RecyclerView是用来替代ListView、GridView的一个牛掰的控件。用起来更灵活,还能实现线性布局(横向、纵向)、网格布局、瀑布流等美观的UI。
在使用RecyclerView时候,必须指定一个适配器Adapter和一个布局管理器LayoutManager。适配器继承RecyclerView.Adapter类
首先在build.gradle中加入依赖库
dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'com.android.support:appcompat-v7:26.1.0' implementation 'com.android.support:recyclerview-v7:26.1.0'//添加RecycleView的依赖库 implementation 'com.android.support.constraint:constraint-layout:1.1.0' testImplementation 'junit:junit:4.12' androidTestImplementation 'com.android.support.test:runner:1.0.2' androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2' } |
布局文件activity_main.xml中添加recyclerview
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent">
<android.support.v7.widget.RecyclerView android:id="@+id/recycler_view" android:layout_width="match_parent" android:layout_height="match_parent" />
</LinearLayout> |
fruit_item.xml中
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="5dp">
<ImageView android:id="@+id/fruit_image" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal"/>
<TextView android:id="@+id/fruit_name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="left" android:layout_marginTop="10dp" />
</LinearLayout> |
Fruit类
package com.fengbang.recyclerviewtest;
public class Fruit {
private String name;
private int imageId;
public Fruit(String name, int imageId) { this.name = name; this.imageId = imageId; }
public String getName() { return name; }
public int getImageId() { return imageId; }
} |
FruitAdapter类
public class FruitAdapter extends RecyclerView.Adapter<FruitAdapter.ViewHolder> {
private List<Fruit> mFruitList;
static class ViewHolder extends RecyclerView.ViewHolder{ ImageView fruitImage; TextView fruitName; public ViewHolder(View view){ super(view);
fruitImage=(ImageView) view.findViewById(R.id.fruit_image); fruitName=(TextView) view.findViewById(R.id.fruit_name); } }
public FruitAdapter(List<Fruit> fruitList){ mFruitList=fruitList; }
@Override public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { View view= LayoutInflater.from(parent.getContext()).inflate(R.layout.fruit_item,parent,false); final ViewHolder holder=new ViewHolder(view); //自定义事件 holder.fruitImage.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { int position=holder.getAdapterPosition(); Fruit fruit = mFruitList.get(position); Toast.makeText(view.getContext(),fruit.getName(),Toast.LENGTH_SHORT).show(); } }); return holder; }
