整理各种查询语句,需要查询数据请参考:
https://blog.csdn.net/chennuan1991/article/details/105240457
基础查询语句,请参考:
https://blog.csdn.net/chennuan1991/article/details/105250429
分组查询
01 查询每门课程的平均成绩
mysql> select avg(degree) from score group by cno;
+-------------+
| avg(degree) |
+-------------+
| 85.3333 |
| 76.3333 |
| 81.6667 |
+-------------+
3 rows in set (0.00 sec)
02 查询score表中至少有2名学生选修,并以3开头的课程的平均分数
mysql> select cno,avg(degree),count(*) from score group by cno having count(cno)>=2 and cno like '3%';
+-------+-------------+----------+
| cno | avg(degree) | count(*) |
+-------+-------------+----------+
| 3-105 | 85.3333 | 3 |
| 3-245 | 76.3333 | 3 |
+-------+-------------+----------+
2 rows in set (0.00 sec)
03 查询至少有2名男生的班号
mysql> select class from students where ssex ='男' group by class having count(*)>1;
+-------+
| class |
+-------+
| 95031 |
| 95033 |
+-------+
2 rows in set (0.00 sec)
04 查询students表中不姓王的同学记录
mysql> select * from students where sname not like '王%';
+-----+--------+------+---------------------+-------+
| sno | sname | ssex | birthday | class |
+-----+--------+------+---------------------+-------+
| 101 | 张三 | 男 | 1977-09-01 00:00:00 | 95033 |
| 102 | 李四 | 男 | 1975-10-02 00:00:00 | 95031 |
| 104 | 铁柱 | 男 | 1976-02-20 00:00:00 | 95033 |
| 105 | 小丽 | 女 | 1975-02-10 00:00:00 | 95031 |
| 106 | 小红 | 男 | 1974-06-03 00:00:00 | 95031 |
| 107 | 小绿 | 男 | 1976-02-20 00:00:00 | 95033 |
| 108 | 大妞 | 女 | 1975-02-10 00:00:00 | 95031 |
| 109 | 乖乖 | 男 | 1974-06-03 00:00:00 | 95031 |
+-----+--------+------+---------------------+-------+
8 rows in set (0.00 sec)
多表查询
01 查询所有学生的sname,cno,degree列(先分表查,再把两个表相等的地方连起来)
扫描二维码关注公众号,回复:
10467691 查看本文章
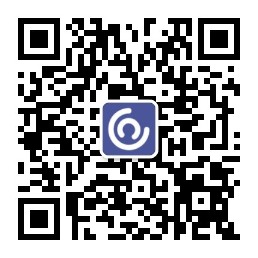
mysql> select sname,cno,degree from score,students where score.sno = students.sno;
+--------+-------+--------+
| sname | cno | degree |
+--------+-------+--------+
| 王五 | 3-105 | 92 |
| 王五 | 3-245 | 86 |
| 王五 | 6-166 | 85 |
| 小丽 | 3-105 | 88 |
| 小丽 | 3-245 | 75 |
| 小丽 | 6-166 | 79 |
| 乖乖 | 3-105 | 76 |
| 乖乖 | 3-245 | 68 |
| 乖乖 | 6-166 | 81 |
+--------+-------+--------+
9 rows in set (0.00 sec)
02 查询所有学生的sno,cname,degree列
mysql> select sno,cname,degree from score,course where course.cno = score.cno;
+-----+-----------------+--------+
| sno | cname | degree |
+-----+-----------------+--------+
| 103 | 计算机导论 | 92 |
| 103 | 操作系统 | 86 |
| 103 | 数字电路 | 85 |
| 105 | 计算机导论 | 88 |
| 105 | 操作系统 | 75 |
| 105 | 数字电路 | 79 |
| 109 | 计算机导论 | 76 |
| 109 | 操作系统 | 68 |
| 109 | 数字电路 | 81 |
+-----+-----------------+--------+
9 rows in set (0.00 sec)
03 查询选修某课程的同学人数多于5人的教师姓名
步骤1:查询选修大于5人的课程号cno
mysql> select cno from score group by cno having count(*)>5;
+-------+
| cno |
+-------+
| 6-166 |
+-------+
1 row in set (0.00 sec)
步骤2: 根据cno查询tno
mysql> select tno from course where cno = (select cno from score group by cno having count(*)>5);
+------+
| tno |
+------+
| 856 |
+------+
