WPF灌水游戏
Xaml代码:
<Window x:Class="WPF灌水.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPF灌水"
mc:Ignorable="d"
Title="灌水游戏" WindowState="Maximized" Background="Linen" Loaded="Window_Loaded">
<Canvas x:Name="BG">
<Image x:Name="PoolImg" Width="140" Height="100" Stretch="Fill" Canvas.Left="1080" Canvas.Top="50" Source="img/Water.jpg"></Image>
<Image x:Name="VImg" Width="100" Height="100" Canvas.Left="1100" Canvas.Top="150" Source="img/V.jpg"></Image>
<Grid x:Name="Maps" Width="700" Height="700" Canvas.Left="800" Canvas.Top="250">
<Grid.RowDefinitions>
<RowDefinition></RowDefinition>
<RowDefinition></RowDefinition>
<RowDefinition></RowDefinition>
<RowDefinition></RowDefinition>
<RowDefinition></RowDefinition>
<RowDefinition></RowDefinition>
<RowDefinition></RowDefinition>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
</Grid.ColumnDefinitions>
</Grid>
</Canvas>
</Window>
Field类:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WPF灌水
{
class Field
{
public Field(int i,int j)
{
RightH = LeftH = UpH = DownH = true;
CanThrong = new Pipe(0);
if (i==0)
{
UpH = false;
}
if (i==6)
{
DownH = false;
}
if (j==0)
{
LeftH = false;
}
if (j==6)
{
RightH = false;
}
}
public Pipe CanThrong { get; set; }
public int ImgIndex { get; set; }
public bool isPd { get; set; }
public bool RightH { get; set; }
public bool LeftH { get; set; }
public bool UpH { get; set; }
public bool DownH { get; set; }
}
}
Pipe类
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WPF灌水
{
class Pipe
{
public Pipe(int index)
{
ImgIndex = index;
switch (index)
{
case 1:
isDown = isRight = true;
break;
case 2:
isDown = isLeft = true;
break;
case 3:
isLeft = isUp = true;
break;
case 4:
isUp = isRight = true;
break;
case 5:
isLeft = isUp = isDown = true;
break;
case 6:
isLeft = isRight = isUp = true;
break;
case 7:
isUp = isDown = isRight = true;
break;
case 8:
isLeft = isRight = isDown = true;
break;
case 9:
isLeft = isRight = true;
break;
case 10:
isUp = isDown = true;
break;
case 11:
isDown = isUp = isLeft = isRight = true;
