
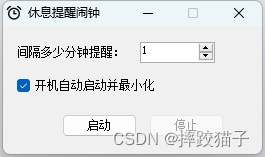
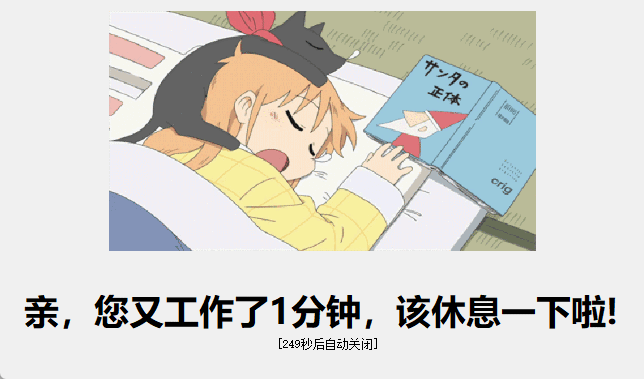
Implementation process
1.1. Create a project
- Open Visual Studio and select Create New Project on the right.
- Enter winform in the search box, select the windows form application, fill in the corresponding save path and click Next. After the creation is successful, as shown in the figure below, there will be a Form form opened by default.
1.2. Time interval configuration page
- Prepare the alarm clock image material, modify the window icon and title style.

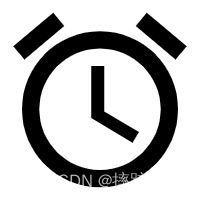
- Configure by setting the properties of the form Text and Icon, and configure the StartPosition property value as CenterScreen, so that the form is displayed in the center by default.
- Drag and drop Label, Button, NumericUpDown, timer and other controls in the toolbox on the left to realize the general layout of the page.
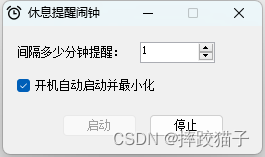
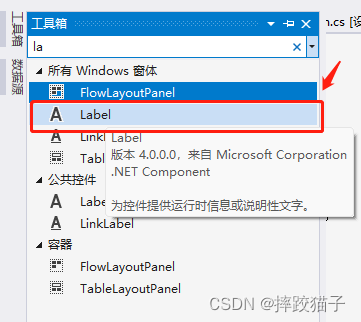
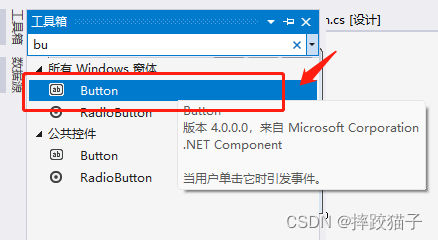
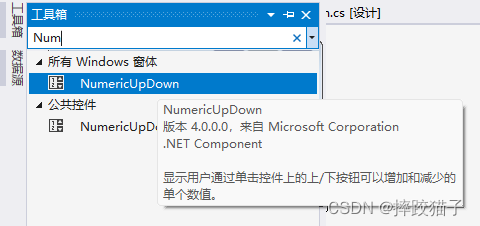
- Double-click the form to enter the code interface and define a counter field at the top.
private int MinCounter = 0;
- Double-click the start button on the form editing interface to generate the corresponding click event code.
- Add the business logic code in the start button click event as follows: restore the counter to 0, disable the start button, release the stop button, and start the timer.
/// <summary>
/// 启动按钮点击事件
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void btnStart_Click(object sender, EventArgs e)
{
MinCounter = 0;
btnStart.Enabled = false;
btnEnd.Enabled = true;
timer1.Enabled = true;
}
- Click the timer control on the form edit page, double-click Tick, and enter the corresponding code. (Tick refers to an event that occurs whenever a specified time interval passes)
private void timer1_Tick(object sender, EventArgs e)
{
}
- In the event, first get the value of the form NumericUupDowm control, which is the number of minutes entered by the user.
int min = tbMin.Value;
- Business code: If the counter is equal to the number of minutes entered by the user, jump to the prompt form and disable the timer control, otherwise the value of the counter is +1.
private void timer1_Tick(object sender, EventArgs e)
{
if (MinCounter == tbMin.Value)
{
timer1.Enabled = false;
using (message msg = new message(tbMin.Value.ToString()))
{
tip.ShowDialog();
}
MinCounter = 0;
timer1.Enabled = true;
}
else
{
MinCounter++;
}
}
1.3. Alarm reminder page
- Select the project to create a new form, customize the name, modify the form title and icon properties according to the above steps; then drag three labels from the toolbox to the form, which are used for displaying pictures, prompt text, countdown, etc.
- Prepare a material map and import it through the image property of one of the label controls, and then modify the value of the AutoSize property to False.
