SPI(Service Provider Interface)是JDK内置的一种服务提供发现机制。
在平时开发中我们其实已经接触到,只不过一般开发真的用不上,像jdbc、apache logging等都用到SPI机制
这些SPI的接口是由Java核心库来提供,而SPI的实现则是作为Java应用所依赖的jar包被包含进类路径(CLASSPATH)中。例如:JDBC的实现mysql就是通过maven被依赖进来。
那么问题来了,SPI的接口是Java核心库的一部分,是由引导类加载器(Bootstrap Classloader)来加载的。SPI的实现类是由系统类加载器(System ClassLoader)来加载的。
引导类加载器在加载时是无法找到SPI的实现类的,因为双亲委派模型中规定,引导类加载器BootstrapClassloader无法委派系统类加载器AppClassLoader来加载。这时候,该如何解决此问题?
线程上下文类加载由此诞生,它的出现也破坏了类加载器的双亲委派模型,使得程序可以进行逆向类加载。
从JDBC案例分析:
Connection conn = java.sql.DriverManager.getConnection(url, "name", "password");
平时按照上述获取数据库连接,接着看DriverManager类
static {
loadInitialDrivers();
println("JDBC DriverManager initialized");
}
在DriverManager类中有一个静态代码块。很明显会调用loadInitialDrivers,接着loadInitialDrivers方法看
private static void loadInitialDrivers() {
String drivers;
try {
drivers = AccessController.doPrivileged(new PrivilegedAction<String>() {
public String run() {
return System.getProperty("jdbc.drivers");
}
});
} catch (Exception ex) {
drivers = null;
}
// If the driver is packaged as a Service Provider, load it.
// Get all the drivers through the classloader
// exposed as a java.sql.Driver.class service.
// ServiceLoader.load() replaces the sun.misc.Providers()
AccessController.doPrivileged(new PrivilegedAction<Void>() {
public Void run() {
ServiceLoader<Driver> loadedDrivers = ServiceLoader.load(Driver.class);
Iterator<Driver> driversIterator = loadedDrivers.iterator();
/* Load these drivers, so that they can be instantiated.
* It may be the case that the driver class may not be there
* i.e. there may be a packaged driver with the service class
* as implementation of java.sql.Driver but the actual class
* may be missing. In that case a java.util.ServiceConfigurationError
* will be thrown at runtime by the VM trying to locate
* and load the service.
*
* Adding a try catch block to catch those runtime errors
* if driver not available in classpath but it's
* packaged as service and that service is there in classpath.
*/
try{
while(driversIterator.hasNext()) {
driversIterator.next();
}
} catch(Throwable t) {
// Do nothing
}
return null;
}
});
println("DriverManager.initialize: jdbc.drivers = " + drivers);
if (drivers == null || drivers.equals("")) {
return;
}
String[] driversList = drivers.split(":");
println("number of Drivers:" + driversList.length);
for (String aDriver : driversList) {
try {
println("DriverManager.Initialize: loading " + aDriver);
Class.forName(aDriver, true,
ClassLoader.getSystemClassLoader());
} catch (Exception ex) {
println("DriverManager.Initialize: load failed: " + ex);
}
}
}
关键的信息在于ServiceLoader这个工具类,它是jdk提供服务实现查找的一个工具类:java.util.ServiceLoader
。使用SPI机制就会用到它来进行服务实现
上述的Driver是在java.sql.Driver包,由jdk提供规范,它是一个接口。那其他厂商是如何实现?
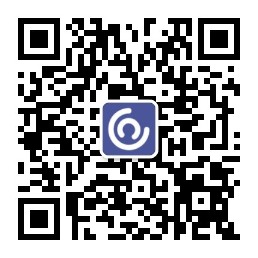
maven引入mysql的依赖会将jar自动加入到类路径/CLASSPATH下
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>6.0.6</version>
</dependency>
而它就提供了SPI机制配置文件(必须是类路径下的META-INF/services路径)
提供的服务实现刚好就是我们引入的mysql包的Driver
文件名需要和接口全限定类名一致java.sql.Driver
com.mysql.cj.jdbc.Driver需要实现java.sql.Driver
public class Driver extends NonRegisteringDriver implements java.sql.Driver {}
线程上下文类加载
在最开始获取数据库连接 的时候,getConnection()方法:
// Worker method called by the public getConnection() methods.
private static Connection getConnection(
String url, java.util.Properties info, Class<?> caller) throws SQLException {
/*
* When callerCl is null, we should check the application's
* (which is invoking this class indirectly)
* classloader, so that the JDBC driver class outside rt.jar
* can be loaded from here.
*/
ClassLoader callerCL = caller != null ? caller.getClassLoader() : null;
synchronized(DriverManager.class) {
// synchronize loading of the correct classloader.
if (callerCL == null) {
callerCL = Thread.currentThread().getContextClassLoader();
}
}
if(url == null) {
throw new SQLException("The url cannot be null", "08001");
}
println("DriverManager.getConnection(\"" + url + "\")");
// Walk through the loaded registeredDrivers attempting to make a connection.
// Remember the first exception that gets raised so we can reraise it.
SQLException reason = null;
for(DriverInfo aDriver : registeredDrivers) {
// If the caller does not have permission to load the driver then
// skip it.
if(isDriverAllowed(aDriver.driver, callerCL)) {
try {
println(" trying " + aDriver.driver.getClass().getName());
Connection con = aDriver.driver.connect(url, info);
if (con != null) {
// Success!
println("getConnection returning " + aDriver.driver.getClass().getName());
return (con);
}
} catch (SQLException ex) {
if (reason == null) {
reason = ex;
}
}
} else {
println(" skipping: " + aDriver.getClass().getName());
}
}
// if we got here nobody could connect.
if (reason != null) {
println("getConnection failed: " + reason);
throw reason;
}
println("getConnection: no suitable driver found for "+ url);
throw new SQLException("No suitable driver found for "+ url, "08001");
}
核心点:Thread.currentThread().getContextClassLoader()
简单实例:
新建ColorInterface接口,2个实现类BlackService、WhiteService分别实现ColorInterface
package com.shentb.hmb.spi;
public interface ColorInterface {
void sayColor();
}
package com.shentb.hmb.spi.impl;
import com.shentb.hmb.spi.ColorInterface;
public class BlackService implements ColorInterface {
@Override
public void sayColor() {
System.err.println("Black");
}
}
package com.shentb.hmb.spi.impl;
import com.shentb.hmb.spi.ColorInterface;
public class WhiteService implements ColorInterface {
@Override
public void sayColor() {
System.err.println("White");
}
}
在项目类路径下新建/META-INF/services目录,目录新建com.shentb.hmb.spi.ColorInterface文件,文件名需要和ColorInterface全限定类名一致
内容为服务实现的全限定类名。