break;
default:
isDown = isUp = isLeft = isRight = false;
break;
}
}
public int ImgIndex { get; set; }
public bool isLeft { get; set; }
public bool isRight { get; set; }
public bool isUp { get; set; }
public bool isDown { get; set; }
}
}
Xaml.cs代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace WPF灌水
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
Image[,] fieldMaps;
Field[,] fieldList;
private void Window_Loaded(object sender, RoutedEventArgs e)
{
fieldMaps = new Image[Maps.RowDefinitions.Count, Maps.ColumnDefinitions.Count];
fieldList = new Field[fieldMaps.GetLength(0), fieldMaps.GetLength(1)];
for (int i = 0; i <fieldMaps.GetLength(0); i++)
{
for (int j = 0; j <fieldMaps.GetLength(1); j++)
{
fieldMaps[i,j]= new Image();
fieldMaps[i, j].Tag = new int[2] { i, j };
fieldMaps[i, j].Width = fieldMaps[i, j].Height = 100;
fieldMaps[i, j].Source = new BitmapImage(new Uri("img/field.jpg", UriKind.Relative));
Grid.SetRow(fieldMaps[i, j], i);
Grid.SetColumn(fieldMaps[i, j], j);
Maps.Children.Add(fieldMaps[i, j]);
fieldMaps[i, j].MouseDown += MainWindow_MouseDown;
fieldList[i, j] = new Field(i,j);
}
}
ReadyPipe();
PoolImg.MouseDown += PoolImg_MouseDown;
}
Random r = new Random();
Image[] ready = new Image[4];
Pipe[] rpipes = new Pipe[4];
void ReadyPipe()
{
for (int i = 0; i < ready.Length; i++)
{
ready[i] = new Image();
if (i==0)
{
ready[i].Width = ready[i].Height = 120;
Canvas.SetLeft(ready[i], 500);
Canvas.SetTop(ready[i], 90);
}
else
{
ready[i].Width = ready[i].Height = 100;
Canvas.SetLeft(ready[i], Canvas.GetLeft(ready[i-1])-ready[i].Width-20);
Canvas.SetTop(ready[i], 100);
}
int index = r.Next(1, 12);
rpipes[i] = new Pipe(index);
ready[i].Source = new BitmapImage(new Uri("img/Ready/"+index+".jpg", UriKind.Relative));
BG.Children.Add(ready[i]);
}
}
private void MainWindow_MouseDown(object sender, MouseButtonEventArgs e)
{
Image img = sender as Image;
img.MouseDown-= MainWindow_MouseDown;
int[] location = img.Tag as int[];
fieldMaps[location[0], location[1]].Source = ready[0].Source;
