单链表是逻辑顺序结构,非物理顺序结构,是将存在不同地址的结点链接起来形成的。每个结点中的结构都相同,都是以,元素数据data和指向下一个结点*next的指针构成的
这里给出百度百科中的单链表逻辑结构
单链表的结构
#include <stdio.h>
#include <stdlib.h>
typedef struct node
{
int data;
struct node* next;
}Node;
这里的data,用于存放单链表节点中的数据,next用于指向下一个结点,默认为NULL,一定是指针形式。
单链表初始化
void create(Node** head)
{
*head = (Node*)malloc(sizeof(Node));
*head = NULL;
}
初始化单链表需要先分配一个sizeof(Node)的地址空间,并将内容置为空,构造单链表的头节点
结点头插
void push_front(Node** head, int d)
{
Node* temp = (Node*)malloc(sizeof(Node));
temp->data = d;
temp->next = *head;
*head = temp;
}
头插法每次会新申请结点,并将新结点插入到链表头部,所以头插中结点是倒序的
查找结点
Node* find(Node* head, int data)
{
Node* cur = head;
while (cur->data != data)
{
cur = cur->next;
}
return cur;
}
查找相应内容的结点,就是遍历链表,找到数据后返回结点即可
任意位置插入
void insert(Node** head, Node* pos, int data)
{
Node* cur = *head;
while (cur != pos)
{
cur = cur->next;
}
Node* temp = (Node*)malloc(sizeof(Node));
temp->data = data;
temp->next = cur->next;
cur->next = temp;
}
删除结点
void erase(Node** head, int data)
{
Node* cur = *head;
while (cur->next->data != data)
{
cur = cur->next;
}
Node* temp = cur->next;
cur->next = cur->next->next;
free(temp);
}
删除结点只需要用遍历找到相应结点,与下下个结点链接,就可以将当前结点删除,最后用free释放空间即可,只不过需要记录上一个结点。
链表反转
因为单链表是顺序向下的,每个结点相对独立,所以链表反转相对困难
链表反转详解地址
扫描二维码关注公众号,回复:
9655543 查看本文章
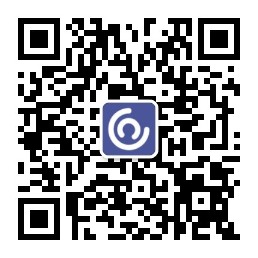
void reverse(Node** head)
{
Node* oldh, *h,*temp;
oldh = *head;
h = *head;
temp = (*head)->next;
while (temp)
{
oldh->next = temp->next;
temp->next = h;
h = temp;
temp = oldh->next;
}
*head = h;
}
源代码获取
完整代码:https://github.com/akh5/C-/blob/master/数据结构/单链表