说明
在编程中有一个原则,称为:Do not Repeat Yourself(DRY)
。重复代码问题的原因是因为任何更改都需要在多个地方修复代码。这通常意味着更多的程序员的工作量和更多的错误的空间。
请注意在下面的示例中,describe
方法由Bird
和Dog
共享:
Bird.prototype = {
constructor: Bird,
describe: function() {
console.log("我的名字是" + this.name);
}
};
Dog.prototype = {
constructor: Dog,
describe: function() {
console.log("我的名字是" + this.name);
}
};
describe
方法在两个地方重复了。可以通过创建一个名为Animal
的supertype
(或父类)来编辑代码以遵循DRY
原则:
function Animal() { };
Animal.prototype = {
constructor: Animal,
describe: function() {
console.log("我的名字是" + this.name);
}
};
由于Animal
包含describe
方法,你可以把它从Bird
和Dog
中删除:
Bird.prototype = {
constructor: Bird
};
Dog.prototype = {
constructor: Dog
};
练习
eat
方法在Cat
和Bear
中重复了。通过将eat
方法移动到Animal
的supertype
,以DRY
的原则编辑代码。
Animal.prototype
应该有eat
属性。Bear.prototype
不应该有eat
属性。Cat.prototype
不应该有eat
属性。
function Cat(name) {
this.name = name;
}
Cat.prototype = {
constructor: Cat,
eat: function() {
console.log("nom nom nom");
}
};
function Bear(name) {
this.name = name;
}
Bear.prototype = {
constructor: Bear,
eat: function() {
console.log("nom nom nom");
}
};
function Animal() { }
Animal.prototype = {
constructor: Animal,
};
答案
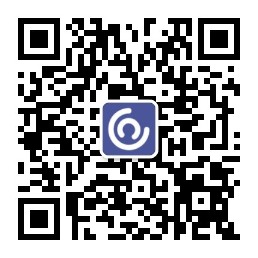
方法 | 描述 |
this | 当前执行代码的环境对象 |
prototype | 给对象添加属性和方法。 |
constructor | 返回对创建此对象的数组函数的引用。 |
console.log() | 用于在控制台输出信息(浏览器按下 F12 打开控制台)。 |
function Cat(name) {
this.name = name;
}
Cat.prototype = {
constructor: Cat,
// eat: function() {
// console.log("nom nom nom");
// }
};
function Bear(name) {
this.name = name;
}
Bear.prototype = {
constructor: Bear,
// eat: function() {
// console.log("nom nom nom");
// }
};
function Animal() { }
Animal.prototype = {
constructor: Animal,
eat: function() {
console.log("nom nom nom");
}
};