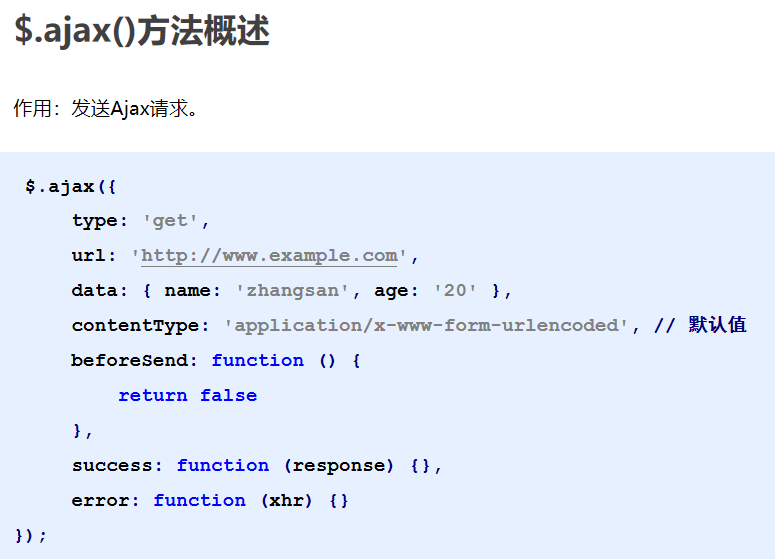
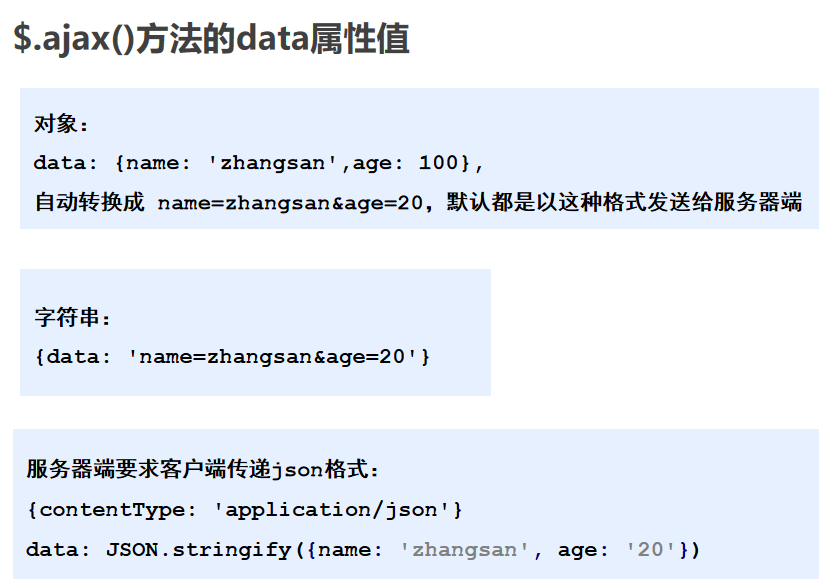
01.$.ajax方法基本使用.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$.ajax方法基本使用</title>
</head>
<body>
<button id="btn">发送请求</button>
<script src="/js/jquery.min.js"></script>
<script>
$('#btn').on('click', function() {
$.ajax({
type: 'post', // 请求方式
url: '/base', // 请求地址 【如果协议、域名、端口都相同,可不用写完整的请求地址。】
// 请求成功以后函数被调用
success: function(response) {
// response为服务器端返回的数据
// 方法内部会自动将json字符串转换为json对象
console.log(response);
},
// 请求失败以后函数被调用
error: function(xhr) {
console.log(xhr)
}
})
});
</script>
</body>
</html>
02.$.ajax方法传递请求参数.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$.ajax方法基本使用</title>
</head>
<body>
<button id="btn">发送请求</button>
<script src="/js/jquery.min.js"></script>
<script>
var params = {
name: 'wangwu',
age: 300
}
$('#btn').on('click', function() {
$.ajax({
type: 'post', // 请求方式
url: '/user', // 请求地址 【如果协议、域名、端口都相同,可不用写完整的请求地址。】
// 向服务器端发送的请求参数
// 自动转换成 name=zhangsan&age=100,然后发送给服务器端
// data: {
// name: 'zhangsan',
// age: 100
// },
data: JSON.stringify(params),
contentType: 'application/json', // 指定参数的格式类型
// 请求成功以后函数被调用
success: function(response) {
// response为服务器端返回的数据
// 方法内部会自动将json字符串转换为json对象
console.log(response);
}
})
});
</script>
</body>
</html>
03.beforeSend方法的说明.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>$.ajax方法基本使用</title>
</head>
<body>
<button id="btn">发送请求</button>
<script src="/js/jquery.min.js"></script>
<script>
$('#btn').on('click', function() {
$.ajax({
type: 'post',
url: '/user',
// 在请求发送之前调用
beforeSend: function() {
alert('请求不会被发送')
return false; // 请求不会被发送
},
// 请求成功以后函数被调用
success: function(response) {
// response为服务器端返回的数据
// 方法内部会自动将json字符串转换为json对象
console.log(response);
}
})
});
</script>
</body>
</html>