asyncio
是Python 3.4版本引入的标准库,直接内置了对异步IO的支持。
import asyncio
@asyncio.coroutine
def hello():
print("Hello world!")
# 异步调用asyncio.sleep(1):
r = yield from asyncio.sleep(1)
print("Hello again!")
# 获取EventLoop:
loop = asyncio.get_event_loop()
# 执行coroutine
loop.run_until_complete(hello())
loop.close()
对yield关键字不清楚的同学看这里:https://blog.csdn.net/mieleizhi0522/article/details/82142856
对yield from关键字不清楚的同学看这里:
https://blog.csdn.net/chenbin520/article/details/78111399 #很棒,推荐
https://blog.csdn.net/soonfly/article/details/78361819
然后,为了简化并更好地标识异步IO,从Python 3.5开始引入了新的语法async
和await
请注意,async
和await
是针对coroutine的新语法,要使用新的语法,只需要做两步简单的替换:
- 把
@asyncio.coroutine
替换为async
- 把
yield from
替换为await
再看一个栗子,对比同步IO和异步IO
#同步IO
import time
def hello():
time.sleep(1)
def run():
for i in range(5):
hello()
print('Hello World:%s' % time.time()) # 任何伟大的代码都是从Hello World 开始的!
if __name__ == '__main__':
run()
输出是:
Hello World:1527595175.4728756
Hello World:1527595176.473001
Hello World:1527595177.473494
Hello World:1527595178.4739306
Hello World:1527595179.474482
------------------------------------------------------------------------
#异步IO:
import time
import asyncio
# 定义异步函数
async def hello():
asyncio.sleep(1)
print('Hello World:%s' % time.time())
def run():
for i in range(5):
loop.run_until_complete(hello())
loop = asyncio.get_event_loop()
if __name__ =='__main__':
run()
输出是:
Hello World:1527595104.8338501
Hello World:1527595104.8338501
Hello World:1527595104.8338501
Hello World:1527595104.8338501
Hello World:1527595104.8338501
再来一个栗子,调用多个协程函数:
import threading
import asyncio
import time
async def hello_A():
print('A----Hello world! (%s)' % threading.currentThread() , time.time())
await asyncio.sleep(1)
print('A----Hello again! (%s)' % threading.currentThread() , time.time())
async def hello_B():
print('B----Hello world! (%s)' % threading.currentThread() , time.time())
await asyncio.sleep(1)
print('B----Hello again! (%s)' % threading.currentThread() , time.time())
loop = asyncio.get_event_loop()
tasks = [hello_A(), hello_B()]
loop.run_until_complete(asyncio.wait(tasks))
loop.close()
Result:
A----Hello world! (<_MainThread(MainThread, started 54064)>) 1568095270.9067237
B----Hello world! (<_MainThread(MainThread, started 54064)>) 1568095270.9067237
A----Hello again! (<_MainThread(MainThread, started 54064)>) 1568095271.9067764
B----Hello again! (<_MainThread(MainThread, started 54064)>) 1568095271.9067764
展示两个错误的调用方式:
扫描二维码关注公众号,回复:
9393403 查看本文章
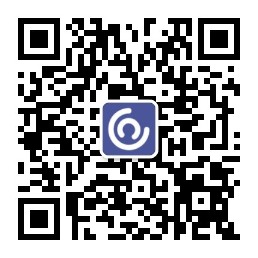
import threading
import asyncio
import time
async def hello_A():
print('A----Hello world! (%s)' % threading.currentThread() , time.time())
await asyncio.sleep(1)
print('A----Hello again! (%s)' % threading.currentThread() , time.time())
async def hello_B():
print('B----Hello world! (%s)' % threading.currentThread() , time.time())
await asyncio.sleep(1)
print('B----Hello again! (%s)' % threading.currentThread() , time.time())
loop = asyncio.get_event_loop()
tasks = [hello_A(), hello_B()]
#loop.run_until_complete(asyncio.wait(tasks))
loop.run_until_complete(hello_A())
loop.run_until_complete(hello_B())
loop.close()
Result:
A----Hello world! (<_MainThread(MainThread, started 47500)>) 1568095328.6755295
A----Hello again! (<_MainThread(MainThread, started 47500)>) 1568095329.6770687
B----Hello world! (<_MainThread(MainThread, started 47500)>) 1568095329.6770687
B----Hello again! (<_MainThread(MainThread, started 47500)>) 1568095330.677155
--------------------------------------------------------------------------------
import threading
import asyncio
import time
async def hello_A():
while True:
print('A----Hello world! (%s)' % threading.currentThread() , time.time())
await asyncio.sleep(1)
print('A----Hello again! (%s)' % threading.currentThread() , time.time())
async def hello_B():
while True:
print('B----Hello world! (%s)' % threading.currentThread() , time.time())
await asyncio.sleep(1)
print('B----Hello again! (%s)' % threading.currentThread() , time.time())
loop = asyncio.get_event_loop()
tasks = [hello_A(), hello_B()]
#loop.run_until_complete(asyncio.wait(tasks))
loop.run_until_complete(hello_A())
loop.run_until_complete(hello_B())
loop.close()
Result:
A----Hello world! (<_MainThread(MainThread, started 21096)>) 1568095399.9003077
A----Hello again! (<_MainThread(MainThread, started 21096)>) 1568095400.9008029
A----Hello world! (<_MainThread(MainThread, started 21096)>) 1568095400.9008029
A----Hello again! (<_MainThread(MainThread, started 21096)>) 1568095401.9012694
A----Hello world! (<_MainThread(MainThread, started 21096)>) 1568095401.9012694
A----Hello again! (<_MainThread(MainThread, started 21096)>) 1568095402.9012957
A----Hello world! (<_MainThread(MainThread, started 21096)>) 1568095402.9012957
A----Hello again! (<_MainThread(MainThread, started 21096)>) 1568095403.9016788
A----Hello world! (<_MainThread(MainThread, started 21096)>) 1568095403.9016788
参考资料:
https://www.liaoxuefeng.com/wiki/1016959663602400/1017970488768640
https://blog.csdn.net/m345376054/article/details/78538726