知识点
- resize: none :textarea不允许拉动
- text-indent :规定文本块中首行文本的缩进
- $.trim(str):去掉字符串起始和结尾的空格
- focus([[data],fn]):当元素获得焦点时,触发 focus 事件
- 如果只将点击事件绑定在li标签上,新增的li标签不会带有绑定事件。
解决方案:
① 在新增li标签时候,为其增加绑定事件。但代码较为冗余。
② 事件委托。将事件绑定在父级标签上。
语法:父级标签.on(事件,事件源,触发函数)
例如:$('.dataList>ul').on('click', 'li', function () {
$(this).animate({
'width': '0px'
}, 1000, function () {
$(this).remove();
});
});
代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
* {padding: 0;margin: 0;list-style: none;}
body {background-image: url("images/body_bg.jpg");}
.box {width: 700px;margin:100px auto;}
.input {width: 700px;height: 200px;border: 1px solid #cccccc;background-color: white;border-radius: 6px;}
.input .content {width: 690px;height: 150px;margin: 5px;box-sizing: border-box;border: 1px solid gray;resize: none; outline: none; padding: 10px;}
.input .submit {width: 100px;height: 30px;float: right;background: orangered;color: white;margin-bottom: 5px;margin-right: 5px; border: none; outline: none;cursor: pointer;}
.dataList {width: 600px;margin: 50px auto;}
.dataList>ul>li {width: 100%;height: 40px;line-height: 40px;color: slategray;background: lightblue;margin-bottom: 5px;border-radius: 40px;text-indent: 10px;cursor: pointer;}
</style>
</head>
<body>
<div class="box">
<div class="input">
<textarea class="content"></textarea>
<button class="submit">发布</button>
</div>
<div class="dataList">
<ul>
<li>今天的天气很好呀!</li>
<li>今天的天气很好呀!</li>
<li>今天的天气很好呀!</li>
</ul>
</div>
</div>
<script type="text/javascript" src="lib/jquery-3.3.1.js"></script>
<script type="text/javascript">
$(function () {
var $content = $('.content');
$('.submit').click(function () {
var content = $content.val();
if($.trim(content).length <= 0){
alert('请输入内容!');
$content.val('');
$('.content').focus();
return;
}
var $newLi = $('<li>' + content +'</li>');
var $ul = $('.dataList>ul');
$newLi.prependTo($ul);
$newLi.hide();
$newLi.slideDown(500);
$content.val('');
$content.focus();
});
$('body').on('click', 'li', function () {
$(this).animate({
'width': '0px'
}, 1000, function () {
$(this).remove();
});
});
});
</script>
</body>
</html>
运行结果
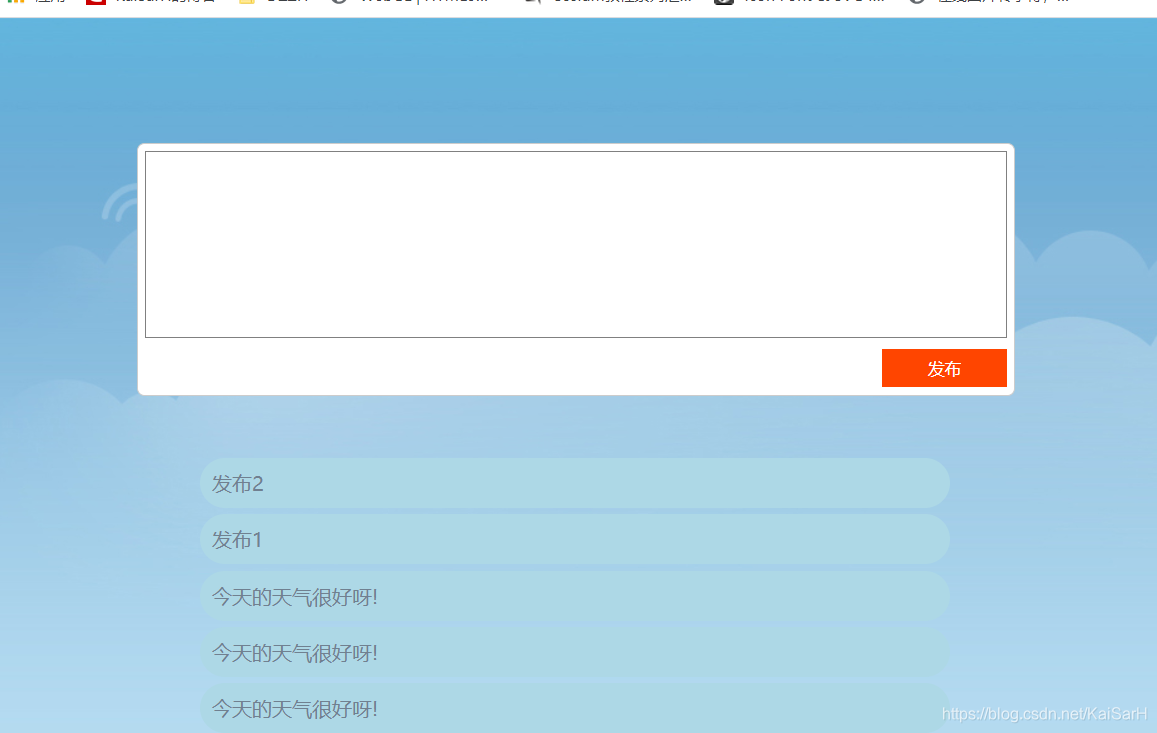