实现功能
- 信息卡呈现在各个模块,并支持用户的搜索与跳转
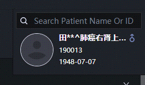
HTML
<div class="patient-card FlexRow">
<div class="input-box FlexCol">
<img class="search-icon" src="../../assets/svg/sousuo.svg">
<input nz-input placeholder="Search Patient Name Or ID" [(ngModel)]="keyWords" (keyup)="search($event);"
(keydown)="enterSubmit($event)" type="text" class="search-input" />
<img class="clear-icon" src="../../assets/svg/clear.svg" (click)="clearKeyWord()" *ngIf="keyWords">
</div>
<div class="search-info" *ngIf="!isLoad && !isSelectedNewPatient && isDropDownList">
<div class="search-card FlexRow" *ngFor="let item of resultUserList" (click)="selectedPatient(item);">
<div class="name-info FlexCol Fill">
<div class="left-info Fill FlexCol">
<div class="name">{{item.patientName}}</div>
<div class="age">{{item.patientAge}}</div>
</div>
<div class="sex">
<span><img src="../../assets/svg/icon_nan.svg" *ngIf="(item.patientSex=='Male')" /></span>
<span><img src="../../assets/svg/icon_nv.svg" *ngIf="(item.patientSex=='Female')" /></span>
</div>
</div>
<div class="patient-id">{{item.patientID}}</div>
</div>
</div>
<div class="search-loading" *ngIf="isLoad">
In the query, please wait a moment.
</div>
<div class="patient-info FlexCol Fill" *ngIf="isHassaerchedPatients && !startSearch">
<img class="left-avatar" src="../../assets/svg/user.svg" />
<div class="right-info Fill">
<p class="patient-name FlexCol">
<span class="name Fill">{{patientName}}</span>
<span class="gender-icon" *ngIf="patientSex"><img src="../../assets/svg/icon_nan.svg"/></span>
<span class="gender-icon" *ngIf="!patientSex"><img src="../../assets/svg/icon_nv.svg"/></span>
</p>
<p class="patient-ID">{{patientID}}</p>
<p class="patient-time">{{selectPatientBirthDate}}(yyyy/MM/dd)</p>
<p class="patient-time">(yyyy/MM/dd)</p>
</div>
</div>
<div *ngIf="!isHassaerchedPatients" class="error-info Fill">
No relevant information was found, please check after inquiry.
</div>
</div>
import { Component, OnInit, Input, Injector } from "@angular/core";
import { PatientDto, PatientServiceProxy, Sorter, ListResultDtoOfPatientDto } from "@shared/service-proxies/service-proxies";
import { ActivatedRoute } from "@angular/router";
import { CurrentPatientService} from "@shared/service-proxies/current-patient.service";
import { PatientHttpService } from "../distribution-service/services/patient-http.service";
import { Subscription } from "rxjs";
import { OpenPatientAction } from "../uView/app-service/patient-action/open-patient-action";
import { ActionExecuter } from "../uView/app-service/action-executer";
@Component({
selector: 'patientCard-component',
templateUrl: './patientCard.component.html',
styleUrls: [
'./patientCard.component.less'
],
})
export class PatientCardComponent implements OnInit {
patients: PatientDto[];
selectPatient: PatientDto;
selectPatientBirthDate: string;
patientName: string;
patientID: string;
patientSex: boolean;
keyWords: string;
resultUserList: PatientDto[];
isHassaerchedPatients: boolean = true;
startSearch: boolean = false;
isLoad: boolean = false;
isSelectedNewPatient: boolean = false;
patientPhotoUrl: string;
searchInput: string;
subscription: Subscription;
isDropDownList:boolean;
@Input()
inputpPatientID: string;
constructor(private _patientService: PatientServiceProxy,
private _activatedRouter: ActivatedRoute,
private _currentPatientService: CurrentPatientService,
private _patientHttp: PatientHttpService,
