Spring 面向切面编程 (AOP)
参考资料: spring framework AOP 官方文档
1、什么是AOP (摘自百度百科)
在软件业,AOP为Aspect Oriented Programming的缩写,意为:面向切面编程,通过预编译方式和运行期动态代理实现程序功能的统一维护的一种技术。AOP是OOP的延续,是软件开发中的一个热点,也是Spring框架中的一个重要内容,是函数式编程的一种衍生范型。利用AOP可以对业务逻辑的各个部分进行隔离,从而使得业务逻辑各部分之间的耦合度降低,提高程序的可重用性,同时提高了开发的效率。
2、使用场景
AOP适用于开发共通性的业务逻辑,诸如日志采集、访问控制、事务处理等
一、AOP相关概念
名词 | 说明 |
---|---|
切面 (Aspect) | 跨越多个业务模块的功能关注点,对多个业务模块的横切面 |
连接点 (Join point) | 程序运行时的一个点,在spring AOP中,连接点表示一个执行的方法 |
通知(Advice) | 特定连接点的某个采集操作。指连接点方法调用过程中执行的代码,包含方法执行前、执行后、发生异常、最终和方法环绕 |
切入点(Pointcut) | 用于匹配连接点的谓词,用于对连接点进行拦截定义。通过切入点表达式,匹配合规的连接点。 |
目标对象(Target object) | 一个或多个切面的对象,也叫“切面对象”。由于spring AOP采用运行时代理实现,所以该对象始终是代理对象 |
个人理解
spring AOP编程,根据业务逻辑需要(如添加操作的日志采集),采用aop 语法规范构建一个业务切面,在匹配的切入点方法触发时,完成所需业务逻辑。(如在用户执行系统添加相关操作时,在方法执行后,记录添加日志)
二、AOP的使用
Spring 使用
在Spring中应用AOP需要启用 @AspectJ 的支持,语法如下
@Configuration
@EnableAspectJAutoProxy
public class AppConfig {
}
SpringBoot 基于注解的AOP
1、引入springboot的AOP依赖
compile ('org.springframework.boot:spring-boot-starter-aop')
以上语法为gradle引用,springboot引用依赖后,默认启动aop相关注解
2、定义切面类
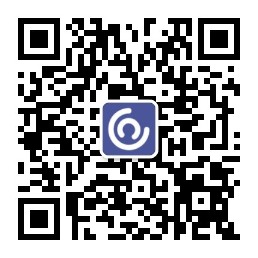
package org.items.tysite.common.aspect;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Component;
import java.lang.reflect.Modifier;
@Aspect (1)
@Component (2)
public class DemoAspect {
@Before(value = "execution(* org.items.tysite.demo.controller..*Controller.demoGet*(..))") (3)
public void demoBefore(JoinPoint joinPoint) {
logger.debug("目标方法名称:"+joinPoint.getSignature().getName());
}
}
(1) @Aspect 切面注解,声明当前类为一个切面
(2) @Component spring注解,声明该类为springboot的组件
(3) @Before(value = “execution(* org.items.tysite.demo.controller..Controller.demoGet(..))”)
public void demoPointcut() {}
定义切点
通过切入点表达式定义当前切面方法所需影响的程序代码
语法定义如下
@Pointcut(“execution(* transfer(..))”) // the pointcut expression
private void anyOldTransfer() {} // the pointcut signature
Spring AOP 支持的切入点表达式如下
- execution:用于匹配方法执行连接点。这是使用Spring AOP时使用的主要切入点指示符。
- within:限制匹配某些类型中的连接点(使用Spring AOP时在匹配类型中声明的方法的执行)。
- this:限制与连接点的匹配(使用Spring AOP时执行方法),其中bean引用(Spring AOP代理)是给定类型的实例。
- target:限制与连接点的匹配(使用Spring AOP时执行方法),其中目标对象(被代理的应用程序对象)是给定类型的实例。
- args:限制与连接点的匹配(使用Spring AOP时执行方法),其中参数是给定类型的实例。
- @target:限制与连接点的匹配(使用Spring AOP时执行方法),其中执行对象的类具有给定类型的注释。
- @args:限制与连接点的匹配(使用Spring AOP时执行方法),其中传递的实际参数的运行时类型具有给定类型的注释。
- @within:限制匹配到具有给定注释的类型中的连接点(使用Spring AOP时在具有给定注释的类型中声明的方法的执行)。
- @annotation:限制连接点的匹配,其中连接点的主题(在Spring AOP中执行的方法)具有给定的注释。
可以使用&&
、||
、!
