#include<stdio.h>
void float2char(float,char*,int);
int main()
{
char buffer[10];
float2char(123.4567,buffer,10);
printf("%f 转换成字符串 %s\n",123.4567,buffer);
float2char(-654.321,buffer,10);
printf("%f 转换成字符串 %s\n",-654.321,buffer);
return 0;
}
void float2char(float slope,char*buffer,int n) //浮点型数,存储的字符数组,字符数组的长度
{
int temp,i,j;
if(slope>=0)//判断是否大于0
buffer[0] = '+';
else
{
buffer[0] = '-';
slope = -slope;
}
temp = (int)slope;//取整数部分
for(i=0;temp!=0;i++)//计算整数部分的位数
temp /=10;
temp =(int)slope;
for(j=i;j>0;j--)//将整数部分转换成字符串型
{
buffer[j] = temp%10+'0';
temp /=10;
}
buffer[i+1] = '.';
slope -=(int)slope;
for(i=i+2;i<n-1;i++)//将小数部分转换成字符串型
{
slope*=10;
buffer[i]=(int)slope+'0';
slope-=(int)slope;
}
buffer[n-1] = '\0';
}
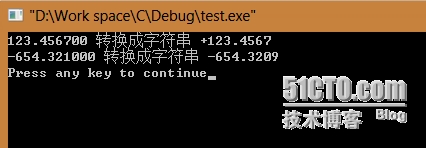
#include<stdio.h>
#include <math.h>
#include <stdlib.h>
const double eps = 1e-11;
void float_to_str(char *str,double num)
{
int high;//float_整数部分
double low;//float_小数部分
char *start=str;
int n=0;
char ch[20];
int i;
high=(int)num;
low=num-high;
while(high>0){
ch[n++]='0'+high%10;
high=high/10;
}
for(i=n-1;i>=0;i--){
*str++=ch[i];
}
num -= (int)num;
double tp = 0.1;
*str++='.';
while(num > eps){//精度限制
num -= tp * (int)(low * 10);
tp /= 10;
*str++='0'+(int)(low*10);
low=low*10.0-(int)(low*10);
}
*str='\0';
str=start;
}
int main()
{
double a;
while( ~scanf("%lf", &a) ) {
char str[20];
float_to_str(str,a);
printf("%s\n\n",str);
}
}
---------------------
作者:MingjaLee
来源:CSDN
原文:https://blog.csdn.net/lijiang1991/article/details/51251634