- 上一篇文章,我们说了一个生成pdf,这次我们再web项目中导出pdf文件
1、导入依赖
<dependency>
<groupId>com.lowagie</groupId>
<artifactId>itext</artifactId>
<version>2.1.7</version>
</dependency>
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itext-asian</artifactId>
<version>5.2.0</version>
</dependency>
2、实体类
package com.example.demo.entity;
import lombok.Data;
@Data
public class Product {
private String productName;
private String productCode;
private float price;
public Product(String productName,String productCode,float price){
this.productName = productName;
this.productCode = productCode;
this.price = price;
}
}
3、controller
package com.example.demo.controller;
import com.example.demo.entity.Product;
import com.example.demo.entity.ViewPDF;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.ModelAndView;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Controller
@RequestMapping("/")
public class PrintPdfController {
@RequestMapping("printPdf")
public ModelAndView printPdf(){
Product product1 = new Product("产品一","cp01",120);
Product product2 = new Product("产品一","cp01",120);
Product product3 = new Product("产品一","cp01",120);
List<Product> products = new ArrayList<>();
products.add(product1);
products.add(product2);
products.add(product3);
Map<String, Object> model = new HashMap<>();
model.put("sheet", products);
return new ModelAndView(new ViewPDF(), model);
}
}
4、工具类
package com.example.demo.util;
import com.example.demo.entity.Product;
import com.lowagie.text.Document;
import com.lowagie.text.DocumentException;
import com.lowagie.text.Font;
import com.lowagie.text.Phrase;
import com.lowagie.text.pdf.BaseFont;
import com.lowagie.text.pdf.PdfPCell;
import com.lowagie.text.pdf.PdfPTable;
import com.lowagie.text.pdf.PdfWriter;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.List;
public class PdfUtil {
public void createPDF(Document document, PdfWriter writer, List<Product> products) throws IOException {
try {
document.addTitle("sheet of product");
document.addAuthor("scurry");
document.addSubject("product sheet.");
document.addKeywords("product.");
document.open();
PdfPTable table = createTable(writer,products);
document.add(table);
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (DocumentException e) {
e.printStackTrace();
} finally {
document.close();
}
}
public static PdfPTable createTable(PdfWriter writer,List<Product> products) throws IOException, DocumentException {
PdfPTable table = new PdfPTable(3);
PdfPCell cell;
int size = 20;
Font font = new Font(BaseFont.createFont("C://Windows//Fonts//simfang.ttf", BaseFont.IDENTITY_H,
BaseFont.NOT_EMBEDDED));
for(int i = 0;i<products.size();i++) {
cell = new PdfPCell(new Phrase(products.get(i).getProductCode(),font));
cell.setFixedHeight(size);
table.addCell(cell);
cell = new PdfPCell(new Phrase(products.get(i).getProductName(),font));
cell.setFixedHeight(size);
table.addCell(cell);
cell = new PdfPCell(new Phrase(products.get(i).getPrice()+"",font));
cell.setFixedHeight(size);
table.addCell(cell);
}
return table;
}
}
5、PDF类
package com.example.demo.entity;
import com.example.demo.util.PdfUtil;
import com.lowagie.text.Document;
import com.lowagie.text.pdf.PdfWriter;
import org.springframework.web.servlet.view.document.AbstractPdfView;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.Date;
import java.util.List;
import java.util.Map;
public class ViewPDF extends AbstractPdfView {
@Override
protected void buildPdfDocument(Map<String, Object> model, Document document, PdfWriter writer,
HttpServletRequest request, HttpServletResponse response) throws Exception {
String fileName = new Date().getTime()+"_quotation.pdf";
response.setCharacterEncoding("UTF-8");
response.setContentType("application/pdf");
response.setHeader("Content-Disposition","attachment;filename=" + new String(fileName.getBytes(), "iso8859-1"));
List<Product> products = (List<Product>) model.get("sheet");
PdfUtil pdfUtil = new PdfUtil();
pdfUtil.createPDF(document, writer, products);
}
}
注意问题
- 这里的Document对象是import com.lowagie.text.Document;
- 当请求头是: response.setHeader(“Content-Disposition”,“filename=” + new String(fileName.getBytes(), “iso8859-1”));
显示页面
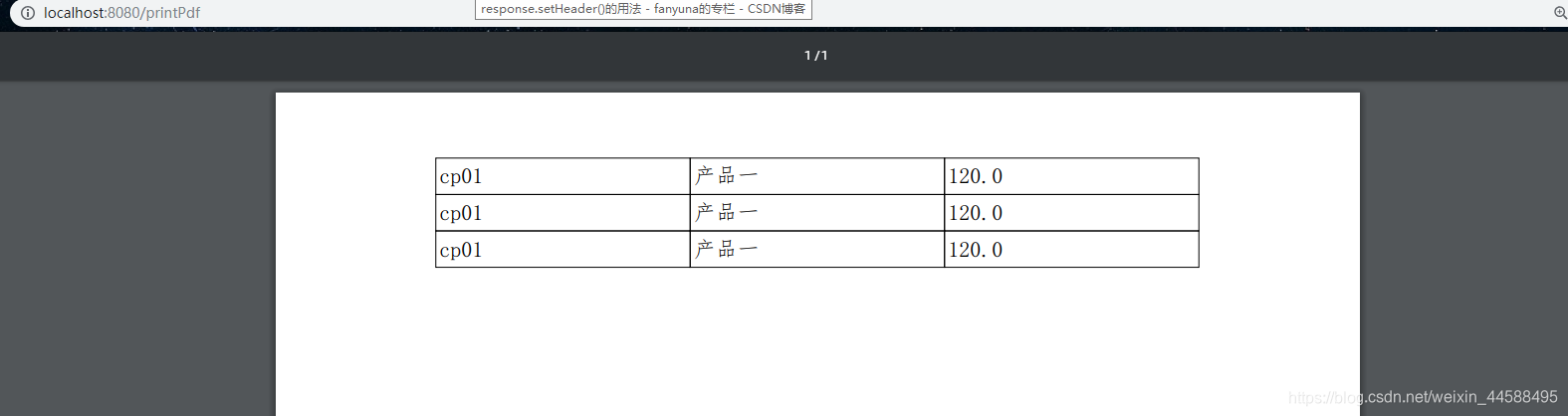
- 当是 response.setHeader(“Content-Disposition”,“attachment;filename=” + new String(fileName.getBytes(), “iso8859-1”));
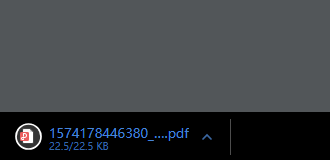