3.1 Linear layout - LinearLayout
A LinearLayout is a view container used to keep all subviews aligned in a single direction ( vertical or horizontal ). you may
Use the android:orientation attribute to specify the layout orientation.
- android:orientation="vertical" vertical arrangement
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ffc"
android:orientation="vertical">
<TextView
android:layout_width="200dp"
android:layout_height="90dp"
android:background="#DD001B"
android:gravity="center"
android:text="1"
android:textColor="#ffffff"
android:textSize="25sp"
/>
<TextView
android:layout_width="100dp"
android:layout_height="90dp"
android:background="#000000"
android:gravity="center"
android:text="2"
android:textColor="#ffffff"
android:textSize="25sp"
/>
<TextView
android:layout_width="80dp"
android:layout_height="90dp"
android:background="#139948"
android:gravity="center"
android:text="3"
android:textColor="#ffffff"
android:textSize="25sp"
/>
</LinearLayout>
- android:orientation="horizontal" horizontal arrangement
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ffc"
android:orientation="horizontal">
<TextView
android:layout_width="200dp"
android:layout_height="90dp"
android:background="#DD001B"
android:gravity="center"
android:text="1"
android:textColor="#ffffff"
android:textSize="25sp"
/>
<TextView
android:layout_width="100dp"
android:layout_height="90dp"
android:background="#000000"
android:gravity="center"
android:text="2"
android:textColor="#ffffff"
android:textSize="25sp"
/>
<TextView
android:layout_width="80dp"
android:layout_height="90dp"
android:background="#139948"
android:gravity="center"
android:text="3"
android:textColor="#ffffff"
android:textSize="25sp"
/>
</LinearLayout>
- Layout weight android:layout_weight
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ffc"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:background="#DD001B"
/>
<TextView
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:background="#000000"
/>
<TextView
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:background="#139948"
/>
</LinearLayout>
3.2 Relative Layout - RelativeLayout
- Relative layout: The child view can set the relative position relative to another sibling view or parent view container through the corresponding layout attribute Description of the attribute:
- relative to sibling elements
attribute name |
attribute meaning |
android:layout_below="@id/aaa" |
Below the specified View |
android:layout_above="@id/aaa" |
Above the specified View |
android:layout_toLeftOf="@id/aaa" |
On the left side of the specified View |
android:layout_toRightOf="@id/aaa" |
On the right side of the specified View |
android:layout_alignTop="@id/aaa" |
Consistent with the upper boundary of the specified View |
android:layout_alignBottom="@id/aaa" |
Consistent with the lower boundary of the specified View |
android:layout_alignLeft="@id/aaa" |
与指定View的左边界一致 |
android:layout_alignRight="@id/aaa" |
与指定View的右边界一致 |
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ffc">
<TextView
android:id="@+id/one"
android:layout_width="120dp"
android:layout_height="120dp"
android:background="#DD001B"
android:gravity="center"
android:text="1"
android:textColor="#ffffff"
android:textSize="40sp"
/>
<TextView
android:layout_toRightOf="@id/one"
android:id="@+id/two"
android:layout_width="120dp"
android:layout_height="120dp"
android:background="#000000"
android:gravity="center"
android:text="2"
android:textColor="#ffffff"
android:textSize="40sp"
/>
<TextView
android:layout_below="@id/two"
android:id="@+id/three"
android:layout_width="120dp"
android:layout_height="120dp"
android:background="#139948"
android:gravity="center"
android:text="3"
android:textColor="#ffffff"
android:textSize="40sp"
/>
</RelativeLayout>
-
- 相对于父元素
属性名称 |
属性含义 |
android:layout_alignParentLeft="true" |
在父元素内左边 |
android:layout_alignParentRight="true" |
在父元素内右边 |
android:layout_alignParentTop="true" |
在父元素内顶部 |
android:layout_alignParentBottom="true" |
在父元素内底部 |
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ffc">
<TextView
android:layout_alignParentRight="true"
android:id="@+id/one"
android:layout_width="120dp"
android:layout_height="120dp"
android:background="#DD001B"
android:gravity="center"
android:text="1"
android:textColor="#ffffff"
android:textSize="40sp"
/>
</RelativeLayout>
-
- 对齐方式
属性名称 |
属性含义 |
