import os
import json
import sys
import hashlib
import time as t
sys.path.append('..\core') # 添加到sys.path中
print(sys.path)
import withdraw # 导入withdraw 虽然报错但是没关系这只是pycharm的问题
"""
尝试把上一章的验证用户登陆的装饰器添加到提现和转账的功能上。
"""
def login_youhua_hash(func):
"""
登录装饰器,文件名:account_hash.json
:return:
"""
def login_decorator(*arg):
count = 1
m = hashlib.md5()
while count < 4: # 循环3次
uesrname = input('请输入你的用户名:')
password_ming = (input('请输入您的密码:'))
m.update(password_ming.encode('utf-8')) # 将输入的密码传入到md5的对象m中
password = m.hexdigest() # 将16进制形式的密码赋值给password
mulu_path = os.path.dirname(os.path.dirname(__file__)) # 当前目录路径
file_path = os.path.join(mulu_path, uesrname) # 拼接成文件的路径
try: # 放在try中执行
fr = open(file_path, 'r')
dict_file = json.load(fr)
fr.close()
guoqishijian_tuple = t.strptime(dict_file['expire_date'], '%Y-%m-%d') # 时间 元祖
guoqishijian = t.mktime(guoqishijian_tuple) # 过期时间的时间戳
now_time = t.time() # 当前时间的 时间戳
if dict_file['status'] == 0: # 判断是否状态为0
if password == dict_file['password']: # 判断是否登陆成功
if now_time < guoqishijian: # 判断是否过期
print('登陆成功')
func(*arg)
break
else:
print('已过期')
break
else:
if count == 3: # 如果输入了三次将dict_file['status'] = 1写入文件
print('账号已锁定')
with open(file_path, 'w', encoding='utf-8')as f:
dict_file['status'] = 1
json.dump(dict_file, f)
break
else: # 否则提示密码错误
print('密码错误')
else:
print('已锁定') # 否则提示已过期退出程序
break
except Exception as e:
print('出错了{}'.format(e.args))
count += 1 # count放在这里,如果文件名输入错误三次也退出
return login_decorator
"""
最近alex买了个Tesla Model S,通过转账的形式,并且支付了5%的手续费,tesla价格为95万。账户文件为json,请用程序实现该转账行为。
需求如下:
目录结构为
.
├── account
│ ├── alex.json
│ └── tesla_company.json
└── bin
└── start.py
当执行start.py时,出现交互窗口
———- ICBC Bank ————-.
1. 账户信息
2. 转账
选择1 账户信息 显示alex的当前账户余额。
选择2 转账 直接扣掉95万和利息费用并且tesla_company账户增加95万
"""
@login_youhua_hash
def bug_car(alex_yu_e, tesla_monkey):
"""
不同目录购买特斯拉
:param alex_yu_e: 你的余额
:param tesla_monkey: 购买特斯拉所需要的钱
:return:
"""
select_num = input(
(
"""
———- ICBC Bank ————-.
1. 账户信息
2. 转账
""")
)
now_mulu_path = os.path.dirname(os.path.dirname(__file__)) # 当前所在目录
alex_path = os.path.join(now_mulu_path, r'account\alex.json') # 你的余额所在json文件路径
tesla_path = os.path.join(now_mulu_path, r'account\tesla_company.json') # 特斯拉账户所在json文件路径
with open(alex_path, 'w', encoding='utf-8')as f:
json.dump({"alex_balace": alex_yu_e}, f) # 写入余额
with open(alex_path, 'r')as f:
yue_dict = json.load(f) # 将余额读取出来dict
if select_num == str(1):
print('alex当前余额:{}'.format(yue_dict['alex_balace'])) # 选择1时返回你的余额
elif select_num == str(2): # 选择2时将从你的余额扣除,特斯拉账户相应增加
buy_yue = (int(yue_dict['alex_balace']) - tesla_monkey) - (tesla_monkey * 0.05) # 你购买 特斯拉+税后 还剩下余额
if buy_yue >= 0 :
with open(alex_path, 'w', encoding='utf-8')as f: # 写入还剩下的余额
json.dump({"alex_balace": buy_yue}, f)
with open(tesla_path, 'w', encoding='utf-8')as f: # 往特斯拉账户写入余额
json.dump({"tesla_balace": tesla_monkey}, f)
print('购买tesla成功')
else:
print('你的余额不够买一辆特斯拉,穷鬼!!!')
bug_car(1000000, 950000)
# withdraw.withdraw_start(yue=1000000, line_of_credit=500000, withdraw_monkey=450000)
# print(os.path.dirname(os.path.dirname(__file__)))
import os
import json
import sys
sys.path.append(r'..\bin')# 将bin目录添加到path中
"""
对上题增加一个需求:提现。
目录结构如下
.
