什么是SignalR 的 中心
SignalR hubs API 使你能够从服务端调用已连接的客户端的方法。在服务端代码,你可以定义被客户端调用的方法。而在客户端,你可以定义从服务端调用的方法。SignalR负责使得实时服务端到客户端以及客户端到服务端的通信成为可能的场景之后的所有事情。
配置SignalR 中心
SignalR 中间件需要一些服务,其通过调用 services.AddSignalR 来进行配置。
services.AddSignalR();
当为ASP.NET Core app 添加了 SignalR 功能时,通过调用endpoint.MapHub 来设置SignalR路由,其位于
Startup.Configure 方法中的app.UseEndpoints 回调中。
app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapHub<ChatHub>("/chathub"); });
创建以及使用 中心(hubs)
通过声明一个继承自 Hub 的类来声明一个中心,并为这个新建的类添加公共的方法。客户端可以调用被定义为public 的方法。
public class ChatHub : Hub { public Task SendMessage(string user, string message) { return Clients.All.SendAsync("ReceiveMessage", user, message); } }
你可以指定返回类型以及参数,包括复杂的类型以及数组,如同你在任何C#方法中所做的那样。SignalR会处理在你的参数以及返回值中的复杂对象的序列化以及反序列化的。
注意:中心(hubs)是瞬变的(transient) 。
- 不要在 中心 类的属性中存储状态。每一个 中心 方法的调用都是在一个新的 中心 实例上进行的。
- 当调用依赖于 中心 保存活动的异步方法时,请使用await。举个例子,形如这样的方法调用
Clients.All.SendAsync(...)
如果其在没有await 的情况下被调用,那么其会失败,这是因为 中心 方法 会在 SendAsync 结束之前完成。
上下文对象
中心 类包含一个上下文对象,其包含了下列可以提供连接信息的一些属性:
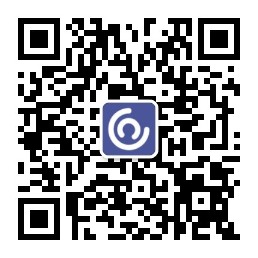
Property | Description |
---|---|
ConnectionId |
Gets the unique ID for the connection, assigned by SignalR. There is one connection ID for each connection. |
UserIdentifier |
Gets the user identifier. By default, SignalR uses the ClaimTypes.NameIdentifier from the ClaimsPrincipal associated with the connection as the user identifier. |
User |
Gets the ClaimsPrincipal associated with the current user. |
Items |
Gets a key/value collection that can be used to share data within the scope of this connection. Data can be stored in this collection and it will persist for the connection across different hub method invocations. |
Features |
Gets the collection of features available on the connection. For now, this collection isn't needed in most scenarios, so it isn't documented in detail yet. |
ConnectionAborted |
Gets a CancellationToken that notifies when the connection is aborted. |
Hub.Context 也包含了如下的方法:
方法 | 描述 |
---|---|
GetHttpContext |
返回对于此连接的HttpContext对象,如果这个连接没有关联一个HTTP 请求,那么便会返回 null。对于HTTP 连接,你可以使用此方法来获取例如HTTP请求头以及查询字符串之类的信息。 |
Abort |
关于这个连接 |
客户端对象
Hub 类 具有一个 Clients 对象,其为了服务端与客户端之前的通信包含了如下属性:
属性 | 描述 |
---|---|
All |
在所有已连接的客户端上调用一个方法 |
Caller |
在调用中心方法的客户端上调用一个方法 |
Others |
在除过调用中心 方法的那个客户端之外的所有客户端上调用一个方法 |
Hub.Clients 同样也包含了如下方法:
Method | Description |
---|---|
AllExcept |
Calls a method on all connected clients except for the specified connections |
Client |
Calls a method on a specific connected client |
Clients |
Calls a method on specific connected clients |
Group |
Calls a method on all connections in the specified group |
GroupExcept |
Calls a method on all connections in the specified group, except the specified connections |
Groups |
Calls a method on multiple groups of connections |
OthersInGroup |
Calls a method on a group of connections, excluding the client that invoked the hub method |
User |
Calls a method on all connections associated with a specific user |
Users |
Calls a method on all connections associated with the specified users |
上表中的每一个属性都会返回一个带有SendAsync方法的对象。SendAsync
方法允许你提供客户端方法的名称和参数用以调用。