总共分为Control,dao,enter,entity,service,util,view这几层。同时还含有一个mapperconfig.xml文件。
1,mapperconfig.xml
这里面用来配置相关数据库的连接和mapper的resource
1 <configuration> 2 <settings> 3 <setting name="cacheEnabled" value="true"/> 4 </settings> 5 <environments default="test"> 6 <environment id="test"> 7 <transactionManager type="JDBC"></transactionManager> 8 <dataSource type="POOLED"> 9 <property name="driver" value="com.mysql.jdbc.Driver"/> 10 <property name="url" value="jdbc:mysql://localhost:3306/bookstore?characterEncoding=UTF-8"/> 11 <property name="username" value="***"/> 12 <property name="password" value="****"/> 13 </dataSource> 14 </environment> 15 </environments> 16 <mappers> 17 <mapper resource="com/chinasofti/bookstore/dao/TypesDao.xml"/> 18 <mapper resource="com/chinasofti/bookstore/dao/BookDao.xml"/> 19 </mappers> 20 </configuration>
2,entity
这是实体类,没什么好说的。就是javabean掌握好就好。总共book和type两个实体类。
1(book)
1 package com.chinasofti.bookstore.entity; 2 3 public class Book { 4 private int bid; 5 private String bname; 6 private String author; 7 private String descn; 8 private int price; 9 private int num; 10 private Types t; 11 12 public Book(int bid, String bname, String author, String descn, int price, int num, Types t) { 13 this.bid = bid; 14 this.bname = bname; 15 this.author = author; 16 this.descn = descn; 17 this.price = price; 18 this.num = num; 19 this.t = t; 20 } 21 22 public Book(String bname, String author, String descn, int price, int num, Types t) { 23 this.bname = bname; 24 this.author = author; 25 this.descn = descn; 26 this.price = price; 27 this.num = num; 28 this.t = t; 29 } 30 31 public Book() { 32 } 33 34 public int getBid() { 35 return bid; 36 } 37 38 public void setBid(int bid) { 39 this.bid = bid; 40 } 41 42 public String getBname() { 43 return bname; 44 } 45 46 public void setBname(String bname) { 47 this.bname = bname; 48 } 49 50 public String getAuthor() { 51 return author; 52 } 53 54 public void setAuthor(String author) { 55 this.author = author; 56 } 57 58 public String getDescn() { 59 return descn; 60 } 61 62 public void setDescn(String descn) { 63 this.descn = descn; 64 } 65 66 public int getPrice() { 67 return price; 68 } 69 70 public void setPrice(int price) { 71 this.price = price; 72 } 73 74 public int getNum() { 75 return num; 76 } 77 78 public void setNum(int num) { 79 this.num = num; 80 } 81 82 public Types getT() { 83 return t; 84 } 85 86 public void setT(Types t) { 87 this.t = t; 88 } 89 90 @Override 91 public String toString() { 92 return "Book{" + 93 "bid=" + bid + 94 ", bname='" + bname + '\'' + 95 ", author='" + author + '\'' + 96 ", descn='" + descn + '\'' + 97 ", price=" + price + 98 ", num=" + num + 99 ", t=" + t + 100 '}'; 101 } 102 }
2(type)
package com.chinasofti.bookstore.entity; import java.io.Serializable; public class Types implements Serializable { private int tid; private String tname; public Types(int tid, String tname) { this.tid = tid; this.tname = tname; } public Types() { } public int getTid() { return tid; } public void setTid(int tid) { this.tid = tid; } public String getTname() { return tname; } public void setTname(String tname) { this.tname = tname; } @Override public String toString() { return "Types{" + "tid=" + tid + ", tname='" + tname + '\'' + '}'; } }
3,dao
