函数式编程
Functional Programming
函数式编程是一种抽象程度很高的编程范式
纯粹的函数式编程语言编写的函数没有变量
函数式编程支持在函数中传入一个函数作为参数,并返回一个函数作为返回值
Python对函数式编程提供部分支持,Python不是纯函数式编程语言
什么是编程范式?
高阶函数
Higher-order function
在Python中变量可以指向函数
>>> abs(-10)
10
>>> f = abs
>>> f(-10)
10
Python中,函数名其实是变量
>>> abs = 10
>>> abs(-10)
Traceback (most recent call last):
File "<pyshell#4>", line 1, in <module>
abs(-10)
TypeError: 'int' object is not callable
>>> abs
10
函数可以接受一个函数作为参数,这类接收函数作为参数的函数称为高阶函数
>>> def add(x, y, f):
return f(x) + f(y)
>>> add(-5, 6, abs)
11
map
map()
函数
扫描二维码关注公众号,回复:
10596523 查看本文章
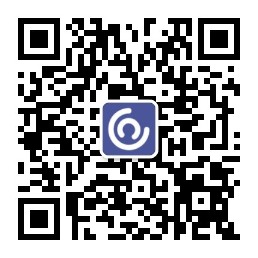
map()接收两个参数,一个是函数,一个是Iterable
map()的作用是将函数作用于Iterable
中的每一个元素,并将结果作为Iterator
返回
>>> r = map(str, [1, 2, 3, 4, 5, 6])
>>> list(r) # r为Iterator,是个惰性序列,使用list()可将所有结果都计算出来并返回
['1', '2', '3', '4', '5', '6']
reduce
reduce
将一个函数作用在一个序列中,这个函数必须接收两个参数。reduce
工作原理如下:
reduce(f, [x1, x2, x3, x4]) = f(((x1, x2), x3), x4)
reduce
函数需要从functools
模块中导入
>>> from functools import reduce
>>> def add(x, y):
return x + y
>>> reduce(add, [1, 3, 5, 7, 9])
25
将序列[1, 2, 3]
转化成整数123
>>> from functools import reduce
>>> def fn(x, y):
return x * 10 + y
>>> reduce(fn, [1, 2, 3])
123
reduce
和map
的应用:将字符串转化成整数
from functools import reduce
DIGITS = {'0': 0, '1': 1, '2': 2, '3': 3, '4': 4, '5': 5, '6': 6, '7': 7, '8': 8, '9': 9}
def str2int(s):
def fn(x, y):
return x * 10 + y
def char2num(s):
return DIGITS[s]
return reduce(fn, map(char2num, s))
# ------------------------------------------------------------------
"""使用lambda表达式简化"""
from functools import reduce
DIGITS = {'0': 0, '1': 1, '2': 2, '3': 3, '4': 4, '5': 5, '6': 6, '7': 7, '8': 8, '9': 9}
def char2num(s):
return DIGITS[s]
def str2int(s):
return reduce(lambda x, y: x * 10 + y, map(char2num, s))
filter
filter()
函数将一个函数作用在一个序列的每个元素上,并根据返回值是True
还是False
来决定元素的去留,False
则丢弃。
我称它为过滤器
def is_odd(n):
return n % 2 == 1
>>> list(filter(is_odd, [1, 2, 4, 5, 6, 9 ,10 ,15]))
[1, 5, 9, 15]
sorted
sorted()
函数
# sorted()函数可对list进行排序
>>> sorted([36, 5, -12, 9, -21])
[-21, -12, 5, 9, 36]
# 可接收一个key函数来自定义排序
>>> sorted([36, 5, -12, 9, -21], key=abs)
[5, 9, -12, -21, 36]
# 反向排序
>>> sorted([36, 5, -12, 9, -21], key=abs, reverse=True)
[36, -21, -12, 9, 5]
# 字符串排序(按ASCII码的大小进行排序)
>>> sorted(['bob', 'about', 'Zoo', 'Credit'])
['Credit', 'Zoo', 'about', 'bob']
匿名函数
能解决什么问题?
lambda
表示匿名函数
,冒号前面的x表示函数参数
lambda表达式不能设置返回值,它的返回值就是表达式的结果
# lambda x: x * x
>>> list(map(lambda x: x * x, [1, 2, 3, 4, 5, 6, 7, 8, 9]))
[1, 4, 9, 16, 25, 36, 49, 64, 81]
lambda x: x * x
# 相当于
def f(x):
return x * x
匿名函数也是函数对象,可以赋值给一个变量
>>> f = lambda x: x * x
>>> f
<function <lambda> at 0x000002BA40A8C4C0>
>>> f(5)
25
可以将lambda表达式作为结果返回
def build(x, y):
return lambda: x * x + y * y