1.this关键字
this关键字的四种常见用法:
- this调用本类属性
- this调用本类普通方法
- this调用本类构造方法
- this调用当前属性
1.1 this调用本类属性:
通过this关键字可以明确的去访问一个类的成员变量,解决与局部变量名称冲突的问题;
范例:
class Human{
int age;
public Human(int age){
this.age=age;
}
public int getAge(){
return this.age;
}
}
可以发现:当参数与类中属性同名时,类中属性无法被正确赋值。此时我们加上this关键字就可以正确的给对象属性赋值。在上面的例子中,构造方法中的参数age是局部变量,但类中的属性age是成员变量,在构造方法中如果使用age,则是访问局部变量,但如果使用this.age则是访问成员变量。
1.2 通过this调用本类成员方法
范例:
class Human{
public void openMouth(){
//省略内容
}
public void speak(){
this.openMouth();
}
}
虽然调用本类普通方法不需要加this也可以正常使用,但强烈建议加上,加上this的目的可以区分方法的定义来源(在继承中有用)。
1.3 this调用本类构造方法
构造方法是在实例化对象时被java虚拟机自动调用的,在程序中不能向调用其他方法一样去调用构造方法,但可以在一个构造方法中使用“this.([参数1,参数2…])”的形式来调用其他的构造方法。
范例:
class Human{
int age;
public Human(){
System.out.println("无参构造方法被调用了…");
}
public Human(int age){
//调用了无参的构造方法
this();
this.age=age;
System.out.println("有参的构造方法被调用了…");
}
}
public class Test {
public static void main(String[] args) {
Human h1=new Human(20);
}
}
结果为:
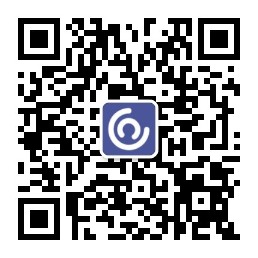
在使用this调用类的构造方法时,应注意:
- 只能在构造方法中使用this调用其他的构造方法,不能在成员方法中使用;
- 在构造方法中,使用this调用构造方法的语句必须位于第一行,且只能出现一次;下面的写法是错误的:
public Human(){
int age=20;
this(age); //调用有参的构造方法,由于不在第一行,编译错误
}
- 不能在一个类的两个构造方法中使用this互相调用,下面的写法编译会出错
class Human{
public Human(){
this(20);
System.out.println("无参构造方法被调用了…");
}
public Human(int age){
//调用了无参的构造方法
this();
this.age=age;
System.out.println("有参的构造方法被调用了…");
}
}
它不能两两互相调用,但是可以B调用A,C可以调用B,范例如下:
class Human{
int age;
String name;
public Human(){
System.out.println("无参构造方法被调用了…");
}
public Human(int age){
//调用了无参的构造方法
this();
this.age=age;
System.out.println("有参的构造方法被调用了…");
}
public Human(String name,int age){
//调用了有参的构造方法
this(age);
this.name=name;
System.out.println("有参的构造方法被调用了…");
}
}
1.4 this调用当前对象
class Human{
//this调用当前对象
public void print(){
System.out.println("print方法"+this);
}
}
public class Test {
public static void main(String[] args) {
Human h1=new Human();
System.out.println("main方法"+h1);
h1.print();
System.out.println("======================");
Human h2=new Human();
System.out.println("main方法"+h2);
h2.print();
}
}
运行结果:
只要对象调用了本类中的方法,这个this就表示当前执行的对象
2.super关键字
(super的具体使用参考之前写的博客:https://mp.csdn.net/postedit/102506011)
当子类重写父类的方法后,子类对象将无法访问父类被重写的方法,为了解决这个问题,在java中专门提供了一个super关键字用于访问父类的成员,
2.1 使用super关键字调用父类的成员变量和成员方法
格式为:
super.成员变量
super.成员方法()
2.2使用super关键字调用父类的构造方法
格式如下:
super([参数1,参数2])
3.this和super比较
super和this在使用上非常相似,但是两者最大的区别是super时子类访问父类,而this是本类的访问处理操作。
能使用super.方法()一定要明确标记是父类的操作。
1.子类覆写父类的方法是因为父类的方法功能不足才需要覆写。
2.方法覆写的时候使用就是public权限。
3.可以说父类是子类的子集。