- Define several fields in the prompt form, which are used to control the countdown of the form closing, display the working time and so on.
private static int CLOSE_SEC = 300;
private string STR_MIN = "";
private int RUN_SEC = 0;
- Receive the passed parameters in the form event and display them.
public TipForm(string _min)
{
STR_MIN = _min;
InitializeComponent();
}
- In the form loading event, set the Text property of label2 as prompt information.
label2.Text = "亲,您又工作了"+ STR_MIN + "分钟,该休息一下啦!";
- Drag a timer control from the toolbox to the form, and set the countdown seconds display and form closing event through its Tick property.
private void timer1_Tick(object sender, EventArgs e)
{
RUN_SEC++;
if (RUN_SEC >= CLOSE_SEC)
{
RUN_SEC = 0;
Close();
} else
{
label2.Text = "[" + (CLOSE_SEC - RUN_SEC) + "秒后自动关闭]";
}
}
- It can be further optimized, and when the form is loaded, the ringtone will be played through the Player to synchronize the reminder.
//播放声音
string wavFile = Application.StartupPath + @"\alarm.wav";
Stream sm = null;
if (File.Exists(wavFile))
{
sm = new FileStream(wavFile, FileMode.Open);
} else
{
sm = Properties.Resources.Alarm02;
}
SoundPlayer player = new SoundPlayer(sm);
player.Play();
player.Dispose();
1.4, boot self-start configuration
- Back to the Form1 form, drag a checkBox control in the toolbox to check whether to enable self-starting.
- Implement a function to set the application to run automatically when it starts up.
/// <summary>
/// 设置应用程序开机自动运行
/// </summary>
/// <param name="fileName">应用程序的文件名</param>
/// <param name="isAutoRun">是否自动运行,为false时,取消自动运行</param>
/// <returns>返回1成功,非1不成功</returns>
public static String SetAutoRunByReg(string fileName, bool isAutoRun)
{
string reSet = string.Empty;
RegistryKey reg = null;
try
{
if (!File.Exists(fileName))
{
reSet = "设置/取消自动启动发生异常:" + fileName + "文件不存在!";
}
string key = @"Software\Microsoft\Windows\CurrentVersion\Run";
string name = Path.GetFileName(fileName);
reg = Registry.LocalMachine.OpenSubKey(key, true);
if (reg == null)
{
reg = Registry.LocalMachine.CreateSubKey(key);
}
if (isAutoRun)
{
reg.SetValue(name, fileName);
reSet = "1";
}
else
{
if (reg.GetValue(name) != null)
{
reg.DeleteValue(name);
}
reSet = "1";
}
}
catch (Exception ex)
{
reSet = "设置/取消自动启动发生异常:[" + ex.Message + "],请尝试用管理员身份运行!";
}
finally
{
if (reg != null)
{
reg.Close();
}
}
return reSet;
}
- Double-click the click event of the checkbox to generate the corresponding event code, and call the above function to achieve the effect of booting automatically.
//开机自动启动并最小化
private void chkBoxBoot_Click(object sender, EventArgs e)
{
string res = SetAutoRunByReg(Application.ExecutablePath, chkBoxBoot.Checked);
if (res != "1")
{
MessageBox.Show(res);
}
}
1.5. Logging
- Define a function in the Form1 form to record the startup and operation logs and store them in text.
/// <summary>
/// 写日志
/// </summary>
/// <param name="msg">日志文本</param>
/// <param name="add_datetime">是否添加时间戳</param>
public static void writeLog(string msg, bool add_datetime)
{
string logfile = Application.StartupPath + "/log.txt";
using (StreamWriter w = File.AppendText(logfile))
{
if (add_datetime)
{
w.WriteLine("{0}\t {1}", DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss", DateTimeFormatInfo.InvariantInfo), msg);
}
else
{
w.WriteLine("{0}", msg);
}
w.Flush();
w.Close();
}
}
- Call the above function in the form event.
- Check the log in the project folder -> bin folder -> Debug folder.
1.6. Minimize prompt
- Configure the properties of Form1 such as Closing and Resize to realize the windows prompt effect.
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
//注意判断关闭事件Reason来源于窗体按钮,否则用菜单退出时无法退出!
if (e.CloseReason == CloseReason.UserClosing)
{
e.Cancel = true; //取消"关闭窗口"事件
this.WindowState = FormWindowState.Minimized; //使关闭时窗口向右下角缩小的效果
notifyIcon1.Visible = true;
notifyIcon1.ShowBalloonTip(3000, "提示", "程序未退出,它在这里!", ToolTipIcon.Info);
this.Hide();
return;
}
}
private void Form1_Resize(object sender, EventArgs e)
{
if (this.WindowState == FormWindowState.Minimized) //最小化到系统托盘
{
notifyIcon1.Visible = true; //显示托盘图标
notifyIcon1.ShowBalloonTip(3000, "提示", "程序未退出,它在这里!", ToolTipIcon.Info);
this.Hide(); //隐藏窗口
}
}
Tomorrow is New Year's Eve. In this day of saying goodbye to the old and welcoming the new, I wish you all the best and a bright future.