fieldList[location[0], location[1]].CanThrong= rpipes[0];
fieldList[location[0], location[1]].ImgIndex = rpipes[0].ImgIndex;
ChangePipe();
}
void ChangePipe()
{
for (int i = 0; i < ready.Length-1; i++)
{
ready[i].Source = ready[i + 1].Source;
rpipes[i] = rpipes[i + 1];
}
int index = r.Next(1, 12);
rpipes[ready.Length - 1] = new Pipe(index);
ready[ready.Length-1].Source = new BitmapImage(new Uri("img/Ready/" + index + ".jpg", UriKind.Relative));
}
private void PoolImg_MouseDown(object sender, MouseButtonEventArgs e)
{
VImg.Source= new BitmapImage(new Uri("img/Water/H10.jpg", UriKind.Relative));
if (fieldList[0, 3].CanThrong.isUp)
{
fieldMaps[0, 3].Source = new BitmapImage(new Uri("img/Water/H" + fieldList[0, 3].ImgIndex + ".jpg", UriKind.Relative));
ThorgthWater(0,3);
}
else
{
MessageBox.Show("游戏失败");
}
}
private void ThorgthWater(int i, int j)
{
if (fieldList[i,j].isPd==false)
{
fieldList[i, j].isPd = true;
if (fieldList[i, j].UpH && fieldList[i, j].CanThrong.isUp && fieldList[i - 1, j].CanThrong.isDown)
{
fieldMaps[i - 1, j].Source = new BitmapImage(new Uri("img/Water/H" + fieldList[i - 1, j].ImgIndex + ".jpg", UriKind.Relative));
ThorgthWater(i - 1, j);
}
if (fieldList[i, j].DownH && fieldList[i, j].CanThrong.isDown && fieldList[i + 1, j].CanThrong.isUp)
{
fieldMaps[i + 1, j].Source = new BitmapImage(new Uri("img/Water/H" + fieldList[i + 1, j].ImgIndex + ".jpg", UriKind.Relative));
ThorgthWater(i + 1, j);
}
if (fieldList[i, j].LeftH && fieldList[i, j].CanThrong.isLeft && fieldList[i, j - 1].CanThrong.isRight)
{
fieldMaps[i, j - 1].Source = new BitmapImage(new Uri("img/Water/H" + fieldList[i, j - 1].ImgIndex + ".jpg", UriKind.Relative));
ThorgthWater(i, j - 1);
}
if (fieldList[i, j].RightH && fieldList[i, j].CanThrong.isRight && fieldList[i, j + 1].CanThrong.isLeft)
{
fieldMaps[i, j + 1].Source = new BitmapImage(new Uri("img/Water/H" + fieldList[i, j + 1].ImgIndex + ".jpg", UriKind.Relative));
ThorgthWater(i, j + 1);
}
}
}
}
}
运行结果:
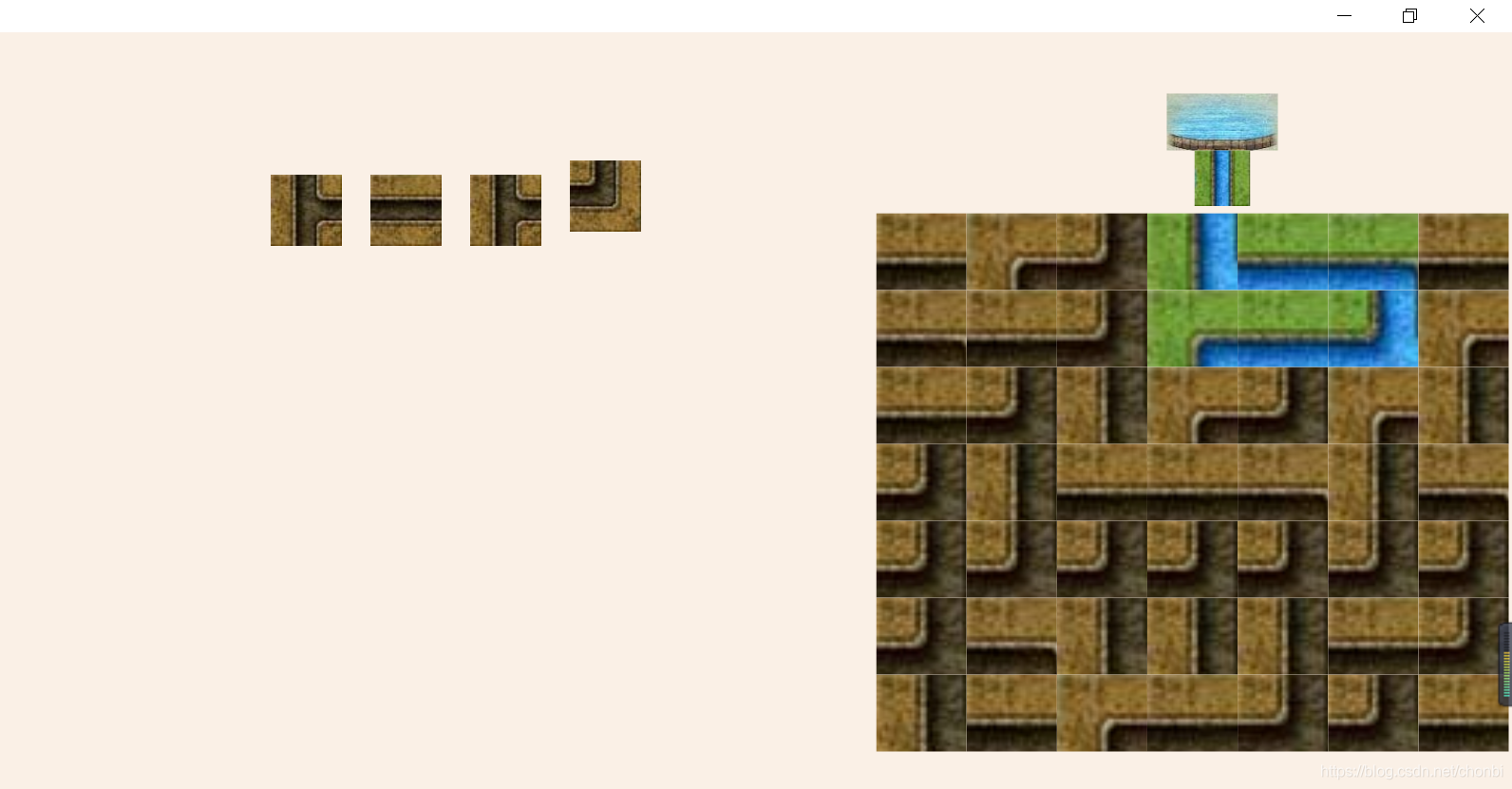