android:layout_centerInParent="true" |
居中布局 |
android:layout_centerVertical="true" |
垂直居中布局 |
android:layout_centerHorizontal="true" |
水平居中布局 |
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ffc">
<TextView
android:layout_centerVertical="true"
android:id="@+id/one"
android:layout_width="120dp"
android:layout_height="120dp"
android:background="#DD001B"
android:gravity="center"
android:text="1"
android:textColor="#ffffff"
android:textSize="40sp"
/>
</RelativeLayout>
-
- 间隔
属性名称 |
属性含义 |
android:layout_marginBottom="" |
离某元素底边缘的距离 |
android:layout_marginLeft="" |
离某元素左边缘的距离 |
android:layout_marginRight ="" |
离某元素右边缘的距离 |
android:layout_marginTop="" |
离某元素上边缘的距离 |
android:layout_paddingBottom="" |
往内部元素底边缘填充距离 |
android:layout_paddingLeft="" |
往内部元素左边缘填充距离 |
android:layout_paddingRight ="" |
往内部元素右边缘填充距离 |
android:layout_paddingTop="" |
往内部元素右边缘填充距离 |
- 父容器定位属性示意图
- 根据兄弟组件定位
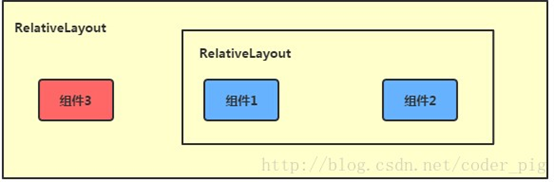
3.3 帧布局 - FrameLayout
最简单的一种布局,没有任何定位方式,当我们往里面添加控件的时候,会默认把他们放到这块区 域的左上角,帧布局的大小由控件中最大的子控件决定,如果控件的大小一样大的话,那么同一时 刻就只能看到最上面的那个组件,后续添加的控件会覆盖前一个。
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ffc">
<View
android:layout_width="400dp"
android:layout_height="400dp"
android:background="#DD001B"
/>
<View
android:layout_width="300dp"
android:layout_height="300dp"
android:background="#000000"
/>
<TextView
android:layout_width="120dp"
android:layout_height="120dp"
android:background="#139948"
/>
</FrameLayout>
3.4 网格布局 GridLayout
属性说明:
名称 |
含义 |
android:columnCount |
列数 |
android:rowCount |
行数 |
android:layout_columnSpan |
横跨的列数 |
android:layout_rowSpan |
横跨的行数 |
<?xml version="1.0" encoding="utf-8"?>
<GridLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/Gridlayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:columnCount="4" //列数
android:orientation="horizontal"
android:rowCount="6" //行数>
<TextView
android:layout_columnSpan="4" //横跨4列
android:layout_gravity="fill" //这一行填充满4列
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
android:background="#ffc"
android:text="0"
android:textSize="50sp"
/>
<Button
android:layout_columnSpan="2" //横跨2列
android:layout_gravity="fill" //这一行填充满2列
android:text="回退"/>
<Button
android:layout_columnSpan="2" //横跨2列
android:layout_gravity="fill" //这一行填充满2列
android:text="清空"/>
<Button android:text="+"/>
<Button android:text="1"/>
<Button android:text="2"/>
<Button android:text="3"/>
<Button android:text="-"/>
<Button android:text="4"/>
<Button android:text="5"/>
<Button android:text="6"/>
<Button android:text="*"/>
<Button android:text="7"/>
<Button android:text="8"/>
<Button android:text="9"/>
<Button android:text="/"/>
<Button android:text="."/>
<Button android:text="0"/>
<Button android:text="="/>
</GridLayout>
计算器模型(完整版)
<?xml version="1.0" encoding="utf-8"?>
<GridLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/Gridlayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:columnCount="4"
android:orientation="horizontal"
android:rowCount="6">
<TextView
android:layout_columnSpan="4"
android:layout_gravity="fill"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
android:background="#ffc"
android:text="0"
android:textSize="50sp" />
<Button
android:layout_columnSpan="2"
android:layout_gravity="fill"
android:text="回退"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button
android:layout_columnSpan="2"
android:layout_gravity="fill"
android:text="清空"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="+"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="1"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="2"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="3"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="-"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="4"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="5"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="6"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="*"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="7"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="8"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="9"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="/"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="."
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="0"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
<Button android:text="="
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"/>
</GridLayout>