2. Add Two Numbers
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Example
Input: (2 -> 4 -> 3) + (5 -> 6 -> 4) Output: 7 -> 0 -> 8 Explanation: 342 + 465 = 807.
输入和输出的数据结构统一为 : (个)一>(十)一>(百)一>(千)……( reverse order)
这给数据处理带来了方便,只需从头开始访问输入节点,每个节点的数据相加后存入输出节点,注意两个节点的和可能大于9,需要进位,存入到下一个节点。
struct ListNode { int val; ListNode *next; ListNode(int x):val(x),next(nullptr){} }; //手写了一遍,发现个别语法错误,仔细琢磨,修正之后,打开LeetCode白板一顿写!提交,失败... //发现几处拼写错误。。语法错误。。return用的->,看来还要多实践,很多坑隐藏在实际的编程细节中,单单有思路是不够的 //把经典的基础题慢慢吃透很重要,一步一个脚印慢慢巩固 class Solution_2_1 { public: ListNode *addTwoNumbers(ListNode *l1, ListNode *l2) { //注意:创建的是对象,不是指针,return语句中用.next访问下一个节点 //也可以这样: //ListNode *dummy = new ListNode(-1);//return结果就可以这样写了 dummy->next; //ListNode *prev = dummy; ListNode dummy(-1); int carry = 0;//记录是否有进位 ListNode *prev = &dummy; //因为是反过来存进去链表的,第一个节点是个位,第二个节点存十位 //所以从头开始遍历即可,结果也是反序存入 for (ListNode *pa = l1, *pb = l2; pa != nullptr || pb != nullptr; pa = pa == nullptr ? nullptr : pa->next, pb = pb == nullptr ? nullptr : pb->next, prev = prev->next) { const int ai = pa == nullptr ? 0 : pa->val; const int bi = pb == nullptr ? 0 : pb->val; const int value = (ai + bi + carry) % 10; carry = (ai + bi + carry) / 10;//和大于10,进位,累积到下一个循环 prev->next = new ListNode(value); } if (carry > 0) prev->next = new ListNode(carry); return dummy.next; } };
67. Add Binary
Given two binary strings, return their sum (also a binary string).
扫描二维码关注公众号,回复:
999353 查看本文章
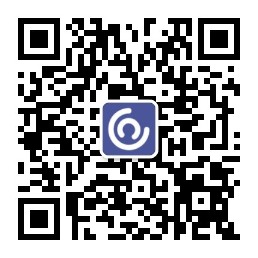
The input strings are both non-empty and contains only characters 1
or 0
.
Example 1:
Input: a = "11", b = "1" Output: "100"
Example 2:
Input: a = "1010", b = "1011" Output: "10101"
参考上一题的思路,先反转字符串,从低到高遍历,结果插入到result前面。
注意string中每个元素到int的转换,即char到int的转换。
之前做的关于一个字符操作的小题:字母轮换
class Solution { public: string addBinary(string a, string b) { string result; const size_t n = a.size() > b.size() ? a.size() : b.size(); reverse(a.begin(), a.end()); reverse(b.begin(), b.end()); int carry = 0; //注意字符和整型的转换,加减'0'即可 for (int i = 0; i != n; ++i) { const int ai = i < a.size() ? a[i]-'0':0; const int bi = i < b.size() ? b[i]-'0':0; const int val = (ai + bi + carry) % 2; carry= (ai + bi + carry)/2; result.insert(result.begin(), val+'0'); } if(carry==1) result.insert(result.begin(), '1'); return result; } };