前言
什么是 MyBatis?
MyBatis 是一款优秀的持久层框架,它支持定制化 SQL、存储过程以及高级映射。MyBatis 避免了几乎所有的 JDBC 代码和手动设置参数以及获取结果集。MyBatis 可以使用简单的 XML 或注解来配置和映射原生类型、接口和 Java 的 POJO(Plain Old Java Objects,普通老式 Java 对象)为数据库中的记录。
准备工作
eclipse,
MySQL
实战
先新建数据
源码:
USE t26shop;
DROP TABLE ShopGood;
DROP TABLE ShopType;
DROP TABLE ShopEmp;
CREATE TABLE ShopEmp
(
empid INT PRIMARY KEY AUTO_INCREMENT
,loginName VARCHAR(20)
,loginPwd VARCHAR(20)
);
CREATE TABLE ShopType
(
typeid INT PRIMARY KEY AUTO_INCREMENT
,typename VARCHAR(20)
);
CREATE TABLE ShopGood
(
goodid INT PRIMARY KEY AUTO_INCREMENT
,goodName VARCHAR(20)
,goodImg VARCHAR(30)
,typeid INT
,gooddate DATE
);
INSERT INTO ShopEmp VALUES(1,'zhangsan','123');
INSERT INTO ShopEmp VALUES(2,'wangwu','123');
INSERT INTO ShopType VALUES(1,'大电器');
INSERT INTO ShopType VALUES(2,'服装');
INSERT INTO ShopType VALUES(3,'食品');
INSERT INTO ShopGood VALUES(2,'海尔冰箱','1.jpg',1,'2013-5-1');
INSERT INTO ShopGood VALUES(1,'LG洗衣机','2.jpg',1,'2013-6-1');
INSERT INTO ShopGood VALUES(3,'青岛啤酒','3.jpg',3,'2013-7-1');
SELECT * FROM ShopEmp;
然后创建一个java web项目
导入jar包,后面会上传整个项目当然也包括jar包。
在src目录下新建两个package包
在src目录下新建一个folder包
我这个包已经创建了的,所有会有报错。
在实体类包新建一个Type实体类
源码:
package com.study.it.entity;
public class Type {
private int id;
private String name;
// 省略get、set
}
新建接口类
源码:
package com.study.it.dao;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import com.study.it.entity.Type;
public interface TypeDao {
public int insertType(@Param("name")String typeName);
public int updateType(int id, String typeName);
public int deleteType(int id);
public int selectCount();
public Type selectById(int id);
public List<Type> selectAll();
public List<Type> selectPage(
@Param("findname")String findname,
@Param("start")int start,
@Param("row")int row,
@Param("orderby")String orderby);
public List<Type> selectByIds(@Param("ids")int[] ids);
}
新建xml,这个xml是存放SQL语句的
源码:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.study.it.dao.TypeDao">
<resultMap type="com.study.it.entity.Type" id="baseMapper">
<id column="typeid" property="id"/>
<result column="typename" property="name"/>
<!-- 一对多 -->
<collection property="goods"
ofType="com.study.it.entity.Good"
column="typeid">
<id column="goodid" property="id"/>
<result column="goodname" property="name"/>
<result column="goodimg" property="img"/>
<result column="gooddate" property="goodDate"/>
</collection>
</resultMap>
<sql id="selectsql">
select t.*,g.* from shoptype t
join shopgood g on t.typeid=g.typeid
</sql>
<insert id="insertType">
insert into shoptype values(default, #{name});
</insert>
<update id="updateType">
update shoptype set typename=#{1} where typeid=#{0};
</update>
<delete id="deleteType">
delete from shoptype where typeid=#{0};
</delete>
<select id="selectCount" resultType="int">
select count(1) from shopType
</select>
<select id="selectById" resultMap="baseMapper">
<include refid="selectsql"/> where typeid=#{0}
</select>
<!-- 查询单个对象偷懒式写法,查询结果和java类的字段名吻合,就不用设置resultMap -->
<!-- <select id="selectById" resultType="com.study.it.entity.Type">
select typeid as id,typename as name from shoptype where typeid=#{0}
</select> -->
<select id="selectAll" resultMap="baseMapper">
<include refid="selectsql"/>
</select>
<!-- #{0} 相当于 ?,${4} 相当于 +。列表不能成为参数 -->
<!-- 这些是属于表的结构,不能用用?号传参,只能用字符串相加来带入 -->
<select id="selectPage" resultMap="baseMapper">
<include refid="selectsql"/>
<if test="findname!=null">
where typename like #{findname}
</if>
order by ${orderby}
limit #{start}, #{row}
</select>
<select id="selectByIds" resultMap="baseMapper">
<include refid="selectsql"/>
<if test="ids!=null">
where typeid in(
<foreach collection="ids" item="id" separator=",">
#{id}
</foreach>
)
</if>
</select>
</mapper>
配置mybatis配置文件,配置数据库驱动、连接字符串、数据库账号和数据库密码
源码:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC" />
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/t26shop?useUnicode=true&characterEncoding=utf-8" />
<property name="username" value="root" />
<property name="password" value="" />
</dataSource>
</environment>
</environments>
<mappers>
<mapper resource="mapper/TypeDaoMapper.xml" />
</mappers>
</configuration>
这些配置完之后就可以编写测试类了,我们这次使用Main方法测试,这次创建的包叫test,我已经创建好了
然后创建一个package包,在包里创建一个带有Main方法的类。
源码:
package test.dao;
import java.io.InputStream;
import java.util.List;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import com.study.it.dao.TypeDao;
import com.study.it.entity.Type;
public class TypeDaoTest {
public static void main(String[] args) {
String resource = "mybatis-config.xml";
// 使用类加载器加载mybatis的配置文件(它也加载关联的映射文件)
InputStream is = TypeDaoTest.class.getClassLoader().getResourceAsStream(resource);
// 构建sqlSession的工厂
SqlSessionFactory sessionFactory = new SqlSessionFactoryBuilder().build(is);
// 创建能执行映射文件中sql的sqlSession
SqlSession session = sessionFactory.openSession();
// 自动帮你产生Dao的实现类(告诉它类名)
TypeDao dao = session.getMapper(TypeDao.class);
// 插入一条类型
// dao.insertType("学习用品");
// 查询行数
// int n = dao.selectCount();
// System.out.println(n);
// 根据id查对象
// Type t = dao.selectById(1);
// System.out.println(t.getName() + "," + t.getId());
// 查询集合
// List<Type> list = dao.selectAll();
// for (Type type : list) {
// System.out.println(type.getName() + "," + type.getId());
// }
// 分页查询
// List<Type> list = dao.selectPage("%%", 0, 3, "typename desc");
// for (Type type : list) {
// System.out.println(type.getName() + "," + type.getId());
// }
// 循环查询
// List<Type> list = dao.selectByIds(new int[]{1,3,4});
// for (Type type : list) {
// System.out.println(type.getName() + "," + type.getId());
// }
session.commit();
session.close();
System.out.println("完毕!");
}
}
过程潦草,多多指教。
本项目还有后半部分类似操作的,但是包含一对多、多对一的操作。
扫描二维码关注公众号,回复:
9470351 查看本文章
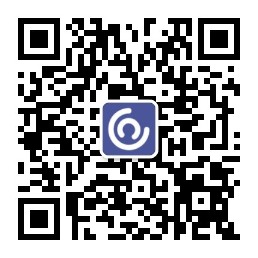