package com.lenovo.www.entity; public class Essay { public static void main(String[] args) { Essay essay = new Essay(); essay.writeEssay(10, "aaa"); essay.writeEssay("派克", "A4纸"); } public void writeEssay() { System.out.println("无参方法"); } public void writeEssay(String pencil,String paper) { System.out.println("用纸和笔写文章"); } public void writeEssay(int a,String b) { System.out.println("用"+a+"纸笔写文章"); } public void writeEssay(String a,int b) { System.out.println("用"+b+"张纸写文章"); } public String writeEssay(String computer) { return computer; } }
面向对象
一.类(抽象概念)
类是具有相同属性和方法的对象的集合
二.对象
用来描述客观事物的一个实体,由一组属性和方法构成
三.面向对象的的优点
可移植性,可维护性,安全性,更符合人类思维方式,易于开发,提高代码可读性。
方法
一.属性:对象具有的特征
二.方法:对象执行的操作,用方法来描述现实事物的行为。
三.如何定义方法
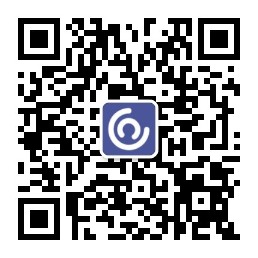
形参列参表:
1.形参列参表用于定义该方法可以接受的参数。
2.形参列参表由零组到多组“参数类型参名”组合而成。
3.多组参数之间以英文(, )逗号隔开,形参类型和形参名之间以英文空格隔开。
4.一旦定义方法时指定了形参列表,则调用该方法时必须传入对应他的参数值—谁调用方法,谁负责为形参赋值
四.定义带参方法的优点:复用性 灵活性
五.方法的重载
1方法的重载只针对于同一类中的方法而言
2.重载关心 参数,有参无参,参数类型不同,参数数量不同,不同类型的参数顺序不同,只要满足这些,就可以实现方法的重载。
3方法重载
1)方法名一样
2)参数列表不一样(参数个数不同或参数类型不同)
eclipse快捷键
1.导包键:CTRL+SHIFT+O
2.代码格式化:CTRL+SHIFT+F
3.重命名:ALT+SHIFT+R
4.ALT+/自动补全或提示代码
构造方法
一.定义
构造方法是一种特殊的方法,主要功能是在创建对象的初始化对象,即为对象或成员变量赋初始值。
二.规则
构造方法与类名相同,可重载多个不同的构造方法。
eg:public car ( ) {
}
三.注意
1构造方法的方法名必须与类名相同。
2.构造方法没有返回类型,也不能定义为void,在方法名前面不声明方法类型。
3.构造方法的主要作用是完成对象的初始化工作
四:构造方法的两个例子;
package com.lenovo.www.day10; /** * 测试类 * * @author lenovo64 *这只小狗是小白,白色正在和那只小猫叫做小黑,黑色在打架 *获胜的动物是黑色 */ public class Test { public static void main(String[] args) { Animal dog = new Animal("小黑", "小猫", "黑色"); Animal cat = new Animal("小白", "小狗", "白色"); String s = dog.play(cat, dog); System.out.println("获胜的动物是" + s); } } class Animal { String name; String kind; int age; String color; long animalID; String date; public Animal(String name, String kind) { this.name = name; this.kind = kind; } public Animal(String name, String color, String kind) { this.name = name; this.color = color; this.kind = kind; } public Animal(String name, int age, long animalID) { this.age = age; this.animalID = animalID; } public String play(Animal dog, Animal cat) { System.out.println("这只" + dog.color + "是" + dog.name + "," + dog.kind + ",正在和那只" + cat.color + "叫做" + cat.name + "," + cat.kind + ",在打架"); return cat.kind; } }
package com.zjm.www.day10; public class Ex_animal { public static void main(String[] args) { // TODO Auto-generated method stub Animal an1 = new Animal("兔子","草",5); Animal an2 = new Animal(); Animal an3 = new Animal(an1,an2); an2.all(an3); } } class Animal{ String name; String eat; int weight; public Animal() { this.name = name; this.eat = "猪蹄"; this.weight = 12; } public Animal(Animal a,Animal b) { this.name = b.name; this.eat = a.eat; this.weight = b.weight; } public Animal(String name,String eat,int weight) { this.name = name; this.eat = eat; this.weight = weight; } public Animal(String name,int weight) { this.name = name; this.weight = weight; } public void eat1() { System.out.println("这个动物喜欢吃" + this.eat); } public void run() { System.out.println("这个动物会跑"); } public void all(Animal an) { System.out.println("这是一只 " + this.name + ",它喜欢吃 " + an.eat + ",它重 " + an.weight +" 斤"); } }***在写代码的过程中需要注意实参和形参的分辨