num * 3
curr_type_012_num %= max_num
curr_type_010_num %= max_num
return (curr_type_012_num + curr_type_010_num) % max_num