题目:https://atcoder.jp/contests/abc155/tasks/abc155_c
这道题的题意是给我们n个string,让我们统计每个string出现的次数,并输出次数最多的一个或多个string(按字典序来排列)
当时我想的是用数组来统计每个string,之后再sort一次遍历输出答案,但是我却不知道怎么用代码来实现,之后想到应该可以用map,但无奈不太熟练,不知道如何使用。
所以去网上学习了一下,map是STL的一个关联容器,提供1对1的hash.
第一个可以称为关键字(key),每个关键字只能在map中出现一次;
第二个可能称为该关键字的值(value);
map可以自动建立key--value的值.key和value可以是你任意需要的类型.
插入元素
// 定义一个map对象
map<int, string> mapStudent;
// 第一种 用insert函數插入pair
mapStudent.insert(pair<int, string>(000, "student_zero"));
// 第二种 用insert函数插入value_type数据
mapStudent.insert(map<int, string>::value_type(001, "student_one"));
// 第三种 用"array"方式插入
mapStudent[123] = "student_first";
mapStudent[456] = "student_second";
遍历!!
之前从hcy学长那里学到了直接用迭代器来遍历,其实也就等于一个for循环,但是map不能轻易用for循环来遍历 比如(for(int i=0;i<map.size();i++)),这里就相当于我们自动默认了map的key为int,当然不对!
于是我们使用迭代器来遍历map
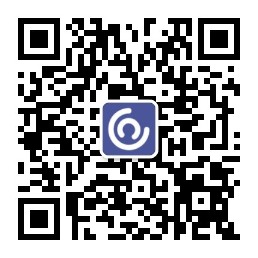
map<x,y> some::iterator iter;
for(iter=some.begin();iter!=map.end();i++)
附:map的各种函数
begin() 返回指向map头部的迭代器
clear() 删除所有元素
count() 返回指定元素出现的次数
empty() 如果map为空则返回true
end() 返回指向map末尾的迭代器
equal_range() 返回特殊条目的迭代器对
erase() 删除一个元素
find() 查找一个元素
get_allocator() 返回map的配置器
insert() 插入元素
key_comp() 返回比较元素key的函数
lower_bound() 返回键值>=给定元素的第一个位置
max_size() 返回可以容纳的最大元素个数
rbegin() 返回一个指向map尾部的逆向迭代器
rend() 返回一个指向map头部的逆向迭代器
size() 返回map中元素的个数
swap() 交换两个map
upper_bound() 返回键值>给定元素的第一个位置
value_comp() 返回比较元素value的函数
AC代码:
/*================================================ # ~ file:ABC155_C.cpp # ~~ date:2020-02-16 # ~~~author: SoMnus_L # |everybody finds love,in the end| # 人の運命か,人の希望は悲しみにつづられているね =================================================*/ #include <iostream> #include <cstdio> #include <cstring> #include <string.h> #include <math.h> #include <algorithm> #include <stack> #include <queue> #include <vector> #include <map> #include <set> #define ll long long const int N=1e6+10; using namespace std; typedef pair<int,int>PII; int n; int ans=-1; map<string,int> a; int main(){ ios::sync_with_stdio(false); cin>>n; while(n--){ string s; cin>>s; a[s]++; ans=max(ans,a[s]); } map<string,int>::iterator iter; for(iter=a.begin();iter!=a.end();iter++){ if(iter->second==ans) cout<<iter->first<<endl; } return 0; }