1,POM
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.hipad</groupId> <artifactId>data-jpa</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>data-jpa</name> <description>spring-data-jpa</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.0.2.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <!-- Web 依赖 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- 测试依赖 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> <version>RELEASE</version> </dependency> <!-- 模板引擎 Thymeleaf 依赖 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> <scope>runtime</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
2,resources
spring.jpa.show-sql=true
3,springboot入口
package com.hipad; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DataJpaApplication { public static void main(String[] args) { SpringApplication.run(DataJpaApplication.class, args); } }
4,Contorller
package com.hipad.controller; import com.hipad.domain.Book; import com.hipad.service.UserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.ModelMap; import org.springframework.web.bind.annotation.ModelAttribute; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; /** * @author: create by libin * @version: v1.0 * @description: com.hipad.controller * @date:2018/5/12 */ @Controller @RequestMapping(value = "book") public class UserController { @Autowired UserService userService; @RequestMapping(method = RequestMethod.GET) public String findPage(ModelMap map){ map.addAttribute("bookList",userService.findAll()); return "bookList"; } @RequestMapping(value = "create",method = RequestMethod.GET) public String createPage(ModelMap map){ Book book = new Book(); book.setName("java从入门到放弃"); book.setWriter("名字"); book.setIntroduction("这是讲述学习java的心酸历程"); map.addAttribute("book",book); map.addAttribute("action","create"); return "bookForm"; } @RequestMapping(value = "create",method = RequestMethod.POST) public String addPage(@ModelAttribute Book book){ Book save= userService.addAll(book); if(save!=null){ return "redirect:/book";// 这里注意写redirect:/book,不然拿不到值 } return "bookForm"; } @RequestMapping(value = "delete/{id}",method = RequestMethod.GET) public String delete(@PathVariable Long id){ userService.delete(id); return "redirect:/book"; } @RequestMapping(value = "update/{id}",method = RequestMethod.GET) public String update(ModelMap map,@PathVariable Long id){ Book book = userService.findById(id); map.addAttribute("book",book); map.addAttribute("action","update"); return "bookForm"; } @RequestMapping(value = "update",method = RequestMethod.POST) public String updateById(@ModelAttribute Book book){ userService.updateById(book); return "redirect:/book"; } }
5,service
package com.hipad.service; import com.hipad.domain.Book; import java.util.List; /** * @author: create by libin * @version: v1.0 * @description: com.hipad.service * @date:2018/5/12 */ public interface UserService { List<Book> findAll(); Book addAll(Book book); void delete(Long id); Book findById(Long id); void updateById(Book book); }
package com.hipad.service.impl; import com.hipad.domain.Book; import com.hipad.domain.UserRepository; import com.hipad.service.UserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.List; /** * @author: create by libin * @version: v1.0 * @description: com.hipad.service.impl * @date:2018/5/12 */ @Service public class UserServiceImpl implements UserService { @Autowired UserRepository userRepository; @Override public List<Book> findAll() { return userRepository.findAll(); } @Override public Book addAll(Book book) { Book save = userRepository.save(book); return save; } @Override public void delete(Long id) { userRepository.deleteById(id); } @Override public Book findById(Long id) { return userRepository.findById(id).get(); } @Override public void updateById(Book book) { userRepository.save(book); } }
6,domain
扫描二维码关注公众号,回复:
923239 查看本文章
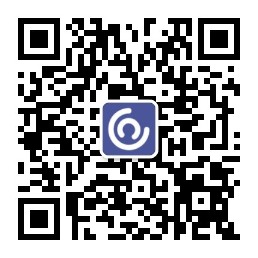
package com.hipad.domain; import org.springframework.data.jpa.repository.JpaRepository; /** * @author: create by libin * @version: v1.0 * @description: com.hipad.domain * @date:2018/5/12 */ public interface UserRepository extends JpaRepository<Book,Long>{ }
package com.hipad.domain; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import java.io.Serializable; /** * @author: create by libin * @version: v1.0 * @description: com.hipad.domain * @date:2018/5/12 */ @Entity public class Book implements Serializable { @Id @GeneratedValue private Long id; private String name; private String writer; private String introduction; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getWriter() { return writer; } public void setWriter(String writer) { this.writer = writer; } public String getIntroduction() { return introduction; } public void setIntroduction(String introduction) { this.introduction = introduction; } @Override public String toString() { return "Book{" + "id=" + id + ", name='" + name + '\'' + ", writer='" + writer + '\'' + ", introduction='" + introduction + '\'' + '}'; } }
th:action="@{/book/{action}(action=${action})}"