Description
Tom and Jerry are playing a game with tubes and pearls. The rule of the game is:
1) Tom and Jerry come up together with a number K.
2) Tom provides N tubes. Within each tube, there are several pearls. The number of pearls in each tube is at least 1 and at most N.
3) Jerry puts some more pearls into each tube. The number of pearls put into each tube has to be either 0 or a positive multiple of K. After that Jerry organizes these tubes in the order that the first tube has exact one pearl, the 2nd tube has exact 2 pearls, …, the Nth tube has exact N pearls.
4) If Jerry succeeds, he wins the game, otherwise Tom wins.
Write a program to determine who wins the game according to a given N, K and initial number of pearls in each tube. If Tom wins the game, output “Tom”, otherwise, output “Jerry”.
Input
The first line contains an integer M (M<=500), then M games follow. For each game, the first line contains 2 integers, N and K (1 <= N <= 100, 1 <= K <= N), and the second line contains N integers presenting the number of pearls in each tube.
Output
For each game, output a line containing either “Tom” or “Jerry”.
Sample Input
2 5 1 1 2 3 4 5 6 2 1 2 3 4 5 5
Sample Output
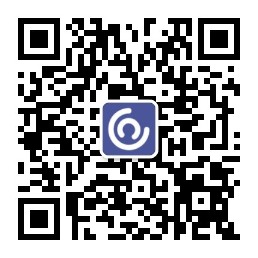
Jerry Tom
题解:有n个盒子,给你盒子初始各有几个豆子,每次可以在一个篮子里放k个豆子,问可能不可能放若干次后,有1个豆子,2个豆子。。。。。n-1个豆子,n个豆子的篮子各有一个。问你行不行。贪心,每个篮子每次+k,遇到之前没有这个数目的情况出现,就确定下来。
代码如下:
#include <iostream>
#include <cstdio>
#include <algorithm>
#include <cstring>
#include <cmath>
#define mem(a,b) memset(a,b,sizeof(a))
#define For(a,b) for(int a=0;a<b;a++)
using namespace std;
const int maxn = 1e3+500;
const int INF = 0x3f3f3f3f;
const int inf = 0x3f;
typedef long long ll;
const ll mod = 1e9+7;
char c[] = {"pqruvwxyz"};
int a[maxn];
int main() {
int t;
cin>>t;
while(t--)
{
int n,k,s[maxn]={0};
cin>>n>>k;
for(int i=0;i<n;i++)
{
cin>>a[i];
}
for(int i=0;i<n;i++)
{
while(1)
{
if(s[a[i]]==0)
{
s[a[i]]=1;
break;
}
if(a[i]>n)
break;
a[i]+=k;
}
}
sort(a,a+n);
int flag=0;
for(int i=0;i<n;i++)
if(a[i]==i+1)
flag++;
if(flag==n)
cout<<"Jerry"<<endl;
else
cout<<"Tom"<<endl;
}
return 0;
}