Hibernate 的查询语言 HQL 之(二)
数据 库 操作 实现类 类
在 org.sf.dao.impl.DepartmentDaoImpl 实现类中添加如下方法,查询系院信息
public List<Department> getAllDepartmentList(){
List<Department> list = null;
Session session = HibernateSessionFactory.getSession();
Query q=session.createQuery("from Department d");
list = q.list();
session.close();
return list;
}
系 系 院 信息 列表 页面
<%@ page language="java" import="java.util.*" pageEncoding="gbk"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix = "c" %>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title> 系别信息列表</title>
<link rel="stylesheet" type="text/css" href="css/mycss.css">
</head>
<body>
<jsp:useBean id="depDao" class="org.sf.dao.impl.DepartmentDaoImpl"></jsp:useBean>
<c:set var = "depList" value="${depDao.allDepartmentList}" />
<table align="center" border="1">
<tr>
<td colspan="5" align="center">
<a href="depAdd.jsp"> 添加系别信息</a>
<a href="DepServlet"> 查询系别信息</a>
</td>
</tr>
<tr>
<th> 编码</th>
<th> 名称</th>
<th> 电话</th>
<th> 领导</th>
<th> 操作</th>
</tr>
<c:forEach items="${depList}" var ="dep">
<tr>
<td>${dep.bm }</td>
<td>${dep.mc }</td>
<td>${dep.tell }</td>
<td>${dep.leader }</td>
<td>
<a href="depEdit.jsp?id=${dep.id}"> 修改</a>
<a href="depDel.jsp?id=${dep.id}"> 删除</a>
</td>
</tr>
</c:forEach>
<tr>
<td colspan="5" align="center">
<a href="depAdd.jsp"> 添加系别信息</a>
<a href="DepServlet"> 查询系别信息</a>
</td>
</tr>
</table>
</body>
</html>
组合 条件 查询
在实际的应用中, 为了提高程序的方便性, 可以使用组合查询条件进行组合
条件查询, 组合查询的关键点是构建查询条件, 下面以查询系院信息为例做组合查询的应用练习
public List<Department> getDepListByQuery(String bm, String mc, String tell, String
leader)
{
List<Department> list = null;
String hql = "from Department d";
String where="";
if(bm!=null && !bm.equals("")){
where += " where d.bm like '%"+bm+"%'";
}
if(mc!=null && !mc.equals("")){
if(where.equals("")){
where += " where mc like '%"+mc+"%'";
}else{
where += " and mc like '%"+mc+"%'";
}
}
if(tell!=null && !tell.equals("")){
if(where.equals("")){
where += " where tell like '%"+tell+"%'";
}else{
where += " and tell like '%"+tell+"%'";
}
}
if(leader!=null && !leader.equals("")){
if(where.equals("")){
where += " where leader like '%"+leader+"%'";
}else{
where += " and leader like '%"+leader+"%'";
}
}
// 判断查询条件是否为空
if(!where.equals("")){
hql += where;
}
Query q = HibernateSessionFactory.getSession().createQuery(hql);
list = q.list();
return list;
}
创建 Servlet 做 做 请求 处理 与 转向
<servlet>
<servlet-name>DepServlet</servlet-name>
<servlet-class>org.sf.servlet.DepServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>DepServlet</servlet-name>
<url-pattern>/DepServlet</url-pattern>
</servlet-mapping>
修改 DepServlet 的 doGet 方法如下:
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request,response);
}
修改 doPost 方法的内容如下:
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
//request.setCharacterEncoding("gbk");
String bm = request.getParameter("bm");
String mc = request.getParameter("mc");
if(mc!=null && !mc.equals("")){
mc = new String(mc.getBytes("iso-8859-1"), "gbk");
}
String tell = request.getParameter("tell");
if(tell!=null && !tell.equals("")){
tell = new String(tell.getBytes("iso-8859-1"), "gbk");
}
String leader = request.getParameter("leader");
j12Hibernate
6
if(leader!=null && !leader.equals("")){
leader = new String(leader.getBytes("iso-8859-1"), "gbk");
}
IDepartmentDao depDao = new DepartmentDaoImpl();
List<Department> depList = depDao.getDepListByQuery(bm, mc, tell, leader);
HttpSession session = request.getSession();
session.setAttribute("depList", depList);
response.sendRedirect("depquery.jsp");
}
实体 间 的 关系 查询
实体间的关系查询可以使用映射文件配置 many-to-one 或 one-to-many 来解
决,也可以使用 join 级联查询来实现
public List<Student> getAllStudentListByJoin(){
List<Student> list = null;
Session session = HibernateSessionFactory.getSession();
Query q = session.createQuery("select s,d from Student s inner join s.department d");
list = q.list();
session.close();
return list;
}
映射 文件 的 配置
<many-to-one name="department" class="org.sf.entity.Department" fetch="select">
<column name="depid">
<comment> 系别id</comment>
</column>
</many-to-one>
显示信息的页面
<%@ page language="java" import="java.util.*" pageEncoding="gbk"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title> 取得并显示所有的学生</title>
</head>
<body>
<jsp:useBean id="stuDao" class="org.sf.dao.impl.StudentDaoImpl"></jsp:useBean>
<table align="center" border="1">
<tr>
<th> 学号</th>
<th> 姓名</th>
<th> 系院</th>
</tr>
<c:forEach var="stu" items="${stuDao.allStudentListByJoin}">
<tr>
<td>${stu[0].stunumber}</td>
<td>${stu[0].stuname}</td>
<td>${stu[1].mc}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
扫描二维码关注公众号,回复:
9081076 查看本文章
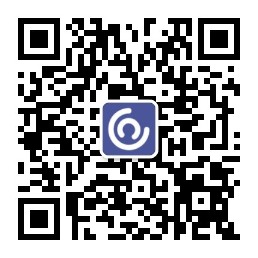
级联查询返回对象为多种类型(这个例子中 select s,d from Student s inner join
s.department d, , 返回 的 类型为 为 Student ,Department 两 两 个 类型 ) ,因些要注意取值的方法,
要取得学生信息使用 stu[0],要取得系院信息使用 stu[1]。