private injector: Injector) {
}
ngOnInit(): void {
let patientId = this._activatedRouter.queryParams['_value']['vPId'];
this.isDropDownList = false;
this._patientService.getAll(null, 10, 0, "", Sorter._2)
.pipe()
.subscribe((result: ListResultDtoOfPatientDto) => {
this.patients = result.items;
console.log("ID:" + patientId);
this.patients.forEach(pa => {
if (patientId == pa.id) {
this.selectPatient = pa;
this.patientName = pa.patientName;
this.patientID = pa.patientID;
if (pa.patientSex == "Male") {
this.patientSex = true;
}
else {
this.patientSex = false;
}
if (pa.patientsBirthDate != null) {
this.selectPatientBirthDate = pa.patientsBirthDate.format('YYYY/MM/DD');
}
this._currentPatientService.patientChanged(this.selectPatient);
console.log("pa:" + pa.id);
}
});
});
}
enterSubmit(obj) {
var searchControl = document.getElementById('searchControl');
if (obj.keyCode == 13) {
}
else {
}
}
search($event) {
this.startSearch = true;
this.isLoad = true;
this.isSelectedNewPatient = false;
this.isDropDownList = true;
this.searchInput = this.keyWords;
if( this.searchInput != null && this.searchInput != ""){
this.searchInput = this.searchInput.trimLeft().trimRight();
}
if(this.keyWords === "" || this.keyWords == null){
return;
}
if (this.searchInput === "" ||this.keyWords.trim() === "") {
this.resultUserList = new Array<PatientDto>();
this.startSearch = false;
this.isLoad = false;
this.isHassaerchedPatients = true;
this.isDropDownList = false;
console.log("keyword is null");
return;
}
if (this.patients === null || this.patients === undefined || this.patients.length === 0) {
this.resultUserList = new Array<PatientDto>();
return;
}
var len: number = this.patients.length;
this.resultUserList = new Array<PatientDto>();
for (let i = 0; i < len; i++) {
let element: PatientDto = this.patients[i];
if (element.patientID.toLowerCase().indexOf(this.searchInput) !== -1
|| element.patientID.toUpperCase().indexOf(this.searchInput) !== -1
|| element.patientName.toLowerCase().indexOf(this.searchInput) !== -1
|| element.patientName.toUpperCase().indexOf(this.searchInput) !== -1
) {
this.resultUserList.push(element);
}
if (this.resultUserList.length == 0) {
this.isHassaerchedPatients = false;
this.isDropDownList = false;
}
else {
this.isHassaerchedPatients = true;
this.resultUserList.sort(p=>p.id);
this.isDropDownList = true;
}
}
this.isLoad = false;
}
clearKeyWord(){
this.keyWords="";
this.isHassaerchedPatients = true;
this.startSearch = false;
this.isDropDownList = false;
console.log("clear keyword")
}
selectedPatient(patient: PatientDto) {
console.log("selected patient");
this.selectPatient = patient;
this.patientName = patient.patientName;
console.log(this.patientName);
this.patientID = patient.patientID;
console.log(this.patientID);
if (patient.patientSex == "Male") {
this.patientSex = true;
console.log("Male");
}
else {
this.patientSex = false;
console.log("Female");
}
if (patient.patientsBirthDate != null) {
this.selectPatientBirthDate = patient.patientsBirthDate.format('YYYY/MM/DD');
}
this._currentPatientService.patientChanged(this.selectPatient);
this.keyWords = this.patientID;
this.isSelectedNewPatient = true;
this.isHassaerchedPatients = true;
this.startSearch = false;
this.isDropDownList = false;
let openPatientAction = new OpenPatientAction(this.injector);
openPatientAction.SetPatientId(patient.id);
new ActionExecuter().ExecuteAsync(openPatientAction);
}
}
备注:由于贴的工程代码,如有不理解的,请留言