目录
1、教程
网页教程:C++教程 | 菜鸟教程
视频教程:https://www.imooc.com/u/1349694/courses?sort=publish (先从起航篇看起)
2、IDE下载
我用的是Vistual Studio 2015
下载地址:https://www.nocang.com/vs2015/
3、学习
3.1、案例一
要求1:提示用户输入一个整数,将该整数分别以8进制、10进制、16进制打印在屏幕上
要求2:提示用户输入一个布尔值(0或1),以布尔方式将值打印在屏幕上
扫描二维码关注公众号,回复:
9257340 查看本文章
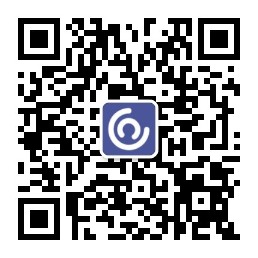
#include <iostream>
#include <stdlib.h>
using namespace std;
// 要求1:提示用户输入一个整数,将该整数分别以8进制、10进制、16进制打印在屏幕上
// 要求2:提示用户输入一个布尔值(0或1),以布尔方式将值打印在屏幕上
int main(){
// 1、提示用户 用 cout 进行数据的输出
cout << "请输入一个整数:" << endl;
// 2、用户进行输入 用 cin 进行数据的接收
int x = 0;
cin >> x;
// 3、将该整数分别以8进制、10进制、16进制打印在屏幕上
// oct dec hex 分别是8进制、10进制、16进制的英文缩写
cout << oct << x << endl;
cout << dec << x << endl;
cout << hex << x << endl;
cout << "请输入一个布尔值(0、1):" << endl;
bool y = false;
cin >> y;
cout << boolalpha << y << endl;
system("pause");
return 0;
}
3.2、命名空间(namespace)
#include<stdlib.h>
#include<iostream>
using namespace std;
namespace A {
int x = 1;
void fun() {
cout << "A" << endl;
}
}
namespace B {
int x = 2;
void fun() {
cout << "B" << endl;
}
void fun2() {
cout << "2B" << endl;
}
}
using namespace B;
int main() {
cout << A::x << endl;
B::fun();
B::fun2();
fun2;
system("pause");
return 0;
}
3.3、案例二
#include<stdlib.h>
#include<iostream>
using namespace std;
/*知识点:bool类型 命名空间 输入输出
题目要求:使用一个函数找出一个整型数组中的最大值或最小值
*/
/* 第一个int 是返回值
int *arr 传入一个数组
int count 数组元素的数量
bool isMax 告诉函数我们要的是最大值还是最小值;
如果传入的是true,说明要的是最大值;如果为传入的是false,说明要的是最小值;
*/
int getMaxOrMin(int *arr,int count,bool isMax) {
int temp = arr[0];
for (int i = 1; i < count;i++) {
if (isMax) {
if (temp < arr[i]) {
temp = arr[i];
}
}else {
if (temp > arr[i]) {
temp = arr[i];
}
}
}
return temp;
}
int main() {
int arr1[4] = { 3,5,1,7 };
bool isMax = false;
cin >> isMax;
cout << getMaxOrMin(arr1, 4, isMax) << endl;
system("pause");
return 0;
}