bookdao.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.chinasofti.bookstore.dao.BookDao"> <resultMap id="book" type="com.chinasofti.bookstore.entity.Book"> <id property="bid" column="BID"/> <result property="bname" column="BNAME"/> <result property="descn" column="DESCN"/> <result property="author" column="author"/> <result property="price" column="PRICE"/> <result property="num" column="num"/> </resultMap> <resultMap id="booktypes" type="com.chinasofti.bookstore.entity.Book" extends="book"> <association property="t" javaType="com.chinasofti.bookstore.entity.Types"> <id property="tid" column="TID"/> <result property="tname" column="TNAME"/> </association> </resultMap> <insert id="insert" parameterType="com.chinasofti.bookstore.entity.Book"> insert into book (bid,bname,author,descn,price,num,tid) values (#{bid},#{bname},#{author},#{descn},#{price},#{num},#{t.tid}); </insert> <delete id="delectById" parameterType="int"> delete from book where bid=#{bid} </delete> <select id="selectByName" parameterType="string" resultMap="booktypes"> select *from (select *from book where bname=#{bname}) b ,types t where b.bid=t.tid </select> <select id="selectAll" resultMap="booktypes"> select*from book b left join types t on bname=#{bname}and b.bid=t.tid </select> <update id="update" parameterType="com.chinasofti.bookstore.entity.Book"> update book <set> <if test="bname!=null">bname=#{bname},</if> <if test="author!=null">author=#{author},</if> <if test="descn!=null">descn=#{descn},</if> <if test="price!=null">price=#{price},</if> <if test="num!=null">num=#{num},</if> </set> where bid=#{bid} </update> </mapper>
bookdao(接口)
package com.chinasofti.bookstore.dao; import com.chinasofti.bookstore.entity.Book; import java.util.List; public interface BookDao { int insert(Book book); int delectById(int bid); int update(Book book); List<Book> selectAll(); Book selectByName(String bname); }
typedao.xml
扫描二维码关注公众号,回复:
9085554 查看本文章
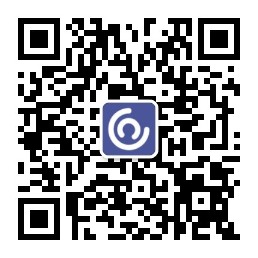
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.chinasofti.bookstore.dao.TypesDao"> <cache/> <sql id="ty">tid,tname</sql> <resultMap id="tp" type="com.chinasofti.bookstore.entity.Types"> <id property="tid" column="TID"/> <result property="tname" column="TNAME"/> </resultMap> <insert id="inserttype" parameterType="string"> insert into types(tname) values (#{tname}); </insert> <delete id="deleteById" parameterType="int"> delete from types where tid=#{tid} </delete> <select id="selectAll" resultMap="tp"> select <include refid="ty"/> from types </select> <select id="selectByName" parameterType="string" resultMap="tp"> select <include refid="ty"/> from types where tname=#{tname} </select> </mapper>
typedao
package com.chinasofti.bookstore.dao; import com.chinasofti.bookstore.entity.Types; import java.util.List; public interface TypesDao { int inserttype(String tname); int deleteById(int tid); List<Types> selectAll(); Types selectByName(String name); }
4,service
bookservice
package com.chinasofti.bookstore.service; import com.chinasofti.bookstore.dao.BookDao; import com.chinasofti.bookstore.entity.Book; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import java.io.IOException; import java.util.List; public class BookService { private SqlSessionFactory factory; private SqlSession session; private BookDao dao; public BookService(){ try { factory = new SqlSessionFactoryBuilder().build(Resources.getResourceAsStream("sqlconfig.xml")); session = factory.openSession(); dao = session.getMapper(BookDao.class); } catch (IOException e) { e.printStackTrace(); System.out.println("sqlsession创建失败"); } } public String addBook(Book book){ Book b = dao.selectByName(book.getBname()); if (b!=null){ return "该书以存在"; } String s= dao.insert(book)>0?"添加成功":"添加失败"; session.commit(); return s; } public List<Book> findAll(){ return dao.selectAll(); } public String remove(int bid){ String s=dao.delectById(bid)>0?"删除成功":"删除失败"; session.commit(); return s; } public String change(Book book){ String s=dao.update(book)>0?"修改成功":"修改失败"; session.commit(); return s; } }
typeservice
