phantomjs截取网页截图
-
场景
有一个视频播放地址,需要对该网页进行截图 -
解决思路:
1.将视频下载到本地,使用ffmpeg进行处理
2.使用phantomjs,phantomjs内置了webkit浏览器引擎,phantomjs可以模拟浏览器打开视频地址,然后进行整个网页的截图。
WebKit 是一个开源的浏览器引擎,与之相对应的引擎有Gecko(Mozilla Firefox 等使用)和Trident(也称MSHTML,IE 使用)
-
选择
第一个方案,ffmpeg只能处理本地视频或者处理RTCP直播流,同时要求的视频直播地址中有部分是直播流,有部分是组件渲染,所以该方案不可行。
因此选择第二个方案。 -
phantomjs进行网页截图,这里以window平台为例
1.首先,去phantomjs官网下载页面下载phantomjs程序,支持window、mac os、linux、freebsd平台。
2.将下载下来的phantomjs添加系统环境变量里
3.编写js文件capture.js"use strict"; //严格模式 var page = require('webpage').create(); var system = require('system'); page.viewportSize = { width : 1024, height : 720 }; if (system.args.length < 3) { console.log('param must greater 2'); phantom.exit(); } else{ var url = system.args[1]; //远程视频地址 var saveFile = system.args[2]; //保存截图的文件路径 page.open(url, function(status) { if (status == 'success'){ // 通过在JS获取页面的渲染高度 var rect = page.evaluate(function () { return document.getElementsByTagName('html')[0].getBoundingClientRect(); }); // 按照实际页面的高度,设定渲染的宽高 page.clipRect = { top: rect.top, left: rect.left, width: rect.width, height: rect.height }; setTimeout(function() { var result = page.render(saveFile); page.close(); console.log(result); phantom.exit(); }, 1000); //延迟截图时间 } }) }
4.在php中进行调用
$url = 'http://xxx'; $savePath = 'c:\test.png'; $jsPath = 'c:\phantomjs.js'; $command = "phantomjs {$jsPath} {$url} {$savePath}"; $result = @exec($command );
这样就对网页进行截图,保存截图在指定路径中。
另外:有大神在github上提交了个操作phantomjs的php类库,可以参考使用:
https://github.com/jonnnnyw/php-phantomjs
http://jonnnnyw.github.io/php-phantomjs/4.0/2-installation/
参考自:
http://phantomjs.org/download.html
http://phantomjs.org/api/webpage/method/render.html
代码实例:
phantomjs和slimerjs,两款都是服务器端的js,简单说来,都是封装了浏览器解析引擎,不同是phantomjs封装的 webkti,slimerjs封装的是Gecko(firefox)。 权衡利弊,决定研究下phantomjs,于是就用phantomjs实现了网 站快照生成。phantomjs的项目地址是:http://phantomjs.org/
代码涉及两个部分,一个是设计业务的index.php,另一个是生成快照的js脚本snapshot.js。代码比较简单,仅仅是实现了功能,没有做过多的修饰。代码分别如下所示:
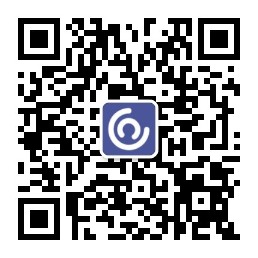
php:
<?php
if (isset($_GET['url']))
{
set_time_limit(0);
$url = trim($_GET['url']);
$filePath = "./cache/".md5($url).'.png';
if (is_file($filePath))
{
exit($filePath);
}
$command = "phantomjs/bin/phantomjs snapshot.js {$url} {$filePath}";
exec($command);
exit($filePath);
}
?>
html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta name="keywords" content="" />
<meta name="description" content="" />
<title>快照生成</title>
<script src="http://code.jquery.com/jquery-1.8.3.min.js"></script>
<style>
* {
margin: 0;
padding: 0;
}
form {
padding: 20px;
}
div {
margin: 20px 0 0;
}
input {
width: 200px;
padding: 4px 2px;
}
#placeholder {
display: none;
}
</style>
</head>
<body>
<form action="" id="form">
<input type="text" id="url" />
<button type="submit">生成快照</button>
<div>
<img src="" alt="" id="placeholder" />
</div>
</form>
<script>
$(function(){
$('#form').submit(function(){
if (typeof($(this).data('generate')) !== 'undefined' && $(this).data('generate') === true)
{
alert('正在生成网站快照,请耐心等待...');
return false;
}
$(this).data('generate', true);
$('button').text('正在生成快照...').attr('disabled', true);
$.ajax({
type: 'GET',
url: '?',
data: 'url=' + $('#url').val(),
success: function(data){
$('#placeholder').attr('src', data).show();
$('#form').data('generate', false);
$('button').text('生成快照').attr('disabled', false);
}
});
return false;
});
});
</script>
</body>
</html>
php利用CutyCapt实现网页高清截图 :
网页截图功能,必须安装IE+CutyCapturl:要截图的网页out:图片保存路径path:CutyCapt路径cmd:CutyCapt执行命令比如:http://你php路径.php?url=http://XX.com
<?php
/*
网页截图功能,必须安装IE+CutyCapt
url:要截图的网页
out:图片保存路径
path:CutyCapt路径
cmd:CutyCapt执行命令
比如:http://你php路径.php?url=http://niutuku9.com/
*/
$url=$_GET["url"];
$imgname=str_replace('http://','',$url);
$imgname=str_replace('https://','',$imgname);
$imgname=str_replace('.','-',$imgname);
$out = 'D:/webroot/test/'.$imgname.'.png';
$path = 'D:/webserver/CutyCapt.exe';
$cmd = "$path --url=$url --out=$out";
echo $cmd;
system($cmd);
?>
CutyCapt下载地址:http://sourceforge.net/projects/cutycapt/files/cutycapt/
windows的不用安装的,直接下载解压放到相对应的路径即可
linux安装CutyCapt教程:http://niutuku9.com/tech/php/273578.shtml
整理参考来自:软盟网