语法组合管理切入点表达式,如下所示
@Pointcut(“execution(public * *(..))”)
private void anyPublicOperation() {}
anyPublicOperation
如果方法执行连接点表示任何公共方法的执行,则匹配。
@Pointcut(“within(com.xyz.someapp.trading..*)”)
private void inTrading() {}
inTrading
如果方法执行在交易模块中,则匹配。
@Pointcut(“anyPublicOperation() && inTrading()”)
private void tradingOperation() {}
tradingOperation
如果方法执行表示交易模块中的任何公共方法,则匹配。
以下示例显示了一些常见的切入点表达式
- 执行任何公共方法:
execution(public * *(..))
- 执行名称以以下开头的任何方法set:
execution(* set*(..))
- 执行AccountService接口定义的任何方法:
execution(* com.xyz.service.AccountService.*(..))
- 执行service包中定义的任何方法:
execution(* com.xyz.service.*.*(..))
- 执行服务包或其子包中定义的任何方法:
execution(* com.xyz.service..*.*(..))
- service包中的任何连接点(仅在Spring AOP中执行方法):
within(com.xyz.service.*)
- service包或其子包中的任何连接点(仅在Spring AOP中执行方法):
within(com.xyz.service..*)
- 代理实现AccountService接口的任何连接点(仅在Spring AOP中执行方法) :
this(com.xyz.service.AccountService)
(4) @Before(“demoPointcut()”)
定义通知
spring提供的advice(通知)注解,在定义时,可以直接以切入点表达式赋值,如下所示
@Before("execution(* org.items.tysite.demo.controller..*Controller.demoGet*(..))")
也可以先定义通知,再调用
@Pointcut("execution(* org.items.tysite.demo.controller..*Controller.demoGet*(..))")
public void demoPointcut() {}
@Before("demoPointcut()")
public void doBefore() {
……省略代码
}
Spring AOP的常用通知(advice)注解如下
-
@Before 该注解注释的方法,在 切入点匹配方法
执行之前
执行。
可以使用JoinPoint
作为参数,获取目标方法的类名、方法名、参数及返回类型信息
。@Before(“execution(* org.items.tysite.demo.controller..Controller.demoGet(..))”)
public void demoBefore(JoinPoint joinPoint) {
} -
@AfterReturning 该注解注释的方法,在切入点匹配方法
执行之后
执行。
可以通过returning
注解参数,获取目标方法的返回结果@AfterReturning( pointcut=“com.xyz.myapp.SystemArchitecture.dataAccessOperation()”,returning=“retVal”)
public void doAccessCheck(Object retVal) {
}
注意:returning的值必须与方法的参数命名一致。
目标方法抛出异常时,将导致本切面方法不执行 -
@AfterThrowing 该注解注释的方法,在切入点匹配方法
抛出异常时
执行
可以通过throwing
注解参数,获取目标方法抛出的异常@AfterThrowing(value = “execution(* org.items.tysite.demo.controller..Controller.demoGet(..))”, throwing = “ex”)
public void demoAfterThrowing(Exception ex) {} -
@After 该注解注释的方法,在切入点匹配方法
执行完成
后执行,无论是否抛出异常该注解注释的方法在 @AfterThrowing 或 @AfterReturning之前执行
-
@Around 该注解注释的方案,在切入点匹配方法 周围进行相关操作
@Around("execution(* org.items.tysite.demo.controller..*Controller.demoGet*(..))")
public Object demoAround(ProceedingJoinPoint pjp) throws Throwable {
try {
logger.debug("Around方案,目标方法执行前的操作");
Object retVal = pjp.proceed();
logger.debug("Around方案,目标方法执行后的操作");
return retVal;
} catch (Throwable throwable) {
logger.debug("Around方案,目标方法抛出异常时的操作");
throw throwable;
} finally {
logger.debug("Around方案, 目标方法执行结束后的操作");
}
}
注意:(1) 此方案的操作优先于以上4个注解执行
(2) 本方法必须返回结果,否则将导致 目标访问无法返回处理结果