package com.chinasofti.bookstore.service; import com.chinasofti.bookstore.dao.TypesDao; import com.chinasofti.bookstore.entity.Types; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import java.io.IOException; import java.util.List; public class TypesService { private SqlSessionFactory factory; private SqlSession session; private TypesDao dao; public TypesService(){ try { factory=new SqlSessionFactoryBuilder().build(Resources.getResourceAsStream("sqlconfig.xml")); session = factory.openSession(); dao = session.getMapper(TypesDao.class); } catch (IOException e) { e.printStackTrace(); System.out.println("创建失败"); } } public String addType(String tname){ Types t = dao.selectByName(tname); if (t!=null){ return "该类别已存在,请重新输入"; } if (dao.inserttype(tname)>0){ session.commit(); return "添加成功"; }else { return "添加失败"; } } public List<Types> findAll(){ return dao.selectAll(); } public String remove(int tid){ String s=dao.deleteById(tid)>0?"删除成功":"删除失败"; session.commit(); return s; } public Types findByName(String tname){ return dao.selectByName(tname); } }
5,util
package com.chinasofti.bookstore.util; import java.util.Scanner; public class UserInput { //创造用于接收用户输入整数的方法 public static int getInt(String wang){ System.out.println(wang); while (true){ Scanner sc=new Scanner(System.in); try { return sc.nextInt(); } catch (Exception e){ System.out.println("对不起,输入格式不正确,请重新输入"); } } } public static double getDouble(String wang){ System.out.println(wang); while (true){ Scanner sc=new Scanner(System.in); try { return sc.nextDouble(); } catch (Exception e){ System.out.println("对不起,输入格式不正确,请重新输入"); } } } public static String getString(String wang){ System.out.println(wang); Scanner sc=new Scanner(System.in); return sc.next(); } }
6,view
package com.chinasofti.bookstore.view; import com.chinasofti.bookstore.entity.Types; import java.util.List; /*输出界面 * 图书管理 1、添加图书类别 2、显示图书类别 3、删除图书类别 4、添加图书 5、查询图书 6、删除图书 7、修改图书 8、根据类别查询图书*/ public class View { public static void welcome(){ System.out.println("---------欢迎来到我的世界--------------"); System.out.println("1、添加图书类别"); System.out.println("2、显示图书类别"); System.out.println("3、删除图书类别"); System.out.println("4、添加图书"); System.out.println("5、查询图书"); System.out.println("6、删除图书"); System.out.println("7、修改图书"); System.out.println("8、根据类别查询图书"); System.out.println("---------------------------------------"); } public static void showType(List<Types> all){ System.out.println("所有类别如下"); System.out.println("编号\t名称"); for (Types t:all){ System.out.println(t.getTid()+"\t"+t.getTname()); } } }
7,control(业务逻辑只写了一个,其余的依葫芦画瓢就好)
package com.chinasofti.bookstore.control; import com.chinasofti.bookstore.entity.Book; import com.chinasofti.bookstore.entity.Types; import com.chinasofti.bookstore.service.BookService; import com.chinasofti.bookstore.service.TypesService; import com.chinasofti.bookstore.util.UserInput; import com.chinasofti.bookstore.view.View; import java.util.List; public class Control { private BookService service; private TypesService typesService; public Control() { this.service = new BookService(); this.typesService=new TypesService(); } public void start(){ //显示主界面 View.welcome(); //接收用户输入的指令 int select = UserInput.getInt("请选择"); if (select<=0){ System.out.println("欢迎下次再来,886"); System.exit(0); }else if(select==4){ this.addbook(); } } private void addbook() { System.out.println("-----------请添加图书--------------"); View.showType(this.typesService.findAll()); System.out.println(this.service.addBook(new Book( UserInput.getString("请输入书名"), UserInput.getString("请输入图书的作者"), UserInput.getString("请输入图书的描述"), UserInput.getInt("请输入图书的价格"), UserInput.getInt("请输入图书的数量"), typesService.findByName(UserInput.getString("请输入类别名称")) ))); } }