一、全局获取Context
Android提供了一个Application类,每当程序启动的时候就会自动将这个类初始化。
定制一个自己的Application类,以便于管理程序内一些全局的状态信息,比如全局Context
。
MyApplication.java
public class MyApplication extends Application {
private static Context context;
@Override
public void onCreate() {
super.onCreate();
context = getApplicationContext();
}
public static Context getContext(){
return context;
}
}
接着要告知系统程序启动初始化MyApplication
类,而不是默认的Application
类。修改AndroidManifest.xml
:
<application
android:name="com.example.day22_skills.MyApplication"
不过这里要加上完整的包名,不然没法找到这个类
这样就实现了一种全局获取Context
的机制,之后不管在任何地方想要使用Context
,只需调用一下MyApplication.getContext()
就行
补充:为了使得
LitePal
兼容,还需要自己的Application
中使用LitePal.initalize(context)
二、使用Intent
传递对象
Intent
一般使用putExtra()
传递数值,可这样只能传递一些常用的数据类型,当想传递一些自定义对象却无从下手
1、Serializable
方式
序列化是将一个对象转换成可存储或可传输的状态,序列化只需要实现Serializable
接口即可
比如有一个自定义类People
,为了将他序列化,在实现Serializable
的同时设置所有字段的getter
和setter
即可
public class People implements Serializable {
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
发送数据:
Person person = new Person();
person.setName("Tom");
person.setAge(20);
Intent intent = new Intent(FirstActivity.this, SecondActivity.class);
intetn.putExtra("person_data", person);
startActivity(intent);
获取数据需要调用getSerializableExtra()
将序列换对象向下转型:
Person person = (Person) getIntent().getSerializableExta("person_data");
2、Parcelable
方式
这种方式比Serialzable
效率更高,但麻烦一些,需要将一个完整的对象分解,分解后的每一部分都是Intent
支持的类型:
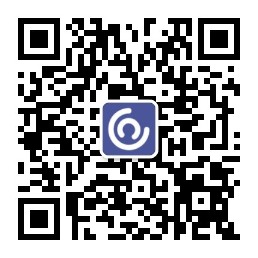
public class People implements Parcelable {
private String name;
private int age;
......
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeString(name);
dest.writeInt(age);
}
public static final Parcelable.Creator<People> CREATOR = new Parcelable.Creator<People>(){
@Override
public People createFromParcel(Parcel source) {
People people = new People();
people.name = source.readString();
people.age = source.readInt();
return people;
}
@Override
public People[] newArray(int size) {
return new People[size];
}
};
}
首先实现Parcelable
接口必须describeContents()
和writeToParcel()
,其中describeContents
直接返回0,而在writeToParcel()
中需要调用Parcel.writeXXX()
方法,将类其中的字段一一写出
接着在People类中提供一个名为CREATOR
的常量,这里提供了一个Parcelable.Creator
接口的一个实现,并将泛型指定为People
,接着继续重写createFromParcel()
和newArray()
:
createFromParcel()
:读取刚才写出的字段,并创建一个People对象并返回,这里读取的顺序必须和刚才写出的顺序相同newArray()
:new出一个People数组,并使用方法中传入的size
作为数组大小即可
这样获取数据:
People people - (People) getIntent().getParcelableExtra("person_data");
Parcelable
方式具有更高的运行效率,所以更推荐