目录
(3)使用 byType 方式自动装配只需将 autowire 的值改成byType即可,需要注意的是,setter方法的属性必须与bean引用的类的类型相同
(4)使用 constructor 方式自动装配使用构造器自动装配,只需将 bean 修改成如下
no:默认方式,手动装配方式,需要通过ref设定bean的依赖关系
byName:根据bean的名字进行装配,当一个bean的名称和其他bean的属性一致,则自动装配
byType:根据bean的类型进行装配,当一个bean的属性类型与其他bean的属性的数据类型一致,则自动装配
constructor:根据构造器进行装配,与 byType 类似,如果bean的构造器有与其他bean类型相同的属性,则进行自动装配
autodetect:如果有默认构造器,则以constructor方式进行装配,否则以byType方式进行装配
(1)no 默认装配方式
示例代码
public class UserServiceImpl implements UserService{
@Override
public void query() {
// TODO Auto-generated method stub
System.out.println("装配完成");
}
}
public class UserDao {
private UserServiceImpl userServiceImpl;
public void query() {
userServiceImpl.query();
System.out.println("Dao执行");
}
public void setUserServiceImpl(UserServiceImpl userServiceImpl) {
this.userServiceImpl = userServiceImpl;
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans
xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.1.xsd">
<bean id="UserDao" class="com.lyq.zs.UserDao">
<property name="userServiceImpl" ref="userServiceImpl"></property>
</bean>
<bean id="userServiceImpl" class="com.lyq.zs.UserServiceImpl"/>
</beans>
扫描二维码关注公众号,回复:
9190085 查看本文章
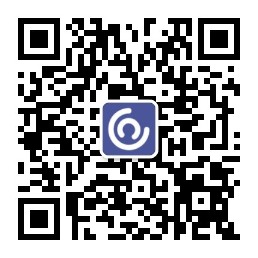
public static void main(String[] args) {
// TODO Auto-generated method stub
ApplicationContext ac=new ClassPathXmlApplicationContext("applicationContext.xml");
//UserService userService = (UserService)ac.getBean("userServiceImpl");
//userService.query();
UserDao dao = ac.getBean(UserDao.class);
dao.query();
}
可以看到,dao执行了自身的query方法
(2)使用 byName 自动装配
只需求修改 xml 配置为
<bean id="UserDao" class="com.lyq.zs.UserDao" autowire="byName">
</bean>
<bean id="userServiceImpl" class="com.lyq.zs.UserServiceImpl"/>
就可以自动装配,需要注意的是,byName方法下,setter方法要与bean的id对应,如bean的id为userServiceImpl,则setter方法就应该是setUserServiceImpl,否则会报错,例如将UserDao改成
public class UserDao {
private UserServiceImpl userServiceImpl1;
public void query() {
userServiceImpl1.query();
System.out.println("Dao执行");
}
public void setUserServiceImpl1(UserServiceImpl userServiceImpl) {
this.userServiceImpl1 = userServiceImpl;
}
}
则运行出错。
(3)使用 byType 方式自动装配
只需将 autowire 的值改成byType即可,需要注意的是,setter方法的属性必须与bean引用的类的类型相同
(4)使用 constructor 方式自动装配
使用构造器自动装配,只需将 bean 修改成如下
public class UserDao {
private UserServiceImpl userServiceImpl;
public UserDao(UserServiceImpl userServiceImpl) {
this.userServiceImpl = userServiceImpl;
}
public void query() {
userServiceImpl.query();
System.out.println("Dao执行");
}
/*public void setUserServiceImp(UserServiceImpl userServiceImpl) {
this.userServiceImpl = userServiceImpl;
}*/
}
并将 autowire 的值改成 constructor 即可
(5)使用 autodetect 方式自动装配
该方法会判断是否有默认构造器,如果有,则按照构造器装配,否则按照 byType 进行装配