项目地址:https://github.com/qjyn1314/hunlun-buir
自学的实践,希望可以帮助到大家
搭建项目过程,略过
使用以下的步骤的时候,前提是已经实现了dubbo+zookeeper的配置
并在两个服务中没有异常的情况下,可以正常的插入到两个数据库数据记录
首先第一步引入,seata的版本:
<!--seata-start--> <dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-seata</artifactId> <version>2.1.0.RELEASE</version> <scope>compile</scope> <exclusions> <exclusion> <groupId>io.seata</groupId> <artifactId>seata-all</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>io.seata</groupId> <artifactId>seata-all</artifactId> <version>1.0.0</version> </dependency> <!--seata-end-->
第二步:
创建 SeataTransactionConfig 配置类:
/** * 创建数据源 * * @return javax.sql.DataSource * @author wangjunming * @since 2020/1/23 15:25 */ @Bean("druidDataSource") @ConfigurationProperties(prefix = "spring.datasource") public DruidDataSource druidDataSource() { return new DruidDataSource(); } /** * 注入seata数据源 * Seata 是通过代理数据源实现分布式事务,所以需要先配置一个数据源的代理,否则事务不会回滚。 * * @param druidDataSource: * @return io.seata.rm.datasource.DataSourceProxy * @author wangjunming * @since 2020/1/21 18:52 */ @Bean @Primary public DataSourceProxy dataSourceProxy(@Qualifier("druidDataSource") DruidDataSource druidDataSource) { return new DataSourceProxy(druidDataSource); } /** * 创建分布式事务的扫描 * * @return io.seata.spring.annotation.GlobalTransactionScanner * @author wangjunming * @since 2020/1/23 15:26 */ @Bean public DataSourceTransactionManager transactionManager(DataSourceProxy dataSourceProxy) { return new DataSourceTransactionManager(dataSourceProxy); }
第三步:
在每个项目的appliction.properties配置文件中进行配置:
扫描二维码关注公众号,回复:
9264871 查看本文章
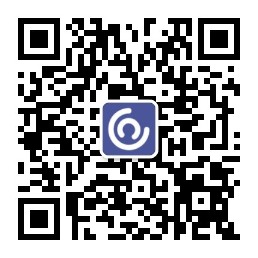
#seata分布式事务异常回滚,实现分布式事务的解决方案,事务组: spring.cloud.alibaba.seata.tx-service-group=my_test_tx_group
第四步:
在resources文件夹中进行创建两个文件:
参考:
registry.conf--seata使用什么类型:
registry { # file 、nacos 、eureka、redis、zk、consul、etcd3、sofa type = "file" nacos { serverAddr = "localhost" namespace = "public" cluster = "default" } eureka { serviceUrl = "http://localhost:1001/eureka" application = "default" weight = "1" } redis { serverAddr = "localhost:6379" db = "0" } zk { cluster = "default" serverAddr = "127.0.0.1:2181" session.timeout = 6000 connect.timeout = 2000 } consul { cluster = "default" serverAddr = "127.0.0.1:8500" } etcd3 { cluster = "default" serverAddr = "http://localhost:2379" } sofa { serverAddr = "127.0.0.1:9603" application = "default" region = "DEFAULT_ZONE" datacenter = "DefaultDataCenter" cluster = "default" group = "SEATA_GROUP" addressWaitTime = "3000" } file { name = "file.conf" } } config { # file、nacos 、apollo、zk type = "file" nacos { serverAddr = "localhost" namespace = "public" cluster = "default" } apollo { app.id = "seata-server" apollo.meta = "http://192.168.1.204:8801" } zk { serverAddr = "127.0.0.1:2181" session.timeout = 6000 connect.timeout = 2000 } file { name = "file.conf" } }
file.conf--seata使用的配置信息
transport { # tcp udt unix-domain-socket type = "TCP" #NIO NATIVE server = "NIO" #enable heartbeat heartbeat = true # the client batch send request enable enable-client-batch-send-request = true #thread factory for netty thread-factory { boss-thread-prefix = "NettyBoss" worker-thread-prefix = "NettyServerNIOWorker" server-executor-thread-prefix = "NettyServerBizHandler" share-boss-worker = false client-selector-thread-prefix = "NettyClientSelector" client-selector-thread-size = 1 client-worker-thread-prefix = "NettyClientWorkerThread" # netty boss thread size,will not be used for UDT boss-thread-size = 1 #auto default pin or 8 worker-thread-size = 8 } shutdown { # when destroy server, wait seconds wait = 3 } serialization = "seata" compressor = "none" } service { #transaction service group mapping vgroup_mapping.my_test_tx_group = "default" #only support when registry.type=file, please don't set multiple addresses default.grouplist = "47.104.78.115:8091" #degrade, current not support enableDegrade = false #disable seata disableGlobalTransaction = false } client { rm { async.commit.buffer.limit = 10000 lock { retry.internal = 10 retry.times = 30 retry.policy.branch-rollback-on-conflict = true } report.retry.count = 5 table.meta.check.enable = false report.success.enable = true } tm { commit.retry.count = 5 rollback.retry.count = 5 } undo { data.validation = true log.serialization = "jackson" log.table = "undo_log" } log { exceptionRate = 100 } support { # auto proxy the DataSource bean spring.datasource.autoproxy = false } }
第五步:就是搭建seata服务,即事务管理服务
本项目中使用docker启动seata事务管理服务
参考:https://seata.io/en-us/docs/ops/deploy-by-docker.html
-d:为此docker镜像进行后台启动
docker run --name seata-server -p 8091:8091 -d seataio/seata-server:latest
直接启动默认就是以file类型进行启动
搭建seata分布式事务,参考的示例: