C++判断时间格式满足yyyy-mm-dd-hh:mm与时间1小于时间2
#include <iostream>
#include <sstream>
using namespace std;
int stringToInt(string str)
{
int result;
istringstream is(str);
is >> result;
return result;
}
bool CheckTime(string time)
{
bool judge = false;
if(time.length()!=16)
return judge;
int i;
string s;
for(i=0;i<4;i++)
s += time[i];
int year = stringToInt(s);
if(year<2017)
return judge;
s = "";
s += time[5];
s += time[6];
int month = stringToInt(s);
if(month<1 || month>12)
return judge;
s = "";
s += time[8];
s += time[9];
int day = stringToInt(s);
if(day<1 || day>31)
return judge;
s = "";
s += time[11];
s += time[12];
int hour = stringToInt(s);
if(hour<0 || hour>=24)
return judge;
s = "";
s += time[14];
s += time[15];
int minute = stringToInt(s);
if(minute<0 || minute>=60)
return judge;
static int dash[3]={4,7,10};
for(i=0;i<3;i++)
if(time[dash[i]]!='-')
return judge;
if(time[13]!=':')
return judge;
judge = true;
return judge;
}
bool CheckTime2(string time1,string time2)
{
bool judge = false;
int i;
string s1,s2;
for(i=0;i<4;i++)
{
s1 += time1[i];
s2 += time2[i];
}
int year1 = stringToInt(s1);
int year2 = stringToInt(s2);
s1 = "";
s2 = "";
s1 += time1[5];
s1 += time1[6];
s2 += time2[5];
s2 += time2[6];
int month1 = stringToInt(s1);
int month2 = stringToInt(s2);
s1 = "";
s2 = "";
s1 += time1[8];
s1 += time1[9];
s2 += time2[8];
s2 += time2[9];
int day1 = stringToInt(s1);
int day2 = stringToInt(s2);
s1 = "";
s2 = "";
s1 += time1[11];
s1 += time1[12];
s2 += time2[11];
s2 += time2[12];
int hour1 = stringToInt(s1);
int hour2 = stringToInt(s2);
s1 = "";
s2 = "";
s1 += time1[14];
s1 += time1[15];
s2 += time2[14];
s2 += time2[15];
int minute1 = stringToInt(s1);
int minute2 = stringToInt(s2);
if(year1<year2)
{
return true;
}
else
{
if(year1==year2)
{
if(month1<month2)
{
return true;
}
else
{
if(month1==month2)
{
if(day1<day2)
{
return true;
}
else
{
if(day1==day2)
{
if(hour1<hour2)
{
return true;
}
else
{
if(hour1==hour2)
{
if(minute1<minute2)
{
return true;
}
else
{
return false;
}
}
else
{
return false;
}
}
}
else
{
return false;
}
}
}
else
{
return false;
}
}
}
else
{
return false;
}
}
return judge;
}
int main()
{
cout << CheckTime("2017-01-28-08:05") << "\t符合yyyy-mm-dd-hh:mm格式" << endl;
cout << CheckTime("2017-01-28-08:000") << "\t不符合字数" << endl;
cout << CheckTime("2000-01-28-08:05") << "\t不符合年>2017" << endl;
cout << CheckTime("2017-00-28-08:05") << "\t不符合01<=月<=12" << endl;
cout << CheckTime("2017-01-00-08:05") << "\t不符合01<=日<=31" << endl;
cout << CheckTime("2017-01-28-99:05") << "\t不符合00<=时<=24" << endl;
cout << CheckTime("2017-01-28-08:99") << "\t不符合00<=分<=60" << endl;
cout << CheckTime("2017-01-28.08:05") << "\t不符合横杆" << endl;
cout << CheckTime("2017-01-28-08.05") << "\t不符合冒号" << endl;
cout << endl << endl << endl;
cout << CheckTime2("2017-01-28-08:05","2017-01-28-08:06") << "\t符合时间1<时间2" << endl;
cout << CheckTime2("2018-01-28-08:05","2017-01-28-11:30") << "\t不符合年1<年2" << endl;
cout << CheckTime2("2017-12-28-08:05","2017-01-28-11:30") << "\t不符合月1<月2" << endl;
cout << CheckTime2("2017-01-31-08:05","2017-01-28-11:30") << "\t不符合日1<日2" << endl;
cout << CheckTime2("2017-01-28-12:05","2017-01-28-11:30") << "\t不符合时1<时2" << endl;
cout << CheckTime2("2017-01-28-11:30","2017-01-28-11:30") << "\t不符合分1<分2" << endl;
return 0;
}
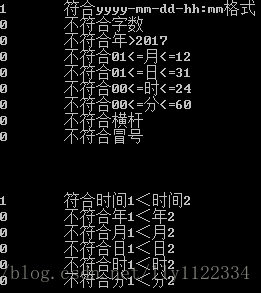