代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
namespace WindowsFormsApp24
{
public partial class Form1 : Form
{
public CityList list = new CityList();
public Form1()
{
InitializeComponent();
}
private void label1_Click(object sender, EventArgs e)
{
}
private void textBox1_TextChanged(object sender, EventArgs e)
{
}
private void button5_Click(object sender, EventArgs e)
{
try
{
CityData data = this.makeData();
int index = Convert.ToInt32(listBox3.Text);
list[index] = data;
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
this.Update();
}
private CityData makeData()
{
string name = textBox1.Text;
int x = 0;
int y = 0;
try
{
x = Convert.ToInt32(textBox2.Text);
y = Convert.ToInt32(textBox3.Text);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
CityData city = new CityData(x, y, name);
return city;
}
public void Update()
{
textBox4.Clear();
for (int i = 0; i < this.list.Length; i++)
{
CityData childData = list[i];
textBox4.AppendText(list[i].name + "\t" + list[i].X + "\t" + list[i].Y + "\n");
}
}
private void listBox1_SelectedIndexChanged(object sender, EventArgs e)
{
}
private void label6_Click(object sender, EventArgs e)
{
}
private void label7_Click(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
CityData data = this.makeData();
this.list.InsertAtFist(data);
this.Update();
}
private void button2_Click(object sender, EventArgs e)
{
CityData data = this.makeData();
this.list.InsertAtRear(data);
this.Update();
}
private void button3_Click(object sender, EventArgs e)
{
try
{
CityData data = this.makeData();
int index = Convert.ToInt32(listBox1.Text);
this.list.Insert(index, data);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
this.Update();
}
private void button4_Click(object sender, EventArgs e)
{
try
{
int index = Convert.ToInt32(listBox2.Text);
this.list.Remove(index);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
this.Update();
}
private void button7_Click(object sender, EventArgs e)
{
textBox8.Clear();
try
{
int pointX = Convert.ToInt32(textBox9.Text);
int pointY = Convert.ToInt32(textBox10.Text);
int distance = Convert.ToInt32(textBox11.Text);
this.list.SearchCity(distance, pointX, pointY);
int len = this.list.dataInfo.Length;
string str = "";
for (int i = 0; i < len; i++)
{
if (list.dataInfo[i] != null)
{
str += list.distances[i].ToString("0.00") + "\t" + list.dataInfo[i].name + "\t" + list.dataInfo[i].X + "\t" + list.dataInfo[i].Y + "\n";
}
textBox8.Text = str;
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void button6_Click(object sender, EventArgs e)
{string name = textBox5.Text;
CityData data = this.list.SearchPosInfo(name);
if (data == null)
{
MessageBox.Show("并没有该城市");
}
else
{
textBox6.Text = data.X.ToString();
textBox7.Text = data.Y.ToString();
}
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void textBox4_TextChanged(object sender, EventArgs e)
{
}
public class CityData : IComparable<CityData>
{
public int X;
public int Y;
public string name;
public CityData(int x, int y, string name)
{
this.X = x;
this.Y = y;
this.name = name;
}
public int CompareTo(CityData other)
{
return other.X == X ? 0 : -1;
}
}
public class CityList : SLinkList<CityData>
{
public CityData[] dataInfo = new CityData[100];
public double[] distances = new double[100];
private int CDLen = 0;
public CityData SearchPosInfo(string name)
{
SNode<CityData> temp = this._pHead;
for (int i = 0; i < this._length; i++)
{
if (temp.Data.name == name)
{
return Locate(i).Data;
}
temp = temp.Next;
}
return null;
}
public void SearchCity(int distance, int pointX, int pointY)
{
SNode<CityData> temp = this._pHead;
for (int i = 0; i < this._length; i++)
{
double dis = Math.Sqrt(Math.Pow((temp.Data.X - pointX), 2) + Math.Pow((temp.Data.Y - pointY), 2));
if (dis <= distance)
{
dataInfo[this.CDLen++] = temp.Data;
distances[this.CDLen - 1] = dis;
}
temp = temp.Next;
}
}
internal void InsertAtFirst(CityData data)
{
throw new NotImplementedException();
}
internal void InsertAtEnd(CityData data)
{
throw new NotImplementedException();
}
}
}
}
运行结果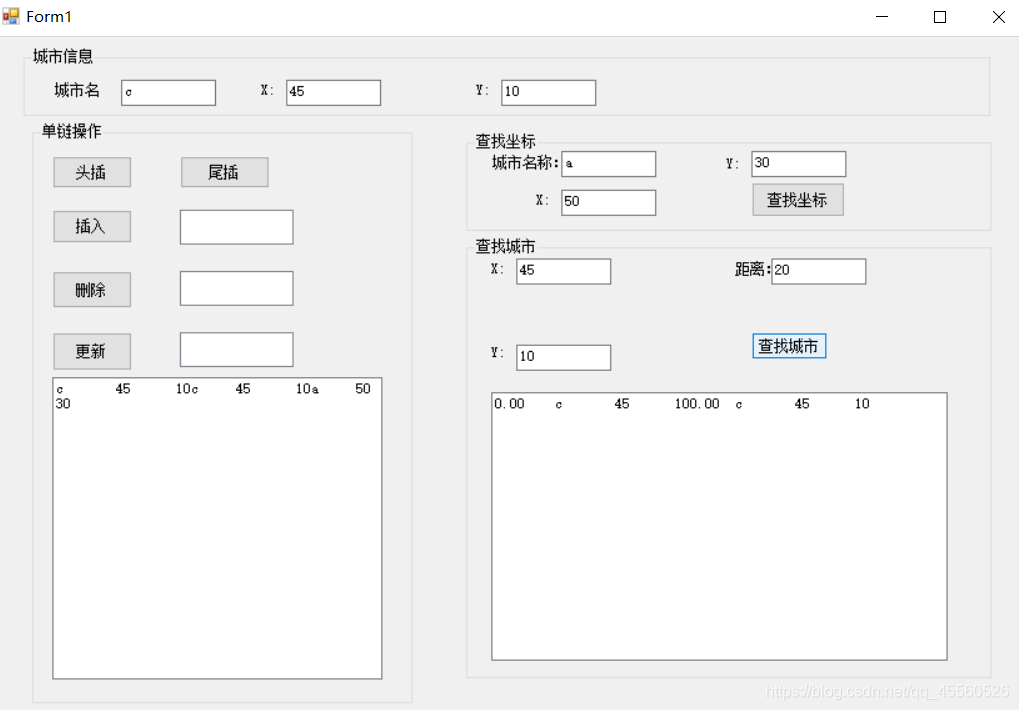
备注:关于listbox的问题我实在是没有研究出来以后再改吧