1.什么是命令行参数?
命令行参数是在运行时赋予程序/脚本的标志。它们包含程序的其他信息,以便可以执行。
并非所有程序都具有命令行参数,因为并非所有程序都需要它们。在我的博客文章的Python脚本中广泛使用了命令行参数,甚至可以说,这个博客上98%的文章都使用了命令行参数。
2.为什么我们使用命令行参数?
如前所述,命令行参数在运行时为程序提供了更多信息。
这使我们可以在不更改代码的情况下即时为程序提供不同的输入。
可以类推命令行参数类似于函数参数,如果知道如何在各种编程语言中声明和调用函数,那么当发现如何使用命令行参数时,就会立即感到宾至如归。
鉴于这是计算机视觉和图像处理博客,因此在此处看到的许多参数都是图像路径或视频路径。
在深度学习的情况下,将看到模型路径或时间点计数作为命令行参数。
在本文中,我们将通过两个脚本示例来学习Python argparse包。
3.Python argparse 库
首先,让我们命名一个新脚本 simple_example.py :
# 导入argparse包
import argparse
# 构造参数并解析参数
ap = argparse.ArgumentParser()
ap.add_argument("-n", "--name", required=True,
help="name of the user")
args = vars(ap.parse_args())
# 打印交互信息
print("Hi there {}, it's nice to meet you!".format(args["name"]))
添加唯一的参数, -n或–name,必须同时指定速记(-n)和普通版本(–name),其中任一标志均可在命令行中使用。如以上所述,–name是必需的参数required=True。
–help是可选参数,终端输入:
python simple_example.py --help
打印出以下提示信息:
usage: simple_example.py [-h] -n NAME
optional arguments:
-h, --help show this help message and exit
-n NAME, --name NAME name of the user
输入以下命令运行脚本:
python simple_example.py --name 哲少
打印出以下结果:
Hi there 哲少, it's nice to meet you!
只要名字没有空格,它就会在输出中正确显示。
4.使用Python解析命令行参数
第二个脚本shape_counter.py:
# USAGE
# python shape_counter.py --input input_01.png --output output_01.png
# python shape_counter.py --input input_02.png --output output_02.png
# 导入必要的软件包
import argparse
import imutils
import cv2
# 构造参数并解析参数
ap = argparse.ArgumentParser()
ap.add_argument("-i", "--input", required=True,
help="path to input image")
ap.add_argument("-o", "--output", required=True,
help="path to output image")
args = vars(ap.parse_args())
# 从磁盘加载图像
image = cv2.imread(args["input"])
# 将图像转换为灰度图像、高斯平滑、阈值求取并二值化
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
blurred = cv2.GaussianBlur(gray, (5,5), 0)
thresh = cv2.threshold(blurred, 60, 255, cv2.THRESH_BINARY)[1]
# 从图像中提取轮廓
cnts = cv2.findContours(thresh.copy(), cv2.RETR_EXTERNAL,
cv2.CHAIN_APPROX_SIMPLE)
cnts = imutils.grab_contours(cnts)
# 在输入的图像上循环绘制轮廓
for c in cnts:
cv2.drawContours(image, [c], -1, (0, 0, 255), 2)
# 显示图像中形状的总数
text = "I found {} total shapes".format(len(cnts))
cv2.putText(image, text, (10, 20), cv2.FONT_HERSHEY_SIMPLEX, 0.5,
(0, 0, 255), 2)
# 保存输出图像到硬盘
cv2.imwrite(args["output"], image)
运行脚本:
python shape_counter.py --input input_01.png --output output_01.png
以不同的参数再次运行脚本:
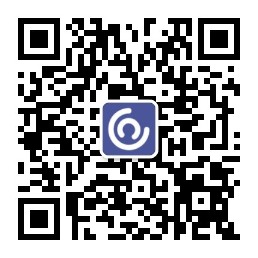
python shape_counter.py --input input_02.png --output output_02.png
最后,要注意一个“陷阱”,有时在此博客上,我的命令行参数标志中带有“-”(破折号),例如–features-db 。抓取参数所包含的值时,您需要使用“ _”(下划线),这有点令人困惑并且有点麻烦,例如以下代码:
# construct the argument parser and parse the arguments
ap = argparse.ArgumentParser()
ap.add_argument("-d", "--dataset", required=True, help="Path to the directory of indexed images")
ap.add_argument("-f", "--features-db", required=True, help="Path to the features database")
ap.add_argument("-c", "--codebook", required=True, help="Path to the codebook")
ap.add_argument("-o", "--output", required=True, help="Path to output directory")
args = vars(ap.parse_args())
# load the codebook and open the features database
vocab = pickle.loads(open(args["codebook"], "rb").read())
featuresDB = h5py.File(args["features_db"], mode="r")
print("[INFO] starting distance computations...")
因为argparse库在解析过程中,Python用下划线替换了破折号。