一、Fragments
react的组件在返回多个元素时,必须要用一个元素包裹起来,否则会报错,但是比如在表格中返回的列里加入了一个div如下:
则会导致html无效,所以react支持使用React.Fragment将多个元素包裹起来,这样并不会在html中添加任何元素。
在不需要key或其他属性时,还可以用一种更简洁的语法来实现:
class Columns extends React.Component { render() { return ( <> <td>Hello</td> <td>World</td> </> ); } }
二、Label标签
在react中,Label标签的for被写作htmlFor,用来寻找id为xxx的表单:
<label htmlFor="namedInput">Name:</label> <input id="namedInput" type="text" name="name"/>
三、如何找到各个DOM节点?
react可以通过给标签添加ref来操作各个标签,并且可以被传递到子组件中:
class CustomTextInput extends React.Component { constructor(props) { super(props); // 创造一个 textInput DOM 元素的 ref this.textInput = React.createRef(); } render() { // 使用 `ref` 回调函数以在实例的一个变量中存储文本输入 DOM 元素 //(比如,this.textInput)。 return ( <input type="text" ref={this.textInput} /> ); } } // 通过ref找到这个标签 focus() { // 使用原始的 DOM API 显式地聚焦在 text input 上 // 注意:我们通过访问 “current” 来获得 DOM 节点 this.textInput.current.focus(); }
扫描二维码关注公众号,回复:
9090238 查看本文章
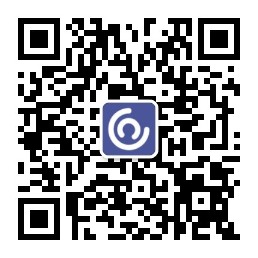
四、JSX
JSX实际上只是React.createElement(comonent, props, ...children)的语法汤,自定义的组件必须大写字母开头,因为小写字母开头的组件会被自动渲染成html标签,而大写字母开头的才会自动变成React.createElement(...)。
React元素类型不能是一个表达式,如果需要动态获取需要渲染的组件,可以通过一个大写字母开头的变量来获取:
const components = { photo: PhotoStory, video: VideoStory }; function Story(props) { // 正确!JSX 类型可以是大写字母开头的变量。 const SpecificStory = components[props.storyType]; return <SpecificStory story={props.story} />; }