@Override public void onBindViewHolder(ViewHolder holder, int position) { Fruit fruit=mFruitList.get(position); holder.fruitImage.setImageResource(fruit.getImageId()); holder.fruitName.setText(fruit.getName());
}
@Override public int getItemCount() { return mFruitList.size(); } } |
MainActivity使用RecycleView
public class MainActivity extends AppCompatActivity {
private List<Fruit> fruitList = new ArrayList<Fruit>();
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
initFruits(); RecyclerView recyclerView = findViewById(R.id.recycler_view); // LinearLayoutManager linearLayoutManager = new LinearLayoutManager(this); // linearLayoutManager.setOrientation(LinearLayoutManager.HORIZONTAL);
StaggeredGridLayoutManager staggeredGridLayoutManager = new StaggeredGridLayoutManager(3,StaggeredGridLayoutManager.VERTICAL);
recyclerView.setLayoutManager(staggeredGridLayoutManager); FruitAdapter fruitAdapter = new FruitAdapter(fruitList); recyclerView.setAdapter(fruitAdapter); }
private void initFruits() { for (int i = 0; i < 2; i++) { Fruit apple = new Fruit(getRandomLengthName("Apple"), R.drawable.apple_pic); fruitList.add(apple); Fruit banana = new Fruit(getRandomLengthName("Banana"), R.drawable.banana_pic); fruitList.add(banana); Fruit orange = new Fruit(getRandomLengthName("Orange"), R.drawable.orange_pic); fruitList.add(orange); Fruit watermelon = new Fruit(getRandomLengthName("Watermelon"), R.drawable.watermelon_pic); fruitList.add(watermelon); Fruit pear = new Fruit(getRandomLengthName("Pear"), R.drawable.pear_pic); fruitList.add(pear); Fruit grape = new Fruit(getRandomLengthName("Grape"), R.drawable.grape_pic); fruitList.add(grape); Fruit pineapple = new Fruit(getRandomLengthName("Pineapple"), R.drawable.pineapple_pic); fruitList.add(pineapple); Fruit strawberry = new Fruit(getRandomLengthName("Strawberry"), R.drawable.strawberry_pic); fruitList.add(strawberry); Fruit cherry = new Fruit(getRandomLengthName("Cherry"), R.drawable.cherry_pic); fruitList.add(cherry); Fruit mango = new Fruit(getRandomLengthName("Mango"), R.drawable.mango_pic); fruitList.add(mango); } }
//为了瀑布流随机生成不同长度的字符串 private String getRandomLengthName(String name){ Random random = new Random(); int length=random.nextInt(20)+1; StringBuilder stringBuilder = new StringBuilder(); for (int i=0;i<length;i++){ stringBuilder.append(name); } return stringBuilder.toString(); } } |
最终结果
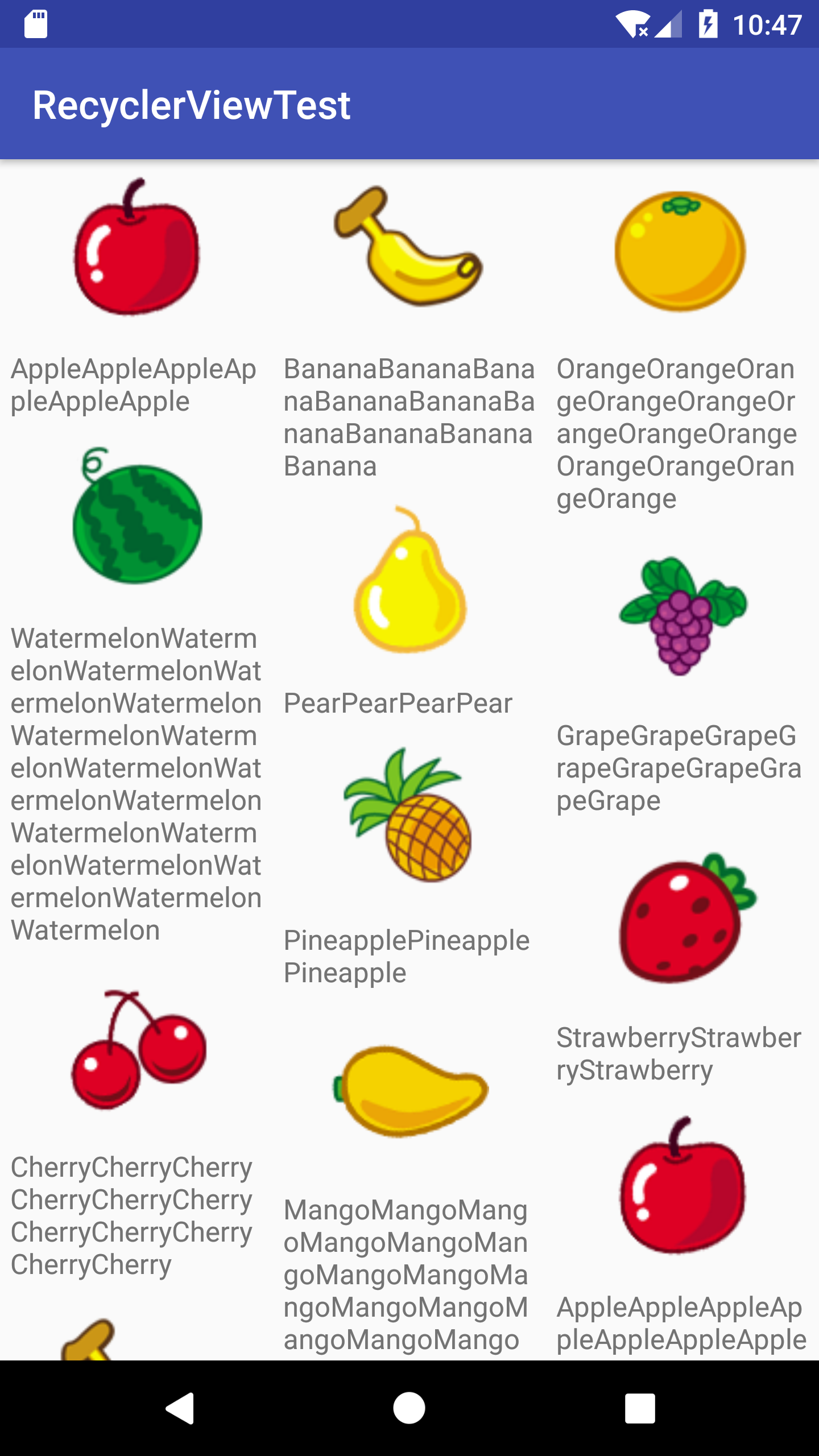