本文翻译自:Entity Framework 5 Updating a Record
I have been exploring different methods of editing/updating a record within Entity Framework 5 in an ASP.NET MVC3 environment, but so far none of them tick all of the boxes I need. 我一直在探索在ASP.NET MVC3环境中编辑/更新实体框架5中的记录的不同方法,但到目前为止,它们都没有勾选我需要的所有框。 I'll explain why. 我会解释原因。
I have found three methods to which I'll mention the pros and cons: 我找到了三种方法,我将提到它的优点和缺点:
Method 1 - Load original record, update each property 方法1 - 加载原始记录,更新每个属性
var original = db.Users.Find(updatedUser.UserId);
if (original != null)
{
original.BusinessEntityId = updatedUser.BusinessEntityId;
original.Email = updatedUser.Email;
original.EmployeeId = updatedUser.EmployeeId;
original.Forename = updatedUser.Forename;
original.Surname = updatedUser.Surname;
original.Telephone = updatedUser.Telephone;
original.Title = updatedUser.Title;
original.Fax = updatedUser.Fax;
original.ASPNetUserId = updatedUser.ASPNetUserId;
db.SaveChanges();
}
Pros 优点
- Can specify which properties change 可以指定更改哪些属性
- Views don't need to contain every property 视图不需要包含每个属性
Cons 缺点
- 2 x queries on database to load original then update it 在数据库上进行2次查询以加载原始数据然后更新它
Method 2 - Load original record, set changed values 方法2 - 加载原始记录,设置更改的值
var original = db.Users.Find(updatedUser.UserId);
if (original != null)
{
db.Entry(original).CurrentValues.SetValues(updatedUser);
db.SaveChanges();
}
Pros 优点
- Only modified properties are sent to database 仅将已修改的属性发送到数据库
Cons 缺点
- Views need to contain every property 视图需要包含每个属性
- 2 x queries on database to load original then update it 在数据库上进行2次查询以加载原始数据然后更新它
Method 3 - Attach updated record and set state to EntityState.Modified 方法3 - 附加更新的记录并将状态设置为EntityState.Modified
db.Users.Attach(updatedUser);
db.Entry(updatedUser).State = EntityState.Modified;
db.SaveChanges();
Pros 优点
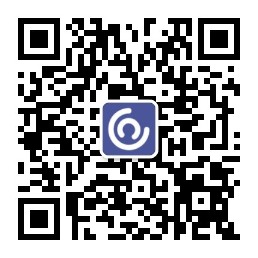
- 1 x query on database to update 1 x查询数据库以进行更新
Cons 缺点
- Can't specify which properties change 无法指定更改哪些属性
- Views must contain every property 视图必须包含每个属性
Question 题
My question to you guys; 我向你们提问; is there a clean way that I can achieve this set of goals? 有没有一种干净的方式可以达到这套目标?
- Can specify which properties change 可以指定更改哪些属性
- Views don't need to contain every property (such as password!) 视图不需要包含每个属性(例如密码!)
- 1 x query on database to update 1 x查询数据库以进行更新
I understand this is quite a minor thing to point out but I may be missing a simple solution to this. 我明白这是一个很小的事情要指出,但我可能错过了一个简单的解决方案。 If not method one will prevail ;-) 如果不是方法一将占上风;-)
#1楼
参考:https://stackoom.com/question/12Leq/实体框架-更新记录
#2楼
You are looking for: 您正在寻找:
db.Users.Attach(updatedUser);
var entry = db.Entry(updatedUser);
entry.Property(e => e.Email).IsModified = true;
// other changed properties
db.SaveChanges();
#3楼
I really like the accepted answer. 我真的很喜欢接受的答案。 I believe there is yet another way to approach this as well. 我相信还有另一种方法可以解决这个问题。 Let's say you have a very short list of properties that you wouldn't want to ever include in a View, so when updating the entity, those would be omitted. 假设您有一个非常短的属性列表,您不希望在View中包含这些属性,因此在更新实体时,这些属性将被省略。 Let's say that those two fields are Password and SSN. 假设这两个字段是密码和SSN。
db.Users.Attach(updatedUser);
var entry = db.Entry(updatedUser);
entry.State = EntityState.Modified;
entry.Property(e => e.Password).IsModified = false;
entry.Property(e => e.SSN).IsModified = false;
db.SaveChanges();
This example allows you to essentially leave your business logic alone after adding a new field to your Users table and to your View. 此示例允许您在向Users表和View添加新字段后,基本上保留业务逻辑。
#4楼
foreach(PropertyInfo propertyInfo in original.GetType().GetProperties()) {
if (propertyInfo.GetValue(updatedUser, null) == null)
propertyInfo.SetValue(updatedUser, propertyInfo.GetValue(original, null), null);
}
db.Entry(original).CurrentValues.SetValues(updatedUser);
db.SaveChanges();
#5楼
I have added an extra update method onto my repository base class that's similar to the update method generated by Scaffolding. 我在我的存储库基类中添加了一个额外的更新方法,类似于Scaffolding生成的更新方法。 Instead of setting the entire object to "modified", it sets a set of individual properties. 它不是将整个对象设置为“已修改”,而是设置一组单独的属性。 (T is a class generic parameter.) (T是类通用参数。)
public void Update(T obj, params Expression<Func<T, object>>[] propertiesToUpdate)
{
Context.Set<T>().Attach(obj);
foreach (var p in propertiesToUpdate)
{
Context.Entry(obj).Property(p).IsModified = true;
}
}
And then to call, for example: 然后打电话,例如:
public void UpdatePasswordAndEmail(long userId, string password, string email)
{
var user = new User {UserId = userId, Password = password, Email = email};
Update(user, u => u.Password, u => u.Email);
Save();
}
I like one trip to the database. 我喜欢一次数据库之旅。 Its probably better to do this with view models, though, in order to avoid repeating sets of properties. 但是,使用视图模型可能更好,以避免重复属性集。 I haven't done that yet because I don't know how to avoid bringing the validation messages on my view model validators into my domain project. 我还没有这样做,因为我不知道如何避免将我的视图模型验证器上的验证消息带到我的域项目中。
#6楼
Just to add to the list of options. 只是添加到选项列表中。 You can also grab the object from the database, and use an auto mapping tool like Auto Mapper to update the parts of the record you want to change.. 您还可以从数据库中获取对象,并使用Auto Mapper等自动映射工具更新要更改的记录部分。