//针对ES6规范(第1-5条)start
1.块级作用域
let/const取代var;
在let和const之间,建议优先使用const,尤其是在全局环境,不应该设置变量,只应设置常量。
2.解构赋值
1)使用数组成员对变量赋值时,优先使用解构赋值。
e.g.
const arr = [1, 2, 3, 4];
// bad
const first = arr[0];
const second = arr[1];
// good
const [first, second] = arr;
2)函数的参数如果是对象的成员,优先使用解构赋值。
e.g.
// bad
function getFullName(user) {
const firstName = user.firstName;
const lastName = user.lastName;
}
// good
function getFullName(obj) {
const { firstName, lastName } = obj;
}
// best
function getFullName({ firstName, lastName }) {
}
3)如果函数返回多个值,优先使用对象的解构赋值,而不是数组的解构赋值。这样便于以后添加返回值,以及更改返回值的顺序。
e.g.
// bad
function processInput(input) {
return [left, right, top, bottom];
}
// good
function processInput(input) {
return { left, right, top, bottom };
}
const { left, right } = processInput(input);
3.对象
单行定义的对象,最后一个成员不以逗号结尾。多行定义的对象,最后一个成员以逗号结尾。
e.g.
// bad
const a = { k1: v1, k2: v2, };
const b = {
k1: v1,
k2: v2
};
// good
const a = { k1: v1, k2: v2 };
const b = {
k1: v1,
k2: v2,
};
4.数组
1)使用扩展运算符(...)拷贝数组。
e.g.
// bad
const len = items.length;
const itemsCopy = [];
let i;
for (i = 0; i < len; i++) {
itemsCopy[i] = items[i];
}
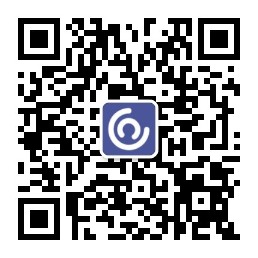
// good
const itemsCopy = [...items];
2)使用 Array.from 方法,将类似数组的对象转为数组。
e.g.
const foo = document.querySelectorAll('.foo');
const nodes = Array.from(foo);
5.Class
1)总是用 Class,取代需要 prototype 的操作。因为 Class 的写法更简洁,更易于理解。
e.g.
// good
class Queue {
constructor(contents = []) {
this._queue = [...contents];
}
pop() {
const value = this._queue[0];
this._queue.splice(0, 1);
return value;
}
}
2)使用extends实现继承,因为这样更简单,不会有破坏instanceof运算的危险。
e.g.
// bad
const inherits = require('inherits');
function PeekableQueue(contents) {
Queue.apply(this, contents);
}
inherits(PeekableQueue, Queue);
PeekableQueue.prototype.peek = function() {
return this._queue[0];
}
// good
class PeekableQueue extends Queue {
peek() {
return this._queue[0];
}
}
//针对ES6规范end
6.分号与逗号
赋值,定义,返回值,方法调用后强制需要加分号
7.语句块内的函数声明
切勿在语句块内声明函数,在 ES5 的严格模式下,这是不合法的。函数声明应该在定义域的顶层。但在语句块内可将函数申明转化为函数表达式赋值给变量。
e.g.
//bad
if (x) {
function foo() {}
}
//good
if (x) {
var foo = function() {};
}
7.eval 函数
eval() 不但混淆语境还很危险,总会有比这更好、更清晰、更安全的另一种方案来写你的代码,因此尽量不要使用 eval 函数。
8.三元条件判断(if 的快捷方法)
用三元操作符分配或返回语句。在比较简单的情况下使用,避免在复杂的情况下使用。
e.g.
//bad
if(x === 10) {
return 'valid';
} else {
return 'invalid';
}
//good
return x === 10 ? 'valid' : 'invalid';
9.变量与声明
1)变量在函数内部或循环控制条件之前提前声明
e.g.
//bad
function test(){
for(var i=0;i<list.length;i++){
var item = list[i];
}
}
//good
function test(){
var i;
var item;
var len = list.length;
for(i=0;i<len;i++){
item = list[i];
}
}
2)变量命名规则 最好是具象的,布尔值需要带 is,has,can, 单位值需要带 _ms,_s,_px 等
//bad
var height = $("#id").height();
var delay = 3 *1000;
var readYet = false;
//good
var height_px = $("#id").height();
var delay_ms = 3 * 1000;
var isRead = false;
3)定义变量时,不使用逗号
//bad
var arr = [],str = "",obj = {};
//good
var arr = [];
var str = "";
var obj = {};
10.常量
1)常量需要大写,定义在文件头部,并使用 _ 分割
e.g.
//bad
var maxsize = 10;
//good
var MAX_SIZE = 10;
11.函数
1)函数内部不允许使用arguments.callee和arguments.caller
arguments.callee详见:https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Functions/arguments/callee
arguments.caller详见:https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Functions/arguments/caller
2)函数参数不得超过5个 (多于5个使用objectType代替)
//bad
function myTestFunc(a,b,c,d,e,f,g,h){}
//good
function myTestFunc(params){
var a = params.a;
var b = params.b;
}
12.注释
1)文件开头必须要有文件说明注释,时间,作者
/**
*@author hxl
*@date 20180228
*@fileoverview 本文件用于规范前端代码,保证可读性一致性。
*/
2)多行单行注释都被允许
.协议
不要指定引入资源所带的具体协议
e.g.
//bad
<script src="http://cdn.com/foundation.min.js"></script>
.example {
background: url(http://static.example.com/images/bg.jpg);
}
//good
<script src="//cdn.com/foundation.min.js"></script>
.example {
background: url(//static.example.com/images/bg.jpg);
}