1、Eclipse下Maven的父子结构展示
2、创建父项目,pom文件
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.curtain</groupId>
<artifactId>frame</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>pom</packaging>
<name>Curtain</name>
<!--
打包的时候,执行顺序
parent:防止所有依赖JAR
console:自定义的JAR(可以理解为项目的后端代码打包)
web:放置配置文件、相关页面
-->
<modules>
<module>curtain-parent</module>
<module>curtain-console</module>
<module>curtain-web</module>
</modules>
</project>
3、创建子项目parent,pom文件
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<groupId>com.curtain</groupId>
<artifactId>frame</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<groupId>com.curtain.frame</groupId>
<artifactId>curtain-parent</artifactId>
<packaging>pom</packaging>
<!--定制属性-->
<properties>
<java-version>1.7</java-version>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<testFailureIgnore>true</testFailureIgnore>
<test.skip>true</test.skip>
<org.springframework-version>4.2.0.RC3</org.springframework-version>
</properties>
<!--依赖包-->
<dependencies>
<!-- SPRING -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${org.springframework-version}</version>
<exclusions>
<!-- Exclude Commons Logging in favor of SLF4j -->
<exclusion>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context-support</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<!-- SPRING WEBSOCKET -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-messaging</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-websocket</artifactId>
<version>${org.springframework-version}</version>
</dependency>
</dependencies>
<build>
<plugins>
<!--插件版本-->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>${java-version}</source>
<target>${java-version}</target>
</configuration>
</plugin>
<!--插件跳过测试-->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<skip>${test.skip}</skip>
<testFailureIgnore>${testFailureIgnore}</testFailureIgnore>
</configuration>
</plugin>
<!--插件生命周期-->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-source-plugin</artifactId>
<executions>
<execution>
<id>attach-source</id>
<phase>package</phase><!-- 要绑定到的生命周期的阶段 -->
<goals>
<goal>jar-no-fork</goal><!-- 要绑定的插件的目标 -->
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
<repositories>
<repository>
<id>spring-releases</id>
<url>https://repo.spring.io/libs-release</url>
</repository>
<repository>
<id>spring-snapshots</id>
<url>http://repo.spring.io/snapshot</url>
<snapshots>
<enabled>true</enabled>
</snapshots>
<releases>
<enabled>false</enabled>
</releases>
</repository>
<repository>
<id>spring-milestones</id>
<url>http://repo.spring.io/milestone</url>
<snapshots>
<enabled>false</enabled>
</snapshots>
<releases>
<enabled>true</enabled>
</releases>
</repository>
</repositories>
<profiles>
<!--私服地址-->
<profile>
<id>wuhan_maven</id>
<activation>
<activeByDefault>true</activeByDefault>
</activation>
<distributionManagement>
<snapshotRepository>
<id>snapshots</id>
<url>http://ip:port/nexus/content/repositories/snapshots/</url>
</snapshotRepository>
<repository>
<id>releases</id>
<url>http://ip:port/nexus/content/repositories/releases/</url>
</repository>
</distributionManagement>
</profile>
<profile>
<id>beijing_maven</id>
<distributionManagement>
<snapshotRepository>
<id>snapshots</id>
<url>http://ip:port/nexus/content/repositories/snapshots/</url>
</snapshotRepository>
<repository>
<id>id</id>
<url>http://ip:port/nexus/content/repositories/releases/</url>
</repository>
</distributionManagement>
</profile>
</profiles>
</project>
4、创建子项目console,JAR文件
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>com.curtain</groupId>
<artifactId>frame</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<groupId>com.curtain.frame</groupId>
<artifactId>curtain-console</artifactId>
</project>
5、创建子项目web,WAR文件
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<!-- <parent>
<groupId>com.curtain.farme</groupId>
<artifactId>curtain-parent</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent> -->
<parent>
<groupId>com.curtain</groupId>
<artifactId>frame</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<groupId>com.curtain.farme</groupId>
<artifactId>curtain-web</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name>curtain-web</name>
<dependencies>
</dependencies>
<!--编译并运行tomcat: war:exploded tomcat7:run -->
<!--打包代码: install -->
<build>
<plugins>
<plugin>
<artifactId>maven-war-plugin</artifactId>
<configuration>
<!-- class文件会自动打JAR包 -->
<archiveClasses>false</archiveClasses>
<failOnMissingWebXml>false</failOnMissingWebXml>
<!--覆盖-->
<overlays>
<overlay>
<groupId>com.curtain.farme</groupId>
<artifactId>curtain-web</artifactId>
</overlay>
</overlays>
</configuration>
</plugin>
</plugins>
</build>
</project>
6、运行父项目的POM文件,打包效果
注意:
1、新建Maven项目,勾选这个,免去选择模板
扫描二维码关注公众号,回复:
927660 查看本文章
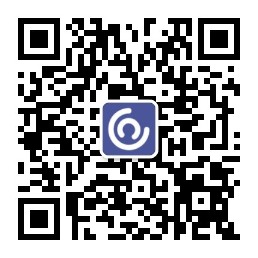
2、想要显示父子结构,除了要按照这样添加POM之外,还需要在父项目的POM中设置
2.1Add是添加已存在的Maven项目在父项目下
2.2Create是创建一个新的Maven项目在父项目下