一、mybatis下载
下载地址:https://github.com/mybatis/mybatis-3/releases
mybatis-3.2.7.jar mybatis的核心包
lib文件夹 mybatis的依赖包所在
mybatis-3.2.7.pdf mybatis使用手册
二、创建java工程加入jar包
加入mybatis核心包、lib里面的所有的依赖包、数据库驱动包
三、加入配置文件
SqlMapConfig.xml:
扫描二维码关注公众号,回复:
1033109 查看本文章
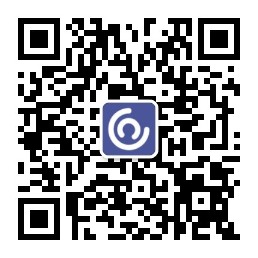
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!-- 别名 --> <typeAliases> <!-- <typeAlias type="com.itheima.mybatis.pojo.User" alias="User"/> --> <package name="com.itheima.mybatis.pojo"/> </typeAliases> <!-- 和spring整合后environment配置将废除 --> <environments default="development"> <environment id="development"> <!-- 使用jdbc事务管理 --> <transactionManager type="JDBC"/> <!-- 数据库连接池 --> <dataSource type="POOLED"> <property name="driver" value="com.mysql.jdbc.Driver"/> <property name="url" value="jdbc:mysql://localhost:3306/mybatis?characterEncoding=utf-8"/> <property name="username" value="root"/> <property name="password" value=""/> </dataSource> </environment> </environments> <!-- mapper的文件位置 --> <mappers> <mapper resource="User.xml"/> </mappers> </configuration>
log4j.properties:
# Global logging configuration log4j.rootLogger=DEBUG, stdout # Console output... log4j.appender.stdout=org.apache.log4j.ConsoleAppender log4j.appender.stdout.layout=org.apache.log4j.PatternLayout log4j.appender.stdout.layout.ConversionPattern=%5p [%t] - %m%n
四、创建实体类
public class User implements Serializable { private static final long serialVersionUID = 1L; private Integer id; private String username;// 用户姓名 private String sex;// 性别 private Date birthday;// 生日 private String address;// 地址
五、sql映射文件
在src下面创建User.xml或者在创建mapper接口的包里面创建并且名字和接口名字相同
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org.//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <!-- namespace命名空间,用于隔离sql --> <mapper namespace="user"> <!-- 通过id查询一个用户 --> <select id="findUserById" parameterType="Integer" resultType="com.itheima.mybatis.pojo.User"> select * from user where id=#{id} </select> <!-- 根据用户名称模糊查询用户列表 #{}占位符 ${}字符串拼接 --> <select id="findUserByUsername" parameterType="String" resultType="com.itheima.mybatis.pojo.User"> <!-- select * from user where username like '%${value}%'--> select * from user where username like "%"#{v}"%" <!--两条sql语句是一样的,第一句是select * from user where username like '%五%' 第二句是select * from user where uername like "%"'五'"%" --> </select> <!-- 添加用户 --> <insert id="insertUser" parameterType="com.itheima.mybatis.pojo.User"> insert into user(username,birthday,address,sex) values (#{username},#{birthday},#{address},#{sex}) </insert> <!-- 更新用户 --> <update id="updateUserById" parameterType="com.itheima.mybatis.pojo.User"> update user set username=#{username},sex=#{sex},birthday=#{birthday},address=#{address} where id=#{id} </update> <!-- 删除 --> <delete id="deleteUserById" parameterType="Integer"> delete from user where id=#{id} </delete> </mapper>
六、测试类
public class MybatisFirstTest { @Test public void testname() throws IOException{ //加载核心配置文件 String resource="sqlMapConfig.xml"; InputStream in=Resources.getResourceAsStream(resource); //创建一个sqlsessionfactory SqlSessionFactory sqlSessionFactory=new SqlSessionFactoryBuilder().build(in); //创建sqlsession SqlSession sqlSession=sqlSessionFactory.openSession(); //执行sql语句 User user=sqlSession.selectOne("user.findUserById",10); System.out.println(user); } //根据用户名称模糊查询用户列biao @Test public void testfindUserByUsername() throws IOException{ //加载核心配置文件 String resource="sqlMapConfig.xml"; InputStream in=Resources.getResourceAsStream(resource); //创建一个sqlsessionfactory SqlSessionFactory sqlSessionFactory=new SqlSessionFactoryBuilder().build(in); //创建sqlsession SqlSession sqlSession=sqlSessionFactory.openSession(); //执行sql语句 List<User> user=sqlSession.selectList("user.findUserByUsername","五"); for(User user2:user){ System.out.println(user2); } } //添加用户 @Test public void testInsertUser() throws IOException{ //加载核心配置文件 String resource="sqlMapConfig.xml"; InputStream in=Resources.getResourceAsStream(resource); //创建一个sqlsessionfactory SqlSessionFactory sqlSessionFactory=new SqlSessionFactoryBuilder().build(in); //创建sqlsession SqlSession sqlSession=sqlSessionFactory.openSession(); //执行sql语句 User user=new User(); user.setUsername("曾志伟"); user.setBirthday(new Date()); user.setAddress("河南"); user.setSex("男"); int i=sqlSession.insert("user.insertUser",user); sqlSession.commit(); } //更新用户 @Test public void testUpdateUserById() throws IOException{ //加载核心配置文件 String resource="sqlMapConfig.xml"; InputStream in=Resources.getResourceAsStream(resource); //创建一个sqlsessionfactory SqlSessionFactory sqlSessionFactory=new SqlSessionFactoryBuilder().build(in); //创建sqlsession SqlSession sqlSession=sqlSessionFactory.openSession(); //执行sql语句 User user=new User(); user.setId(29); user.setUsername("何炅"); user.setBirthday(new Date()); user.setAddress("湖南"); user.setSex("男"); int i=sqlSession.update("user.updateUserById",user); sqlSession.commit(); } //删除 @Test public void testDelete() throws IOException{ //加载核心配置文件 String resource="sqlMapConfig.xml"; InputStream in=Resources.getResourceAsStream(resource); //创建一个sqlsessionfactory SqlSessionFactory sqlSessionFactory=new SqlSessionFactoryBuilder().build(in); //创建sqlsession SqlSession sqlSession=sqlSessionFactory.openSession(); sqlSession.delete("user.deleteUserById",29); sqlSession.commit(); } }