1 row in set (0.00 sec)
步骤3:根据tno查询tname
mysql> select tno,tname from teacher where tno =(select tno from course where cno = (select cno from score group by cno having count(*)>5));
+-----+--------+
| tno | tname |
+-----+--------+
| 856 | 张丹 |
+-----+--------+
1 row in set (0.00 sec)
三表关联查询
01 查询所有学生的sname,cname,degree列(找到3张表可以连接在一起的表)
mysql> select sname,cname,degree from students,course,score where students.sno = score.sno and course.cno =score.cno;
+--------+-----------------+--------+
| sname | cname | degree |
+--------+-----------------+--------+
| 王五 | 计算机导论 | 92 |
| 王五 | 操作系统 | 86 |
| 王五 | 数字电路 | 85 |
| 小丽 | 计算机导论 | 88 |
| 小丽 | 操作系统 | 75 |
| 小丽 | 数字电路 | 79 |
| 乖乖 | 计算机导论 | 76 |
| 乖乖 | 操作系统 | 68 |
| 乖乖 | 数字电路 | 81 |
+--------+-----------------+--------+
9 rows in set (0.00 sec)
子查询
01 查询95031班学生每门课程的平均分
步骤1:查询出95031班有多少学生
mysql> select sno from students where class='95031';
+-----+
| sno |
+-----+
| 102 |
| 105 |
| 106 |
| 108 |
| 109 |
+-----+
5 rows in set (0.00 sec)
步骤2:查询出在95031班学生的所有成绩
mysql> select cno,degree,sno from score where sno in (select sno from students where class ='95031');
+-------+--------+-----+
| cno | degree | sno |
+-------+--------+-----+
| 3-105 | 88 | 105 |
| 3-245 | 75 | 105 |
| 6-166 | 79 | 105 |
| 3-105 | 76 | 109 |
| 3-245 | 68 | 109 |
| 6-166 | 81 | 109 |
+-------+--------+-----+
6 rows in set (0.00 sec)
步骤3:以课程分组,计算出每科的平均值
mysql> select cno,avg(degree)from score where sno in (select sno from students where class ='95031') group by cno;
+-------+-------------+
| cno | avg(degree) |
+-------+-------------+
| 3-105 | 82.0000 |
| 3-245 | 71.5000 |
| 6-166 | 80.0000 |
+-------+-------------+
3 rows in set (0.01 sec)
02 查询选修‘3-105’课程的成绩高于‘109’号同学‘3-105’成绩的所有同学的记录
步骤1:查询109号同学3-105的成绩,作为子查询的条件
mysql> select degree from score where sno ='109' and cno ='3-105';
+--------+
| degree |
+--------+
| 76 |
+--------+
1 row in set (0.00 sec)
步骤2:使用上面的查询结果作为条件进行最后的查询
mysql> select * from score where degree>(select degree from score where sno ='109' and cno ='3-105') and cno = '3-105';
+-----+-------+--------+
| sno | cno | degree |
+-----+-------+--------+
| 103 | 3-105 | 92 |
| 105 | 3-105 | 88 |
+-----+-------+--------+
2 rows in set (0.00 sec)
03 查询出‘计算机系’教师所教课程的成绩表
mysql> select tno from teacher where tdepar = '计算机系';
+-----+
| tno |
+-----+
| 804 |
| 825 |
+-----+
2 rows in set (0.00 sec)
mysql> select cno from course where tno in(select tno from teacher where tdepar = '计算机系');
+-------+
| cno |
+-------+
| 3-245 |
| 3-105 |
+-------+
2 rows in set (0.00 sec)
mysql> select cno,degree from score where cno in(select cno from course where tno in(select tno from teacher where tdepar = '计算机系'));
+-------+--------+
| cno | degree |
+-------+--------+
| 3-245 | 86 |
| 3-245 | 75 |
| 3-245 | 68 |
| 3-105 | 92 |
| 3-105 | 88 |
| 3-105 | 76 |
+-------+--------+
6 rows in set (0.00 sec)
参考:
感谢code 158 编程俱乐部