按照《深入理解Java虚拟机》中测试内存分配与回收策略中的代码进行了测试,
JDK8官方文档中JVM相关的文档地址https://docs.oracle.com/javase/8/docs/technotes/guides/vm/gctuning/
测试代码如下,使用的是JDK8,默认的垃圾收集器是Parallel Scavenge+Parallel Old
public class TestAllocation {
private static final int _1MB = 1024 * 1024;
public static void main(String[] args) {
byte[] allocation1, allocation2, allocation3, allocation4;
allocation1 = new byte[2 * _1MB];
allocation1 = new byte[2 * _1MB];
allocation1 = new byte[2 * _1MB];
allocation1 = new byte[4 * _1MB];
}
}
在使用Serial/Serial Old 垃圾收集器的情况下测试结果如下:
[root@iZbp13pwlxqwiu1xxb6szsZ com]# java -verbose:gc -Xms20M -Xmx20M -Xmn10M -XX:+PrintGCDetails -XX:SurvivorRatio=8 -XX:+UseSerialGC TestAllocation
[GC (Allocation Failure) [DefNew: 6815K->259K(9216K), 0.0030945 secs] 6815K->2307K(19456K), 0.0031645 secs] [Times: user=0.00 sys=0.00, real=0.00 secs]
Heap
def new generation total 9216K, used 4437K [0x00000000fec00000, 0x00000000ff600000, 0x00000000ff600000)
eden space 8192K, 51% used [0x00000000fec00000, 0x00000000ff014930, 0x00000000ff400000)
from space 1024K, 25% used [0x00000000ff500000, 0x00000000ff540c98, 0x00000000ff600000)
to space 1024K, 0% used [0x00000000ff400000, 0x00000000ff400000, 0x00000000ff500000)
tenured generation total 10240K, used 2048K [0x00000000ff600000, 0x0000000100000000, 0x0000000100000000)
the space 10240K, 20% used [0x00000000ff600000, 0x00000000ff800010, 0x00000000ff800200, 0x0000000100000000)
Metaspace used 2492K, capacity 4486K, committed 4864K, reserved 1056768K
class space used 267K, capacity 386K, committed 512K, reserved 1048576K
[root@iZbp13pwlxqwiu1xxb6szsZ com]#
发生一次Minor GC,新生代空间由6815K变成了259K,
在使用Parallel Scavenge/Parallel Old垃圾收集器测试结果如下:
[root@iZbp13pwlxqwiu1xxb6szsZ com]# java -verbose:gc -Xms20M -Xmx20M -Xmn10M -XX:+PrintGCDetails -XX:SurvivorRatio=8 TestAllocation
Heap
PSYoungGen total 9216K, used 6980K [0x00000000ff600000, 0x0000000100000000, 0x0000000100000000)
eden space 8192K, 85% used [0x00000000ff600000,0x00000000ffcd1020,0x00000000ffe00000)
from space 1024K, 0% used [0x00000000fff00000,0x00000000fff00000,0x0000000100000000)
to space 1024K, 0% used [0x00000000ffe00000,0x00000000ffe00000,0x00000000fff00000)
ParOldGen total 10240K, used 4096K [0x00000000fec00000, 0x00000000ff600000, 0x00000000ff600000)
object space 10240K, 40% used [0x00000000fec00000,0x00000000ff000010,0x00000000ff600000)
Metaspace used 2491K, capacity 4486K, committed 4864K, reserved 1056768K
class space used 267K, capacity 386K, committed 512K, reserved 1048576K
[root@iZbp13pwlxqwiu1xxb6szsZ com]#
新生代剩余的空间已经不足以存放allocation4对象,该对象直接被分配在老年代,此时并不会发生GC。
大对象直接进入老年代
我们直接分配一个4M的对象
public class TestAllocation {
private static final int _1MB = 1024 * 1024;
public static void main(String[] args) {
byte[] allocation1;
allocation1 = new byte[4 * _1MB];
}
}
虚拟机提供了一个-XX:PretenureSizeThreshold参数,令大于这个设置值的对象直接在老年代分配。这样做的目的是避免在Eden区及两个Survivor区之间发生大量的内存复制。
我们进行测试如下:
[root@iZbp13pwlxqwiu1xxb6szsZ com]# java -Xms20M -Xmx20M -Xmn10M -XX:+PrintGCDetails -XX:SurvivorRatio=8 -XX:+UseSerialGC TestAllocation
Heap
def new generation total 9216K, used 4932K [0x00000000fec00000, 0x00000000ff600000, 0x00000000ff600000)
eden space 8192K, 60% used [0x00000000fec00000, 0x00000000ff0d1000, 0x00000000ff400000)
from space 1024K, 0% used [0x00000000ff400000, 0x00000000ff400000, 0x00000000ff500000)
to space 1024K, 0% used [0x00000000ff500000, 0x00000000ff500000, 0x00000000ff600000)
tenured generation total 10240K, used 0K [0x00000000ff600000, 0x0000000100000000, 0x0000000100000000)
the space 10240K, 0% used [0x00000000ff600000, 0x00000000ff600000, 0x00000000ff600200, 0x0000000100000000)
Metaspace used 2491K, capacity 4486K, committed 4864K, reserved 1056768K
class space used 267K, capacity 386K, committed 512K, reserved 1048576K
[root@iZbp13pwlxqwiu1xxb6szsZ com]# java -Xms20M -Xmx20M -Xmn10M -XX:+PrintGCDetails -XX:SurvivorRatio=8 -XX:+UseSerialGC -XX:PretenureSizeThreshold=3145728 TestAllocation
Heap
def new generation total 9216K, used 835K [0x00000000fec00000, 0x00000000ff600000, 0x00000000ff600000)
eden space 8192K, 10% used [0x00000000fec00000, 0x00000000fecd0ff0, 0x00000000ff400000)
from space 1024K, 0% used [0x00000000ff400000, 0x00000000ff400000, 0x00000000ff500000)
to space 1024K, 0% used [0x00000000ff500000, 0x00000000ff500000, 0x00000000ff600000)
tenured generation total 10240K, used 4096K [0x00000000ff600000, 0x0000000100000000, 0x0000000100000000)
the space 10240K, 40% used [0x00000000ff600000, 0x00000000ffa00010, 0x00000000ffa00200, 0x0000000100000000)
Metaspace used 2491K, capacity 4486K, committed 4864K, reserved 1056768K
class space used 267K, capacity 386K, committed 512K, reserved 1048576K
[root@iZbp13pwlxqwiu1xxb6szsZ com]#
当没有使用-XX:PretenureSizeThreshold参数时,一个4M的对象分配到了Eden区,当使用了-XX:PretenureSizeThreshold=3145728,(就是3M,该参数不能直接写成3M)时,这个对象直接被分配到了老年代。
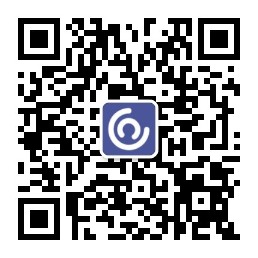
注意:PretenureSizeThreshold参数只对Serial和ParNew两款收集器有效,ParallelScavenge收集器不认识这个参数,ParallelScavenge收集器一般并不需要设置。如果遇到必须使用此参数的场合,可以考虑ParNew加CMS的收集器组合。