├── account
│ └── alex.json
│ └── tesla_company.json
├── bin
│ └── start.py
└── core
└── withdraw.py
当执行start.py时,出现交互窗口
———- ICBC Bank ————-
1. 账户信息
2. 提现
选择1 账户信息 显示alex的当前账户余额和信用额度。
选择2 提现 提现金额应小于等于信用额度,利息为5%,提现金额为用户自定义。
体现代码的实现要写在withdraw.py里
"""
def withdraw_start(yue, line_of_credit, withdraw_monkey):
"""
实现一个提现的需求
:param yue: 当前余额
:param line_of_credit: 信用额度
:param withdraw_monkey: 提现金额
:return:
"""
select_num = input(
"""
———- ICBC Bank ————-
1. 账户信息
2. 提现
"""
)
yue, line_of_credit, withdraw_monkey = int(yue), int(line_of_credit), int(withdraw_monkey) # 类型转换
now_on_mulu = os.path.dirname(os.path.dirname(__file__))
alex_path = os.path.join(now_on_mulu, r'account\alex.json')
with open(alex_path, 'w', encoding='utf-8')as f:
json.dump({'alex_balace': yue, 'line_of_credit': line_of_credit}, f) # 写入余额,信用额度
with open(alex_path, 'r')as f:
alex_dict = json.load(f)
alex_yue = alex_dict['alex_balace'] # 你的当前余额
alex_line_of_credit = alex_dict['line_of_credit'] # 你的信用额度
if select_num == str(1):
print('alex当前余额:{}'.format(alex_yue))
elif select_num == str(2):
if str(withdraw_monkey) > str(alex_line_of_credit): # 判断提现金额是否小于信用额度
print('你的胃口也太大了吧 ,不知道你的信用额度就这么点么!!!')
else:
alex_withdraw_monkey = alex_yue + withdraw_monkey # 提现后的金额=当前余额+提现金额
alex_line_of_credit = line_of_credit - withdraw_monkey - (withdraw_monkey * 0.05) # 提现后信用额度=信用额度-提现金额-利息
with open(alex_path, 'w', encoding='utf-8')as f:
json.dump({'alex_balace': alex_withdraw_monkey, 'line_of_credit': alex_line_of_credit}, f)
print("""
提现成功:{}
当前账户余额:{}
当前信用额度:{}
""".format(withdraw_monkey, alex_withdraw_monkey, alex_line_of_credit))
else:
print('输入错误了哈!,认真读题~~~')
withdraw_start(yue=1000000, line_of_credit=500000, withdraw_monkey=450000)
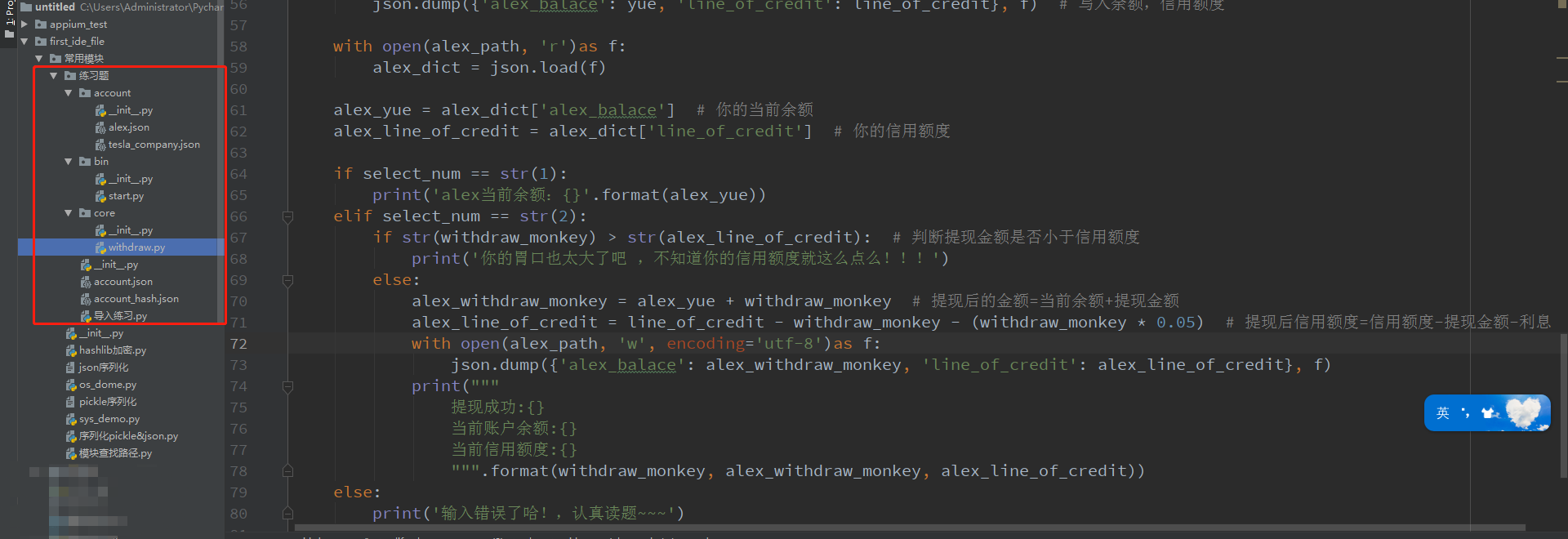