How many layouts does Android have?
- LinearLayout (linear layout)
- RelativeLayout (relative layout)
- FrameLayout (frame layout)
- TableLayout (table layout)
- GridLayout (grid layout)
- AbsoluteLayout (absolute layout)
LinearLayout
LinearLayout, also known as linear layout, is a very commonly used layout.
Multiple views (here called subviews, subitems) can be placed in LinearLayout. The subview can be TextView, Button, or LinearLayout, RelativeLayout, etc. They will be arranged sequentially in a column or row. Next, some settings in xml are introduced.
First put a LinearLayout in xml.
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
</LinearLayout>
Some properties are introduced below.
Vertical arrangement and horizontal arrangement
Determine whether to arrange the subviews horizontally or vertically by setting the orientation. Optional values are vertical and horizontal.
vertical arrangement
Set orientation to vertical.
android:orientation="vertical"
horizontal arrangement
Set orientation to horizontal.
android:orientation="horizontal"
Arrangement gravity
Determine the arrangement of subviews. gravity
It means "gravity", which we extend to the direction in which the subview will move closer. gravity
There are several options to choose from. The commonly used ones are start, end, left, right, top, and bottom.
For example:
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="start"
android:orientation="vertical">
</LinearLayout>
Below are the gravity options. We will call LinearLayout the "parent view" or " container ".
constant | Define value | describe |
---|---|---|
top | 30 | Push the view to the top of the container. The size of the view will not be changed. |
bottom | 50 | Push the view to the bottom of the container. The size of the view will not be changed. |
center | 11 | The subview is centered both horizontally and vertically. The size of the view will not be changed. |
center_horizontal | 1 | The subview is centered horizontally. The size of the view will not be changed. |
center_vertical | 10 | The child view is vertically centered. The size of the view will not be changed. |
start | 800003 | Push the view to the beginning of the container. The size of the view will not be changed. |
end | 800005 | Put the child view at the end of the container. Does not change the size of the view. |
left | 3 | Subviews are arranged starting from the left side of the container. The size of the view will not be changed. |
right | 5 | Subviews are arranged starting from the right side of the container. The size of the view will not be changed. |
start and left, end and right do not necessarily have the same effect. For RTL (right to left) type mobile phones, such as some Arabic systems. start is from right to left. We rarely see RTL in our daily life, usually LTR. But it is still recommended to use start instead of left.
Gravity can set multiple values at the same time, |
connected with the or symbol. For example android:gravity="end|center_vertical"
.
<LinearLayout
android:layout_width="match_parent"
android:layout_height="60dp"
android:gravity="end|center_vertical"
android:orientation="vertical">
</LinearLayout>
layout_gravity of child view
layout_gravity looks somewhat similar to gravity.
android:gravity
Control its own internal child elements.android:layout_gravity
It tells the parent element its position.
The value range is the same as gravity. The meanings represented are also similar.
<LinearLayout
android:layout_width="match_parent"
android:layout_height="100dp"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#90CAF9"
android:text="none" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:background="#9FA8DA"
android:text="center" />
</LinearLayout>
Split proportion layout_weight
You can set the layout_weight of the subview to determine the space proportion. When setting layout_weight
, it is generally necessary to set layout_width="0dp"
.
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:background="#FFCC80"
android:orientation="horizontal">
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:background="#eaeaea"
android:gravity="center"
android:text="1" />
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="2" />
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:background="#eaeaea"
android:text="1" />
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="3"
android:background="#BEC0D1"
android:text="3" />
</LinearLayout>
The sum of the split proportions weightSum
android:weightSum
Defines the maximum value of the sum of weights of child views. If not specified directly, it will be layout_weight
the sum of all child views. If you want to give a single subview half of the space, you can set the subview's layout_weight to 0.5, and set the LinearLayout's weightSum to 1.0.
The value can be a floating point number, for example 9.3
.
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:background="#4DB6AC"
android:weightSum="9.3"
android:orientation="horizontal">
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="4.6"
android:background="#eaeaea"
android:gravity="center"
android:text="4.6" />
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="#7986CB"
android:layout_weight="2.5"
android:text="2.5" />
</LinearLayout>
dividing line divider
Set the divider and showDividers properties.
<pre id="__code_8" style="box-sizing: inherit; color: var(--md-code-fg-color); font-feature-settings: "kern"; font-family: "Roboto Mono", SFMono-Regular, Consolas, Menlo, monospace; direction: ltr; position: relative; margin: 1em 0px; line-height: 1.4;"> `android:divider="@drawable/divider_linear_1"
android:showDividers="middle"
Setting the color directly to the divider is invalid.
Create a new xml in the drawable directory called divider_linear_1.xml
.
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<solid android:color="#7986CB" />
<size
android:width="1dp"
android:height="1dp" />
</shape>
Size must be specified, otherwise it will not be displayed when used as a divider.
LinearLayout sets the divider.
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#eaeaea"
android:divider="@drawable/divider_linear_1"
android:orientation="vertical"
android:showDividers="middle">
....
<LinearLayout
android:layout_width="match_parent"
android:layout_height="100dp"
android:layout_marginTop="20dp"
android:background="#FFD9D9"
android:divider="@drawable/divider_linear_1"
android:orientation="horizontal"
android:showDividers="middle">
...
showDividers has several options:
- middle dividing line in the middle
- beginning dividing line
- end the dividing line at the end
- none no dividing line
Share one last time
[Produced by Tencent Technical Team] Getting started with Android from scratch to mastering it, Android Studio installation tutorial + full set of Android basic tutorials
Android programming introductory tutorial
Java language basics from entry to familiarity
Kotlin language basics from entry to familiarity
Android technology stack from entry to familiarity
Comprehensive learning on Android Jetpack
For novices, it may be difficult to install Android Studio. You can watch the following video to learn how to install and run it step by step.
Android Studio installation tutorial
With the Java stage of learning, it is recommended to focus on video learning at this stage and supplement it with book checking and filling in gaps. If you mainly focus on books, you can type the code based on the book's explanations, supplemented by teaching videos to check for omissions and fill in the gaps. If you encounter problems, you can go to Baidu. Generally, many people will encounter entry-level problems and give better answers.
You need to master basic knowledge points, such as how to use the four major components, how to create Service, how to layout, simple custom View, animation, network communication and other common technologies.
A complete set of zero-based tutorials has been prepared for you. If you need it, you can add the QR code below to get it for free.
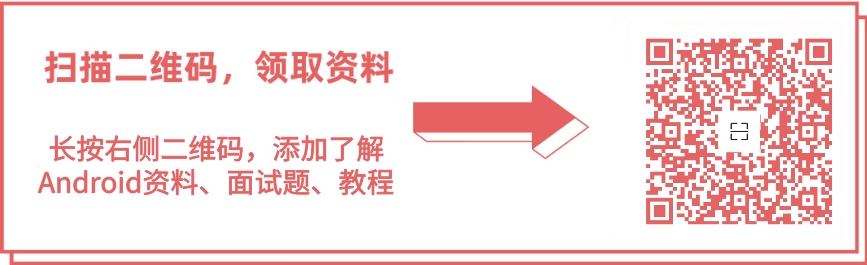
A complete set of basic Android tutorials