这篇主要为闭包练习题及答案合集
题目来源:https://github.com/CodesmithLLC/cs-bin-solutions
1. Challenge 1
Create a function createFunction that creates and returns a function. When that created function is called, it should print “hello”.
const function1 = createFunction();
// now we'll call the function we just created
function1(); //should console.log('hello');
Answer:
function createFunction() {
const innerFunc = () => {
console.log('hello');
}
return innerFunc;
}
// UNCOMMENT THESE TO TEST YOUR WORK!
const function1 = createFunction();
function1();
2. Challenge 2
Create a function createFunctionPrinter that accepts one input and returns a function. When that created function is called, it should print out the input that was used when the function was created.
function createFunctionPrinter(input) {
}
// UNCOMMENT THESE TO TEST YOUR WORK!
// const printSample = createFunctionPrinter('sample');
// printSample();
// const printHello = createFunctionPrinter('hello');
// printHello();
Answer
function createFunctionPrinter(input) {
const inner =()=>{
console.log(input)
}
return inner;
}
3. Challenge 3
Examine the code for the outer function. Notice that we are returning a function and that function is using variables that are outside of its scope.
Uncomment those lines of code. Try to deduce the output before executing.
function outer() {
let counter = 0; // this variable is outside incrementCounter's scope
function incrementCounter () {
counter ++;
console.log('counter', counter);
}
return incrementCounter;
}
const willCounter = outer();
const jasCounter = outer();
// Uncomment each of these lines one by one.
// Before your do, guess what will be logged from each function call.
// willCounter();
// willCounter();
// willCounter();
// jasCounter();
// willCounter();
Answer:
// willCounter(); // Logs 'counter 1'
// willCounter(); // Logs 'counter 2'
// willCounter(); // Logs 'counter 3'
// jasCounter(); // Logs 'counter 1'
// willCounter(); // Logs 'counter 4'
Challenge 4
Now we are going to create a function addByX that returns a function that will add an input by x.
const addByTwo = addByX(2);
addByTwo(1); //should return 3
addByTwo(2); //should return 4
addByTwo(3); //should return 5
const addByThree = addByX(3);
addByThree(1); //should return 4
addByThree(2); //should return 5
const addByFour = addByX(4);
addByFour(4); //should return 8
addByFour(10); //should return 14
Question:
function addByX(x) {
}
const addByTwo = addByX(2);
// now call addByTwo with an input of 1
// now call addByTwo with an input of 2
Answer
function addByX(x) {
//const inner = y=>{ //两种写法都可以
function inner(y){
let result = x+y;
console.log(result);
}
return inner;
}
const addByTwo = addByX(2);
addByTwo(9)
const addByFour = addByX(4);
addByFour(10); //should return 8
Challenge 5
Write a function once that accepts a callback as input and returns a function. When the returned function is called the first time, it should call the callback and return that output. If it is called any additional times, instead of calling the callback again it will simply return the output value from the first time it was called.
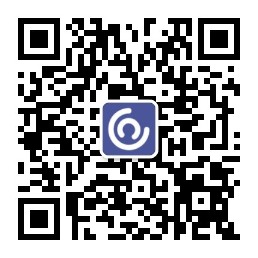
function once(func) {
}
const onceFunc = once(addByTwo);
// UNCOMMENT THESE TO TEST YOUR WORK!
// console.log(onceFunc(4)); //should log 6
// console.log(onceFunc(10)); //should log 6
// console.log(onceFunc(9001)); //should log 6
Answer:
function once(func) {
let counter = 0;
let countVal;
const innerFunc = val =>{
if(counter ===0){
countVal = func(val);
counter ++;
}
return countVal;
}
return innerFunc;
}
const onceFunc = once(addByTwo);
// UNCOMMENT THESE TO TEST YOUR WORK!
console.log(onceFunc(4)); //should log 6
console.log(onceFunc(10)); //should log 6
console.log(onceFunc(9001)); //should log 6
Challenge 6
Write a function after that takes the number of times the callback needs to be called before being executed as the first parameter and the callback as the second parameter.
function after(count, func) {
}
const called = function() { console.log('hello') };
const afterCalled = after(3, called);
// afterCalled(); // -> nothing is printed
// afterCalled(); // -> nothing is printed
// afterCalled(); // -> 'hello' is printed
Answer:
function after(count, func) {
let initialCounter =0;
let countVal;
const innerFunc = (val)=>{
if(initialCounter != count){
console.log("nothing is printed");
initialCounter++;
}
if (initialCounter === count){
countVal = func(val);
}
return countVal;
}
return innerFunc;
}
const called = function() { console.log('hello') };
const afterCalled = after(3, called);
afterCalled(); // -> nothing is printed
afterCalled(); // -> nothing is printed
afterCalled(); // -> 'hello' is printed
Alternatives:
function after(count, func) {
let counter = 0;
return (val) => {
if (++counter >= count) func(val);
}
}
const called = function() { console.log('hello') };
const afterCalled = after(3, called);
Challenge 7
Write a function rollCall that accepts an array of names and returns a function. The first time the returned function is invoked, it should log the first name to the console. The second time it is invoked, it should log the second name to the console, and so on, until all names have been called. Once all names have been called, it should log ‘Everyone accounted for’.
function rollCall(names) {
}
// UNCOMMENT THESE TO TEST YOUR WORK!
// const rollCaller = rollCall(['Victoria', 'Juan', 'Ruth'])
// rollCaller() // -> Should log 'Victoria'
// rollCaller() // -> Should log 'Juan'
// rollCaller() // -> Should log 'Ruth'
// rollCaller() // -> Should log 'Everyone accounted for'
Answer:
function rollCall(names) {
return () => {
if (!names.length) return console.log('Everyone accounted for');
console.log(names.shift());
}
}
Question Reference: https://github.com/CodesmithLLC/cs-bin-solutions