因为前两个月的新冠疫情公司的开工时间都有延迟,在家里待着蹭吃蹭喝逐渐忘记自己还是一个IT从业人员,复工之后才逐渐回神,想起自己还有博客。哈哈哈
最近开发时遇到了关于ref的使用,看了文档之后了解到refs的使用在16.3版本之后已经出现了变更,就此记录一下
16.3之后版本的Ref推荐用法(React.createRef()):
1.简单使用:
// 代码引入了typeScript
// 通过createRef使用 React16.3之后的推荐用法
export class RefCreate extends React.Component{
// 泛型中的HTML类型需要根据对应的ref元素进行更改
private btnRef:React.RefObject<HTMLButtonElement> = React.createRef();
getRef = () => {
console.log(this.btnRef);
}
render(){
return (
<>
<button ref={this.btnRef}>最新的ref用法</button>
<button onClick={this.getRef}>获取Ref</button>
</>
)
}
}
2.父组件需要获取子组件中的Ref
// 代码使用了TypeScript
// 在父组件通过createRef()创建Ref,通过ref传递给子组件,子组件通过forwardRef()方法对ref进行转发,转发至对应的DOM
// 子组件
export const FancyButton =
React.forwardRef<HTMLButtonElement, PropsWithoutRef<ComponentProps<'button'>> & RefAttributes<HTMLButtonElement>>((props, ref) => {
return <button ref={ref}>{props.children}</button>
})
// 父组件
class ParentRef extends React.Component<{},{}>{
// 定义Ref
private childRefs:React.RefObject<HTMLButtonElement> = React.createRef();
getChildRef = () => {
console.log(this.childRefs.current);
}
render(){
return (
<>
<FancyButton btnRef={this.childRefs} />
<button onClick={this.getChildRef}>获取子组件ref</button>
</>
)
}
}
这个里面值得一说的是关于forward转发的泛型,当时看了ts声明没有很明白,百度之后才知道对应泛型的类型(有点长):
<HTMLButtonElement, PropsWithoutRef<ComponentProps<'button'>> & RefAttributes<HTMLButtonElement>>
16.3之前版本的Ref用法:
1.简单用法(字符串Ref)
// 字符串类型的ref 通过this.refs.XX调用 已经不再推荐使用
export default class RefsButton extends React.Component{
constructor(props:any){
super(props);
this.state={
}
}
getMyRefs = () => {
console.log(this.refs.myBtn);
}
render(){
return(
<>
<button ref="myBtn">字符串类型的ref</button>
<button onClick={this.getMyRefs}>获取ref</button>
</>
)
}
}
2.简单用法(回调函数的Ref)
扫描二维码关注公众号,回复:
9763341 查看本文章
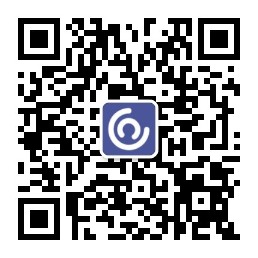
// 通过Ref回调函数的形式使用
export class RefFunction extends React.Component{
btnRef:HTMLButtonElement | null = null;
setRef = (el:HTMLButtonElement) => {
this.btnRef = el;
}
getRef = () => {
console.log(this.btnRef);
}
render(){
return (
<>
<button ref={this.setRef}>通过回调形式设置ref</button>
<button onClick={this.getRef}>获取Ref</button>
</>
)
}
}
3.父组件调用子组件的Ref
// 父组件获取子组件的ref ref通过回调获取 通过props传递
// 子组件
export class PropRef extends React.Component<{btnRef: (el:HTMLButtonElement) => any},{}>{
constructor(props:any){
super(props);
this.state={
}
}
render(){
return(
<>
<button ref={this.props.btnRef}>子按钮</button>
</>
)
}
}
// 父组件
class PropRef extends React.Component<{},{}>{
constructor(props:any){
super(props);
this.state={
}
}
private parentRef: HTMLButtonElement | null = null;
getChildRef = () => {
console.log(this.parentRef);
}
render(){
return(
<>
<PropRef btnRef={el => this.parentRef = el} />
<button onClick={this.getChildRef}>获取子组件ref</button>
</>
)
}
}
暂时整理的ref使用方式大概就这些,希望对各位有所帮助
over